实现编辑全部在使用deleteItem删除全部时显示新增addItem按钮事件<template> <div> <a-table :pagination="false" :columns="columns" :dataSource="dataSource"> <!-- //循环展示数据或input输入框 --> <template v-for="col in ['abbreviation', 'fullName', 'nodes']" :slot="col" slot-scope="text, record, index" > <div :key="col"> <a-input v-if="editableData[record.key]" v-model="editableData[record.key][col]" /> <template v-else>{{ text }}</template> </div> </template> <!-- //操作 --> <template slot="operation" slot-scope="text, record, index"> <span v-if="editableData[record.key]"> <a-icon type="check" @click="save(record.key)" /> </span> <span v-else> <a-icon type="delete" @click="deleteItem(record.key)" /> <a-icon type="edit" @click="edit(record.key)" /> <a-icon type="plus" v-if="index==dataSource.length-1" @click="addItem(record.key)" /> </span> </template> </a-table> </div> </template> <script> import { cloneDeep } from "lodash"; export default { data() { return { editableData: [], //正在编辑的数组 columns: [ { title: "简称", dataIndex: "abbreviation", scopedSlots: { customRender: "abbreviation" } }, { title: "全称", dataIndex: "fullName", scopedSlots: { customRender: "fullName" } }, { title: "来源", dataIndex: "nodes", scopedSlots: { customRender: "nodes" } }, { title: "操作", dataIndex: "operation", scopedSlots: { customRender: "operation" } } ], //表格数据 dataSource: [ { key: 0, abbreviation: "简称1", fullName: "全称1", nodes: "来源1" }, { key: 1, abbreviation: "简称2", fullName: "全称2", nodes: "来源2" }, { key: 2, abbreviation: "简称3", fullName: "全称3", nodes: "来源3" }, { key: 3, abbreviation: "简称14", fullName: "全称14", nodes: "来源14" } ] }; }, components: {}, props: ["tableDatas"], watch: {}, updated() {}, created() {}, methods: { addItem(key) { let item = { key: key + 1, abbreviation: "", fullName: "", nodes: "" }; this.dataSource.splice(key + 1, 0, item); this.$set(this.editableData, key + 1, item); }, deleteItem(key) { this.dataSource = this.dataSource.filter(item => item.key !== key); }, edit(key) { let editItem = cloneDeep( this.dataSource.filter(item => key === item.key)[0] ); this.$set(this.editableData, key, editItem); }, save(key) { Object.assign( this.dataSource.filter(item => key === item.key)[0], this.editableData[key] ); this.$set(this.editableData, key, null); } } }; </script>
时间: 2023-07-06 22:38:57 浏览: 224
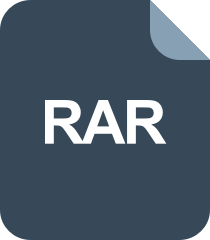
Flex使用<mx:Tree>控件创建树(可添加和删除节点)
根据您提供的代码,我理解您的需求是在删除所有数据项时,在页面上显示一个新增按钮。为了实现这个功能,您可以在删除全部数据项时,手动向数据源中添加一个空数据项,并将其 key 属性值设置为当前数据源长度。另外,您需要在渲染数据项时,判断当前数据项是否为空数据项,以便在页面上显示新增按钮。
下面是修改后的代码示例:
```
<template>
<div>
<a-table :pagination="false" :columns="columns" :dataSource="dataSource">
<!-- //循环展示数据或input输入框 -->
<template v-for="col in ['abbreviation', 'fullName', 'nodes']" :slot="col" slot-scope="text, record, index">
<div :key="col">
<a-input v-if="editableData[record.key]" v-model="editableData[record.key][col]" />
<template v-else>
{{ record.key === 'new' ? '' : text }}
</template>
</div>
</template>
<!-- //操作 -->
<template slot="operation" slot-scope="text, record, index">
<span v-if="editableData[record.key]">
<a-icon type="check" @click="save(record.key)" />
</span>
<span v-else>
<a-icon type="delete" @click="deleteItem(record.key)" />
<a-icon type="edit" @click="edit(record.key)" />
<a-icon type="plus" v-if="record.key === 'new'" @click="addItem()" />
</span>
</template>
</a-table>
</div>
</template>
<script>
import { cloneDeep } from "lodash";
export default {
data() {
return {
editableData: [], //正在编辑的数组
columns: [
{
title: "简称",
dataIndex: "abbreviation",
scopedSlots: { customRender: "abbreviation" }
},
{
title: "全称",
dataIndex: "fullName",
scopedSlots: { customRender: "fullName" }
},
{
title: "来源",
dataIndex: "nodes",
scopedSlots: { customRender: "nodes" }
},
{
title: "操作",
dataIndex: "operation",
scopedSlots: { customRender: "operation" }
}
],
//表格数据
dataSource: [
{ key: 0, abbreviation: "简称1", fullName: "全称1", nodes: "来源1" },
{ key: 1, abbreviation: "简称2", fullName: "全称2", nodes: "来源2" },
{ key: 2, abbreviation: "简称3", fullName: "全称3", nodes: "来源3" },
{ key: "new", abbreviation: "", fullName: "", nodes: "" }
]
};
},
components: {},
props: ["tableDatas"],
watch: {},
updated() {},
created() {},
methods: {
addItem() {
let item = { key: this.dataSource.length, abbreviation: "", fullName: "", nodes: "" };
this.dataSource.splice(this.dataSource.length - 1, 0, item);
this.$set(this.editableData, item.key, item);
},
deleteItem(key) {
this.dataSource = this.dataSource.filter(item => item.key !== key);
},
edit(key) {
let editItem = cloneDeep(this.dataSource.filter(item => key === item.key)[0]);
this.$set(this.editableData, key, editItem);
},
save(key) {
Object.assign(this.dataSource.filter(item => key === item.key)[0], this.editableData[key]);
this.$set(this.editableData, key, null);
}
}
};
</script>
```
希望这个答案能够帮助到您。
阅读全文
相关推荐
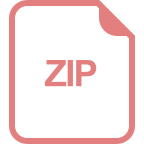
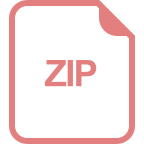
















