U8 (void(*function)(void), U32 time)
时间: 2023-11-16 08:04:17 浏览: 36
This is a function declaration in C programming language.
The function is named "U8" and it takes two arguments:
1. A pointer to a function that takes no arguments and returns nothing (void(*function)(void))
2. An unsigned 32-bit integer variable named "time" (U32 time)
The purpose of this function is not clear without additional context, but it appears to be used for scheduling tasks to run at specific times.
相关问题
修改下述程序,实现用定时器0定时,使得LED灯每1ms闪烁一次:// // INT0---P3.2 ---connect to a button // when the button is pressed, the LED changes between fast blink and slow blink // ------CH 2021.7.29 //************************************************************* #include "STC32G.h" #include "stdio.h" #include "intrins.h" typedef unsigned char u8; typedef unsigned int u16; typedef unsigned long u32; #define MAIN_Fosc 24000000UL //========================================================================== // sbit INT1 = P3^3; bit flag=0; // /************* function declaration **************/ void delay_ms(u8 ms); /******************** Main function**************************/ void main(void) { u8 i; WTST = 0; P0M1 = 0x00; P0M0 = 0x00; //IO set up P1M1 = 0x00; P1M0 = 0x00; P2M1 = 0x00; P2M0 = 0x00; P3M1 = 0x0f; P3M0 = 0x00; P4M1 = 0xff; P4M0 = 0x00; P5M1 = 0x00; P5M0 = 0x00; P6M1 = 0x00; P6M0 = 0x00; P7M1 = 0x00; P7M0 = 0x00; EX0 = 1; //interrupt enable EA = 1; //all interrupt enable IT0=1; // edge trigger while(1) { if(flag==0) { i=1000; } else {i=10; } P2=0xff; delay_ms(i); P2=0x00; delay_ms(i); } } //======================================================================== void delay_ms(u8 ms) { u16 i; do{ i = MAIN_Fosc / 6000; while(--i); }while(--ms); } /********************* INT0*************************/ void INT0_int (void) interrupt 0 { flag=~flag; }
下面是修改后的程序,使用定时器0实现LED每1ms闪烁一次:
```c
#include "STC32G.h"
#include "stdio.h"
#include "intrins.h"
typedef unsigned char u8;
typedef unsigned int u16;
typedef unsigned long u32;
#define MAIN_Fosc 24000000UL
bit flag = 0;
void delay_ms(u8 ms);
void main(void)
{
u8 i;
WTST = 0;
P0M1 = 0x00;
P0M0 = 0x00;
P1M1 = 0x00;
P1M0 = 0x00;
P2M1 = 0x00;
P2M0 = 0x00;
P3M1 = 0x0f;
P3M0 = 0x00;
P4M1 = 0xff;
P4M0 = 0x00;
P5M1 = 0x00;
P5M0 = 0x00;
P6M1 = 0x00;
P6M0 = 0x00;
P7M1 = 0x00;
P7M0 = 0x00;
EX0 = 1;
EA = 1;
IT0 = 1;
TMOD |= 0x01; // 设置定时器计数模式为模式1
TH0 = (65536 - (MAIN_Fosc / 1000)) / 256; // 设置定时器初值
TL0 = (65536 - (MAIN_Fosc / 1000)) % 256;
TR0 = 1; // 启动定时器
