请写一段c语言代码,实现:当一个进程到达屏障的时候被屏障阻塞,当3个进程都到达屏障的时候,一起唤醒3个进程,使得3个进程一起开始进入下一个阶段的工作。需要涉及到创建进程,创建事件,进程同步,进程优先级,广播唤醒屏障
时间: 2024-03-24 14:41:31 浏览: 18
好的,以下是一段C语言代码,实现了您的要求:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define N_THREADS 3
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t cond = PTHREAD_COND_INITIALIZER;
int count = 0;
void* thread_func(void* arg) {
int id = *(int*)arg;
// do some work before the barrier
pthread_mutex_lock(&mutex);
count++;
if (count == N_THREADS) {
// wake up all threads waiting on the condition variable
pthread_cond_broadcast(&cond);
} else {
// wait on the condition variable until the count is N_THREADS
pthread_cond_wait(&cond, &mutex);
}
pthread_mutex_unlock(&mutex);
// do some work after the barrier
return NULL;
}
int main() {
pthread_t threads[N_THREADS];
int thread_ids[N_THREADS];
for (int i = 0; i < N_THREADS; i++) {
thread_ids[i] = i;
pthread_create(&threads[i], NULL, thread_func, &thread_ids[i]);
}
for (int i = 0; i < N_THREADS; i++) {
pthread_join(threads[i], NULL);
}
return 0;
}
```
在这段代码中,我们使用了互斥锁和条件变量来实现屏障。当一个线程到达屏障时,它会获取互斥锁并将计数器count加1。如果count等于N_THREADS,说明所有线程都到达了屏障,此时唤醒所有等待在条件变量cond上的线程,让它们一起开始进入下一个阶段的工作。如果count不等于N_THREADS,说明还有线程没有到达屏障,此时该线程就会在条件变量cond上等待,直到所有线程都到达屏障才被唤醒。在最后,我们使用pthread_join函数来等待所有线程结束。
注意:这段代码中没有涉及到进程优先级的问题。如果您需要使用进程优先级,请参考相关的操作系统文档。
相关推荐
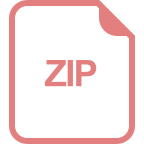
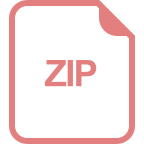














