编程实现 a) 调用 pipe()创建无名管道 b) 调用 fork 创建一个子进程 c) 子进程 - 用 signal( )安装信号 SIGALRM 在 SIGALRM 的信号处理函数内执行下列操作: 打印出 pid 和接收到的信号值 向管道内写入所有的小写字母,打印出进程号及成功写入的字节数 - 用 signal( )安装信号 SIGINT 在 SIGINT 的信号处理函数内执行下列操作: 打印出 pid 和接收到的信号值 重新安装信号 SIGINT,采用默认方式处理该信号 - 设置一个定时器,2s 后产生 SIGALRM 信号 - 进入 while(1)循环,每隔 1s 打印出一行” ...child is waiting” d) 父进程 - 打印出进程号 - 从管道读出数据,每次读 8 个字符,直到读出管道中的所有内容 打印出每次读出的字节数及读出的内容 - 向子进程发送信号 SIGINT - 等待 1s - 再次向子进程发送信号 SIGINT - 等待子进程结束 - 父进程退出
时间: 2024-03-03 16:48:27 浏览: 101
下面是 C 语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
#include <sys/wait.h>
#define BUFFER_SIZE 26
void sig_alrm(int signo);
void sig_int(int signo);
int main() {
int fd[2]; // 管道
pid_t pid;
char buf[BUFFER_SIZE];
int n;
if (pipe(fd) < 0) { // 创建管道
perror("pipe error");
exit(1);
}
if ((pid = fork()) < 0) { // 创建子进程
perror("fork error");
exit(1);
} else if (pid == 0) { // 子进程
signal(SIGALRM, sig_alrm);
signal(SIGINT, sig_int);
alarm(2); // 设置定时器
while (1) {
printf("...child is waiting\n");
sleep(1);
}
} else { // 父进程
printf("parent pid=%d\n", getpid());
close(fd[1]); // 关闭写端
while ((n = read(fd[0], buf, BUFFER_SIZE)) > 0) { // 从管道读出数据
printf("read %d bytes: %s\n", n, buf);
}
if (n < 0) {
perror("read error");
exit(1);
}
kill(pid, SIGINT); // 向子进程发送 SIGINT 信号
sleep(1);
kill(pid, SIGINT); // 再次向子进程发送 SIGINT 信号
waitpid(pid, NULL, 0); // 等待子进程结束
exit(0);
}
}
void sig_alrm(int signo) {
printf("child pid=%d received SIGALRM signal %d\n", getpid(), signo);
char buf[BUFFER_SIZE];
int n = 0;
char c = 'a';
while (c <= 'z') {
buf[n++] = c++;
}
if (write(STDOUT_FILENO, buf, n) != n) {
perror("write error");
exit(1);
}
printf("child pid=%d wrote %d bytes to pipe\n", getpid(), n);
alarm(2); // 重新设置定时器
}
void sig_int(int signo) {
printf("child pid=%d received SIGINT signal %d\n", getpid(), signo);
signal(SIGINT, SIG_DFL); // 重新安装信号 SIGINT,采用默认方式处理该信号
}
```
阅读全文
相关推荐
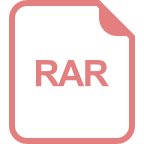
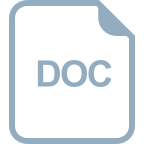



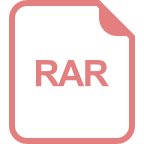
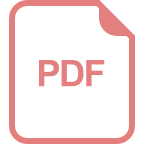
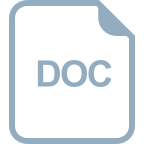
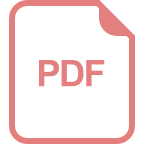
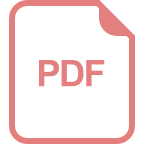
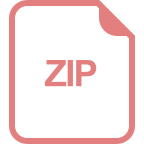
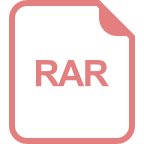
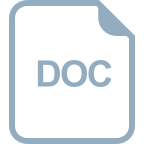
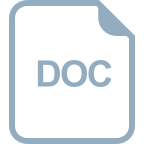
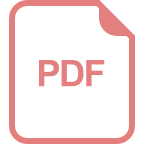
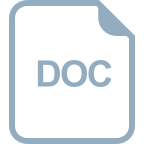
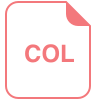
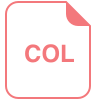