YOLO格式数据集转换成VOC数据集的python代码
时间: 2023-11-13 22:03:45 浏览: 52
以下是将YOLO格式数据集转换为VOC格式数据集的Python代码示例:
```python
import os
import xml.etree.ElementTree as ET
# Define the classes present in your dataset
classes = ["class1", "class2", "class3"]
def convert_yolo_to_voc(yolo_path, voc_path):
"""
Convert YOLO format dataset to VOC format dataset
:param yolo_path: path to the YOLO dataset directory
:param voc_path: path to the VOC dataset directory
"""
# Create the VOC dataset directory if it doesn't exist
if not os.path.exists(voc_path):
os.makedirs(voc_path)
# Loop through all the files in the YOLO dataset directory
for file_name in os.listdir(yolo_path):
if file_name.endswith(".txt"):
# Open the YOLO annotation file
with open(os.path.join(yolo_path, file_name), "r") as f:
lines = f.readlines()
# Extract the image dimensions from the corresponding image file
img_path = os.path.join(yolo_path, file_name.replace(".txt", ".jpg"))
img = Image.open(img_path)
img_width, img_height = img.size
# Create a new VOC annotation file with the same name as the YOLO annotation file
xml_file_name = file_name.replace(".txt", ".xml")
xml_file_path = os.path.join(voc_path, xml_file_name)
root = ET.Element("annotation")
ET.SubElement(root, "filename").text = file_name.replace(".txt", ".jpg")
size = ET.SubElement(root, "size")
ET.SubElement(size, "width").text = str(img_width)
ET.SubElement(size, "height").text = str(img_height)
# Loop through each line in the YOLO annotation file
for line in lines:
class_id, x_center, y_center, box_width, box_height = map(float, line.split())
# Convert the YOLO format coordinates to VOC format coordinates
x_min = int((x_center - box_width/2) * img_width)
y_min = int((y_center - box_height/2) * img_height)
x_max = int((x_center + box_width/2) * img_width)
y_max = int((y_center + box_height/2) * img_height)
# Create a new object element in the VOC annotation file
object_tag = ET.SubElement(root, "object")
ET.SubElement(object_tag, "name").text = classes[int(class_id)]
bndbox = ET.SubElement(object_tag, "bndbox")
ET.SubElement(bndbox, "xmin").text = str(x_min)
ET.SubElement(bndbox, "ymin").text = str(y_min)
ET.SubElement(bndbox, "xmax").text = str(x_max)
ET.SubElement(bndbox, "ymax").text = str(y_max)
# Write the VOC annotation file to disk
tree = ET.ElementTree(root)
tree.write(xml_file_path)
# Call the function with the YOLO and VOC dataset directory paths
yolo_path = "/path/to/yolo/dataset"
voc_path = "/path/to/voc/dataset"
convert_yolo_to_voc(yolo_path, voc_path)
```
这段代码将YOLO格式数据集目录中的所有文本文件转换为VOC格式的XML文件,并将它们保存到指定的目录中。每个XML文件对应于一个图像文件,其中包含图像的名称、尺寸和每个对象的边界框。如果您需要更改类别名称,请修改代码中的classes列表。
相关推荐
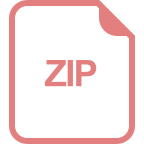
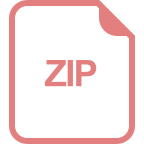














