x = x.permute(0, 2, 1) # change back to shape [batch_size, seq_len, input_dim]是什么意思
时间: 2023-12-12 07:05:50 浏览: 217
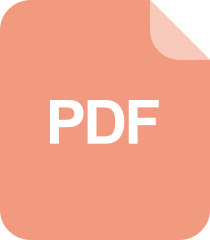
pytorch permute维度转换方法
`x.permute(0, 2, 1)` 表示将 `x` 的维度进行变换,使得原先的第二维变成了第三维,第三维变成了第二维,即将形状为 `[batch_size, input_dim, seq_len]` 的 `x` 变换为形状为 `[batch_size, seq_len, input_dim]` 的 `x`。这是因为在多头注意力机制层中,输入和输出张量的形状必须满足一定的条件,其中第二维表示序列长度(也就是输入的词数或者图像的像素数),第三维表示特征维度。在这个模型中,输入经过多头注意力机制层后的输出形状为 `[seq_len, batch_size, input_dim]`,因此最后需要将第二维和第三维交换,以使输出的形状为 `[batch_size, seq_len, input_dim]`。这样,输出就能够方便地输入到后续的线性层中进行处理。
阅读全文
相关推荐
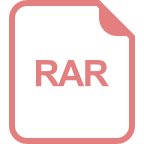
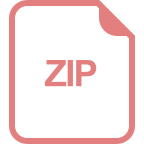
