while (1)
{
if (flag == 0)
{
i = 1000;
}
else
{
i = 10;
}
P2 = 0xff;
delay_ms(i);
P2 = 0x00;
delay_ms(i);
}
}
void delay_ms(u8 ms)
{
u16 i;
do
{
i = MAIN_Fosc / 6000;
while (--i)
;
} while (--ms);
}
void INT0_int(void) interrupt 0
{
flag = ~flag;
}
```
这个修改后的程序使用了定时器0来实现延时,每1ms触发一次中断,控制LED的闪烁。请确保定时器计数频率正确设置,并且定时器的中断向量正确配置为中断0。
u8api 自定义按键
### 回答1:
u8api 自定义按键是一项非常实用的功能,它允许您在使用u8g2库的过程中,根据自己的喜好和需要,自定义按键的功能和触发方式。
通过u8api自定义按键,您可以实现各种按键功能,例如菜单切换、状态改变、数值调整、动作执行等等。同时,您还可以自定义按键触发方式,例如短按、长按、双击、滑动等等。这些操作可以通过编写自定义函数来实现。
要使用u8api自定义按键功能,首先需要在代码中定义按键的GPIO引脚、触发方式、处理函数等。然后,在u8g2库中添加对应的按键处理函数和触发方式。
例如,在u8g2库中添加一个双击触发的按键处理函数,可以使用以下代码:
```
void u8g2_AddButton_DoubleClick(u8g2_t *u8g2, uint8_t pin) {
if (u8g2_GetPinState(u8g2, pin) == 0) {
if (u8g2_GetTickCount(u8g2) - u8g2->gpio.button_last_tick[pin] < u8g2ButtonDoubleClickDelay) {
u8g2->gpio.button_last_tick[pin] = 0xFFFFFFFE;
if (u8g2->gpio.button_fn[pin]) {
u8g2->gpio.button_fn[pin](u8g2, pin, U8G2_BTN_DOUBLECLICK);
}
}
else {
u8g2->gpio.button_last_tick[pin] = u8g2_GetTickCount(u8g2);
}
}
}
```
然后,在代码中调用该函数,即可实现双击触发的按键操作:
```
u8g2_AddButton_DoubleClick(&u8g2, U8X8_PIN_A0);
```
总之,u8api自定义按键功能可以帮助您更好地控制设备和交互界面,提高用户体验和功能性,是非常值得尝试的一项功能。
### 回答2:
u8api是一种开源的物联网开发框架,可以用于快速搭建智能家居、智能办公等物联网应用。其中,u8api提供了自定义按键的功能,可以通过设置按键的行为,实现对智能设备的控制。
使用u8api自定义按键,需要先定义按键的GPIO口和按键事件。 GPIO口即GPIO引脚,按键事件包括按下、松开、长按等。在u8api中,可以通过以下代码定义按键:
u8_gpio_setfunction(u8_gpio_pin_t gpio_pin, u8_gpio_function_t function);
u8_gpio_setinttype(u8_gpio_pin_t gpio_pin, u8_gpio_inttype_t inttype, u8_gpio_intpolarity_t intpolarity);
u8_gpio_setintcallback(u8_gpio_pin_t gpio_pin, u8_gpio_intcallback_t intcallback);
其中,u8_gpio_setfunction用于设置GPIO口的功能模式,如输入、输出等;u8_gpio_setinttype用于设置GPIO口的中断类型,如下降沿、上升沿等;u8_gpio_setintcallback用于设置GPIO口的中断回调函数,即响应按键事件的函数。
在设置好按键的GPIO口和按键事件后,就可以用编程语言(如Lua、Python等)编写控制智能设备的脚本了。例如,可以通过检测按键的状态(按下或松开),控制智能灯的开关、亮度等属性。
总的来说,u8api的自定义按键功能可以方便地实现智能家居、智能办公等物联网应用中的按键控制。通过编写简单的脚本,可以实现对智能设备的多种操作,提高了智能化生活的便捷性。
### 回答3:
u8api 自定义按键,是针对嵌入式设备设计的应用程序编程接口(API)。通常,这个接口提供了一种方式,允许开发者在嵌入式系统上自定义按键,并对按键触发事件进行处理。
开发人员通常会首先配置u8api 自定义按键 API,并将其绑定到特定的物理按键上。因此,即使嵌入式设备上没有预设的按键,应用程序也能够支持按键输入。
一旦按键被绑定,用户便可以执行各种操作。例如,可以在按键的触发事件上运行特定代码,例如打开调试模式、暂停或恢复应用程序、调整音量等等。
小型设备和嵌入式系统中的自定义按键功能对于用户体验和功能实现都非常重要。由于它们可以提高设备的易用性和可扩展性,使得用户可以更轻松地完成任务并快速地适应新的环境。对于嵌入式系统的应用开发者,u8api 自定义按键 API 可以帮助开发者轻松地添加必要的功能,以满足他们的客户需求,从而提高设备的可用性。
相关推荐
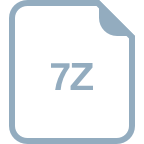
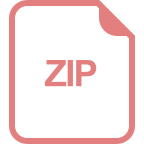
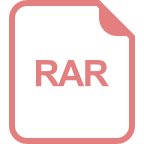













