基于cordic算法的nco实现
时间: 2023-10-09 10:04:23 浏览: 78
CORDIC算法(Coordinate Rotation Digital Computer)是一种用于计算三角函数和旋转变换的算法。NCO(Numerically Controlled Oscillator)是一种数字控制的振荡器,可用于产生各种信号波形,如正弦波、方波等。
基于CORDIC算法实现的NCO主要分为以下几步:
1. 初始化:设置初始角度、幅度、步长等参数。
2. 计算:根据CORDIC算法的迭代公式,通过不断旋转坐标系来计算所需的三角函数值。
3. 输出:根据计算结果,生成相应的信号波形输出。
下面是一个基于CORDIC算法的NCO实现的示例代码(使用Verilog语言):
```
module cordic_nco (
input clk, // 时钟信号
input rst, // 复位信号
input signed [31:0] freq, // 频率参数
output signed [31:0] sine // 正弦波输出
);
// 定义常量
localparam integer ANGLE_WIDTH = 32; // 角度宽度
localparam integer FREQ_WIDTH = 32; // 频率宽度
localparam integer CORDIC_DEPTH = 16; // CORDIC算法迭代深度
localparam integer SCALE = 1 << (CORDIC_DEPTH - 1); // 缩放因子
// 定义变量
reg signed [ANGLE_WIDTH-1:0] angle; // 角度
reg signed [ANGLE_WIDTH-1:0] phase; // 相位
reg signed [FREQ_WIDTH-1:0] phase_inc; // 相位增量
reg signed [CORDIC_DEPTH-1:0] cordic_vector [0:CORDIC_DEPTH-1]; // CORDIC向量表
reg signed [31:0] sine_out; // 正弦波输出
// 初始化
initial begin
angle = 0;
phase = 0;
phase_inc = freq * SCALE / (2 * $bits(sine_out));
cordic_vector[0] = SCALE;
for (int i = 1; i < CORDIC_DEPTH; i = i+1) begin
cordic_vector[i] = cordic_vector[i-1] >> 1;
end
end
// 迭代计算
always @(posedge clk or posedge rst) begin
if (rst) begin
angle <= 0;
phase <= 0;
sine_out <= 0;
end else begin
angle <= angle + phase_inc;
phase <= angle;
// CORDIC算法迭代
for (int i = 0; i < CORDIC_DEPTH; i = i+1) begin
if (phase >= 0) begin
sine_out = sine_out + cordic_vector[i];
phase = phase - arctan(2**(-i));
end else begin
sine_out = sine_out - cordic_vector[i];
phase = phase + arctan(2**(-i));
end
end
end
end
// 输出正弦波
assign sine = sine_out;
// 计算反正切函数值
function signed [ANGLE_WIDTH-1:0] arctan;
input signed [ANGLE_WIDTH-1:0] x;
begin
if (x >= 0) begin
arctan = {ANGLE_WIDTH{1'b0}};
end else begin
arctan = {ANGLE_WIDTH{1'b1}};
end
for (int i = 0; i < ANGLE_WIDTH; i = i+1) begin
if (x >= 0) begin
x = x - (1 << (-i));
arctan[i] = 1;
end else begin
x = x + (1 << (-i));
arctan[i] = -1;
end
end
end
endfunction
endmodule
```
在这个例子中,我们使用了一个16位的CORDIC向量表来进行迭代计算。在每个时钟周期中,我们根据频率参数和缩放因子计算出相应的相位增量,并将角度加上相位增量。然后,我们使用CORDIC算法迭代计算正弦波输出。最后,我们将正弦波输出赋值给输出端口。
相关推荐
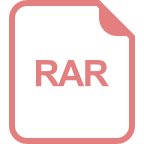
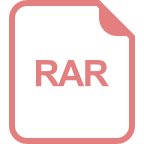














