如何在linux中利用输出输入流在文献中查单词,要求将管理的单词加入到出现频率统计的数据库中,并要输出结果,还要求用到c++链表数据结构struct list_head
时间: 2024-02-22 19:55:16 浏览: 67
在Linux中,可以使用命令行工具grep来查找文献中的单词。例如,如果要查找单词"example"在一个名为"document.txt"的文本文件中出现的次数,可以使用以下命令:
```
grep -o -w 'example' document.txt | wc -l
```
其中,-o选项表示只输出匹配的单词,-w选项表示只匹配完全匹配的单词,wc命令可以统计输出结果的行数,从而得到单词出现的次数。
要将管理的单词加入到出现频率统计的数据库中,可以使用C++中的链表数据结构struct list_head来实现。具体来说,可以定义一个结构体,其中包含一个字符串表示单词,一个整数表示出现的次数,还有一个struct list_head类型的指针表示链表中的下一个元素。然后,在每次查找到一个新的单词时,遍历链表,查找是否已经存在该单词,如果存在则将出现次数加一,否则将该单词添加到链表中。
最后,要输出结果,可以遍历链表,依次输出每个单词以及出现的次数。具体实现可以参考以下代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <list>
using namespace std;
struct WordCount {
string word;
int count;
struct list_head list;
};
int main() {
// 打开文本文件
ifstream file("document.txt");
if(!file.is_open()) {
cerr << "Failed to open file" << endl;
return 1;
}
// 定义链表头
struct list_head head;
INIT_LIST_HEAD(&head);
// 逐行读取文件
string line;
while(getline(file, line)) {
// 查找单词
size_t pos = 0;
while((pos = line.find_first_of("abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ", pos)) != string::npos) {
size_t end = line.find_first_not_of("abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ", pos);
string word = line.substr(pos, end - pos);
// 遍历链表,查找单词是否已经存在
struct WordCount *p;
list_for_each_entry(p, &head, list) {
if(p->word == word) {
p->count++;
goto found;
}
}
// 如果单词不存在,则添加到链表中
struct WordCount *new_word = new struct WordCount;
new_word->word = word;
new_word->count = 1;
list_add_tail(&new_word->list, &head);
found:
pos = end;
}
}
// 输出结果
struct WordCount *p;
list_for_each_entry(p, &head, list) {
cout << p->word << ": " << p->count << endl;
}
return 0;
}
```
阅读全文
相关推荐
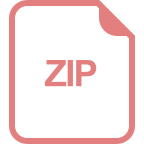
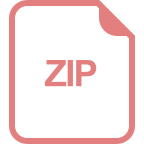
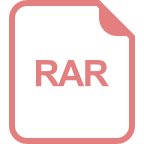
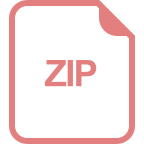
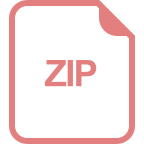
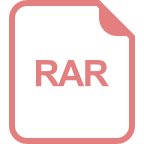
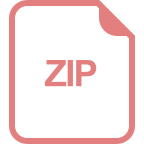
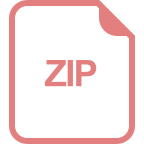
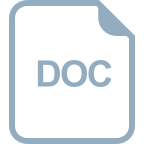
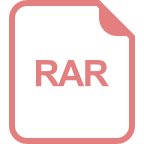
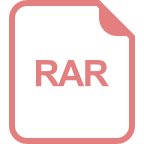
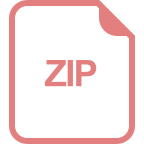
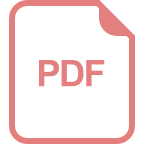
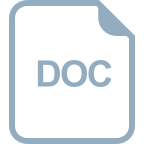
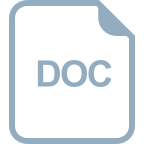