首页损失函数用这个loss_function=tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=x_train,labels=y_train))
损失函数用这个loss_function=tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=x_train,labels=y_train))
时间: 2023-12-19 18:07:00 浏览: 27
这个损失函数是交叉熵损失函数,常用于多分类问题中。其中,logits是模型的输出,labels是真实标签。tf.nn.softmax_cross_entropy_with_logits()函数会对logits进行softmax计算,然后计算交叉熵损失,最后使用tf.reduce_mean()函数对所有样本的损失求平均值。这个损失函数的作用是衡量模型输出与真实标签之间的差距,目标是使得这个差距最小化。
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
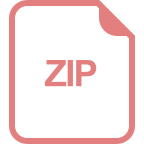
基于三层感知机实现手写数字识别-内含源码和说明书.zip
基于三层感知机实现手写数字识别-内含源码和说明书.zip
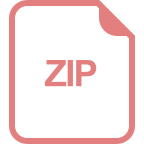
setuptools-40.7.0.zip
Python库是一组预先编写的代码模块,旨在帮助开发者实现特定的编程任务,无需从零开始编写代码。这些库可以包括各种功能,如数学运算、文件操作、数据分析和网络编程等。Python社区提供了大量的第三方库,如NumPy、Pandas和Requests,极大地丰富了Python的应用领域,从数据科学到Web开发。Python库的丰富性是Python成为最受欢迎的编程语言之一的关键原因之一。这些库不仅为初学者提供了快速入门的途径,而且为经验丰富的开发者提供了强大的工具,以高效率、高质量地完成复杂任务。例如,Matplotlib和Seaborn库在数据可视化领域内非常受欢迎,它们提供了广泛的工具和技术,可以创建高度定制化的图表和图形,帮助数据科学家和分析师在数据探索和结果展示中更有效地传达信息。
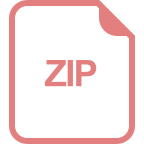
搭建VGG16神经网络实现图像分类-内含源码和说明书.zip
搭建VGG16神经网络实现图像分类-内含源码和说明书.zip
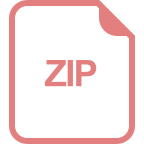
setuptools-40.6.1.zip
Python库是一组预先编写的代码模块,旨在帮助开发者实现特定的编程任务,无需从零开始编写代码。这些库可以包括各种功能,如数学运算、文件操作、数据分析和网络编程等。Python社区提供了大量的第三方库,如NumPy、Pandas和Requests,极大地丰富了Python的应用领域,从数据科学到Web开发。Python库的丰富性是Python成为最受欢迎的编程语言之一的关键原因之一。这些库不仅为初学者提供了快速入门的途径,而且为经验丰富的开发者提供了强大的工具,以高效率、高质量地完成复杂任务。例如,Matplotlib和Seaborn库在数据可视化领域内非常受欢迎,它们提供了广泛的工具和技术,可以创建高度定制化的图表和图形,帮助数据科学家和分析师在数据探索和结果展示中更有效地传达信息。
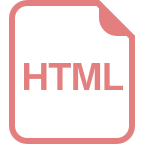
华为OD机试D卷 - 判断字符串子序列 - 免费看解析和代码.html
私信博主免费获取真题解析以及代码
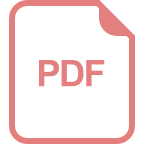
zigbee-cluster-library-specification
最新的zigbee-cluster-library-specification说明文档。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
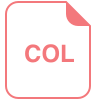
实现实时数据湖架构:Kafka与Hive集成
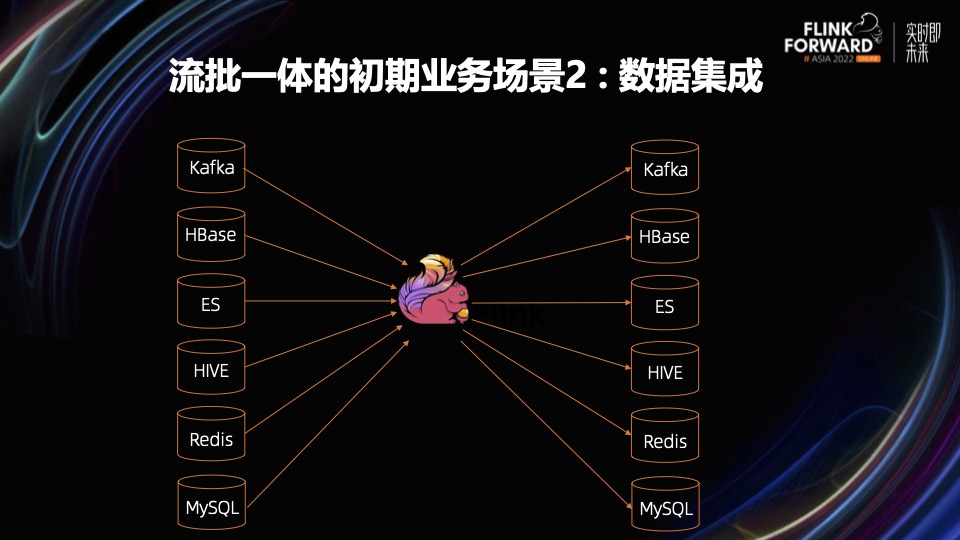
# 1. 实时数据湖架构概述**
实时数据湖是一种现代数据管理架构,它允许企业以低延迟的方式收集、存储和处理大量数据。与传统数据仓库不同,实时数据湖不依赖于预先定义的模式,而是采用灵活的架构,可以处理各种数据类型和格式。这种架构为企业提供了以下优势:
- **实时洞察:**实时数据湖允许企业访问最新的数据,从而做出更明智的决策。
- **数据民主化:**实时数据湖使各种利益相关者都可

用 Python 画一个可以动的爱心
好的,我可以帮助您使用Python来绘制一个动态的爱心。您可以使用turtle库来实现。以下是实现代码:
```python
import turtle
import math
# 设置画布和画笔
canvas = turtle.Screen()
canvas.bgcolor("black")
pencil = turtle.Turtle()
pencil.speed(0)
pencil.color("red", "pink")
pencil.pensize(3)
# 定义爱心函数
def draw_love(heart_size, x_offset=0, y_offset=0):
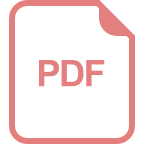
JSBSim Reference Manual
JSBSim参考手册,其中包含JSBSim简介,JSBSim配置文件xml的编写语法,编程手册以及一些应用实例等。其中有部分内容还没有写完,估计有生之年很难看到完整版了,但是内容还是很有参考价值的。