使用libjpeg库将yuv420sp压缩为jpeg C++代码
时间: 2023-09-30 12:06:10 浏览: 113
以下是使用libjpeg库将YUV420sp图像压缩为JPEG的C++代码示例:
```cpp
#include <stdio.h>
#include <jpeglib.h>
// YUV420sp图像数据
// yData:Y分量数据
// uvData:U和V分量交替存储的数据
// width:图像宽度
// height:图像高度
void compressYUV420spToJPEG(unsigned char* yData, unsigned char* uvData, int width, int height, const char* filename) {
struct jpeg_compress_struct cinfo;
struct jpeg_error_mgr jerr;
// 创建JPEG压缩对象并设置错误处理
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_compress(&cinfo);
// 打开输出文件
FILE* outfile;
if ((outfile = fopen(filename, "wb")) == NULL) {
fprintf(stderr, "Can't open output file.\n");
return;
}
jpeg_stdio_dest(&cinfo, outfile);
// 设置图像参数
cinfo.image_width = width;
cinfo.image_height = height;
cinfo.input_components = 3;
cinfo.in_color_space = JCS_YCbCr;
jpeg_set_defaults(&cinfo);
// 设置压缩参数
jpeg_set_quality(&cinfo, 90, TRUE);
jpeg_set_colorspace(&cinfo, JCS_YCbCr);
jpeg_default_colorspace(&cinfo);
// 开始压缩
jpeg_start_compress(&cinfo, TRUE);
// 每次处理一行数据
JSAMPROW row[1];
int row_stride = width * 3; // 一行数据的字节数
row[0] = new JSAMPLE[row_stride];
while (cinfo.next_scanline < cinfo.image_height) {
// 将Y分量数据复制到输出行
for (int i = 0; i < width; i++) {
row[0][i * 3] = yData[cinfo.next_scanline * width + i];
}
// 将UV分量数据复制到输出行
for (int i = 0; i < width; i += 2) {
row[0][i * 3 + 1] = uvData[(cinfo.next_scanline / 2) * width + i];
row[0][i * 3 + 2] = uvData[(cinfo.next_scanline / 2) * width + i + 1];
}
// 压缩当前行数据
jpeg_write_scanlines(&cinfo, row, 1);
}
// 完成压缩
jpeg_finish_compress(&cinfo);
// 释放资源
jpeg_destroy_compress(&cinfo);
fclose(outfile);
delete[] row[0];
}
int main() {
// 假设已有YUV420sp图像数据,存储在yData和uvData中
unsigned char* yData;
unsigned char* uvData;
int width;
int height;
// 调用函数进行压缩
compressYUV420spToJPEG(yData, uvData, width, height, "output.jpg");
return 0;
}
```
请注意,此代码中假设已经拥有YUV420sp图像数据,存储在yData和uvData中。你需要替换为实际的图像数据。此外,你需要安装libjpeg库并在编译时链接该库。具体的编译命令可以根据你的开发环境进行调整。
阅读全文
相关推荐
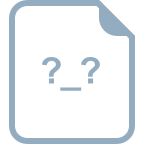

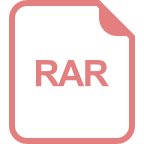

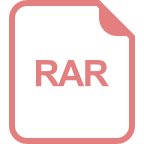
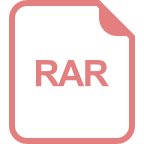
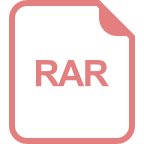
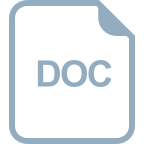
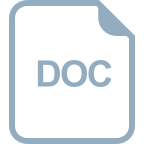
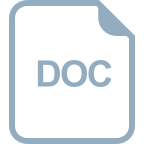






