基于ffmpeg进行RTSP推流,推流格式为udp传输 c++
时间: 2024-06-09 09:08:14 浏览: 170
可以使用FFmpeg来进行RTSP推流。下面是一个简单的C++代码示例,演示如何使用FFmpeg进行RTSP推流。
首先需要安装FFmpeg库,可以从官网下载或者使用包管理器进行安装。
代码示例:
```c++
extern "C"
{
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
}
int main(int argc, char* argv[])
{
av_register_all();
AVFormatContext* pFormatCtx = NULL;
AVOutputFormat* fmt = NULL;
AVStream* videoStream = NULL;
AVCodec* videoCodec = NULL;
AVCodecContext* videoCodecCtx = NULL;
AVFrame* videoFrame = NULL;
uint8_t* videoBuffer = NULL;
int videoBufferSize = 0;
int videoFrameCount = 0;
char* outputUrl = "udp://localhost:1234";
avformat_alloc_output_context2(&pFormatCtx, NULL, "udp", outputUrl);
if (!pFormatCtx) {
return -1;
}
fmt = pFormatCtx->oformat;
videoStream = avformat_new_stream(pFormatCtx, NULL);
if (!videoStream) {
return -1;
}
videoCodecCtx = videoStream->codec;
videoCodecCtx->codec_id = fmt->video_codec;
videoCodecCtx->codec_type = AVMEDIA_TYPE_VIDEO;
videoCodecCtx->width = 640;
videoCodecCtx->height = 480;
videoCodecCtx->time_base.num = 1;
videoCodecCtx->time_base.den = 25;
videoCodec = avcodec_find_encoder(videoCodecCtx->codec_id);
if (!videoCodec) {
return -1;
}
if (avcodec_open2(videoCodecCtx, videoCodec, NULL) < 0) {
return -1;
}
videoFrame = av_frame_alloc();
videoBufferSize = avpicture_get_size(videoCodecCtx->pix_fmt, videoCodecCtx->width, videoCodecCtx->height);
videoBuffer = (uint8_t*)av_malloc(videoBufferSize);
avpicture_fill((AVPicture*)videoFrame, videoBuffer, videoCodecCtx->pix_fmt, videoCodecCtx->width, videoCodecCtx->height);
avformat_write_header(pFormatCtx, NULL);
//开始推流
while (videoFrameCount < 1000) {
av_new_packet(&videoPacket, videoBufferSize);
//将YUV数据填充到视频帧中
//...
//将视频帧编码并写入输出流
videoFrame->pts = videoFrameCount++;
int gotPacket = 0;
int ret = avcodec_encode_video2(videoCodecCtx, &videoPacket, videoFrame, &gotPacket);
if (ret < 0) {
return -1;
}
if (gotPacket) {
av_write_frame(pFormatCtx, &videoPacket);
av_free_packet(&videoPacket);
}
}
av_write_trailer(pFormatCtx);
avcodec_close(videoCodecCtx);
av_frame_free(&videoFrame);
av_free(videoBuffer);
avformat_free_context(pFormatCtx);
return 0;
}
```
在这个例子中,我们使用FFmpeg库进行RTSP推流,输出格式为UDP传输。我们首先初始化FFmpeg库,然后创建一个输出上下文。我们使用AVFormatContext结构体来表示输出上下文,fmt结构体来表示输出格式。我们创建一个视频流和一个视频编解码器上下文,设置视频流的属性,如宽度、高度、帧率等。我们查找并打开视频编解码器,并为视频帧分配内存。
在推流前,我们调用avformat_write_header函数,写入文件头。然后我们循环读取YUV数据,将其填充到视频帧中,并将视频帧编码成压缩格式并写入输出流中。我们使用avcodec_encode_video2函数来编码视频帧,并使用AVPacket结构体来表示编码后的视频数据。最后,我们调用av_write_frame函数将视频数据写入输出流中。
当我们完成推流后,我们调用av_write_trailer函数来写入文件尾,并释放内存。
注意:在实际使用时,需要根据具体的需求进行修改和优化。
阅读全文
相关推荐
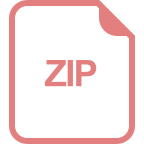
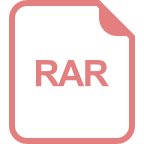
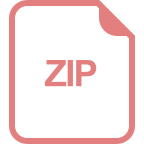















