c++建立图的邻接矩阵
时间: 2023-10-20 18:28:48 浏览: 33
在C++中,我们可以使用二维数组来表示图的邻接矩阵。其中,每个元素表示两个顶点之间是否有边相连,如果相连则为1,否则为0。
以下是一个简单的示例代码:
```c++
const int MAXN = 100; // 最大顶点数
int G[MAXN][MAXN]; // 邻接矩阵
int main() {
int n, m; // n为顶点数,m为边数
cin >> n >> m;
for (int i = 1; i <= m; i++) {
int u, v; // u和v分别为一条边的两个端点
cin >> u >> v;
G[u][v] = G[v][u] = 1; // 无向图需要将两个方向都设置为1
}
return 0;
}
```
其中,输入的第一行为顶点数n和边数m,接下来m行为每条边的两个端点u和v。在处理边的时候,需要将G[u][v]和G[v][u]都设置为1,因为无向图中的一条边对应着两个方向。
相关问题
c++输出地图邻接矩阵
假设地图中有n个点,我们可以使用一个n*n的矩阵来表示它们之间的连接关系。如果两个点之间有连接,则在矩阵中对应位置上的值为1,否则为0。下面是一个简单的C++程序,用于输出地图的邻接矩阵:
```c++
#include <iostream>
using namespace std;
const int MAXN = 100;
int map[MAXN][MAXN]; // 定义地图的邻接矩阵
int main()
{
int n; // 地图中的点数
cin >> n;
for (int i = 0; i < n; i++)
for (int j = 0; j < n; j++)
cin >> map[i][j]; // 输入地图的邻接矩阵
// 输出地图的邻接矩阵
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++)
cout << map[i][j] << " ";
cout << endl;
}
return 0;
}
```
在这个程序中,我们通过一个二维数组`map`来存储地图的邻接矩阵,然后通过两个嵌套的循环遍历这个数组,输出每一个元素的值。注意,在输入地图的邻接矩阵时,我们也是通过两个嵌套的循环来完成的。
c++dfs算法 邻接矩阵
C++中的DFS算法是一种图遍历算法,它可以用于解决很多问题,如图的连通性、最短路径、最小生成树等。邻接矩阵是一种表示图的数据结构,它可以用于存储图的信息,包括节点之间的连接关系和权值等。在使用DFS算法时,我们可以通过邻接矩阵来表示图,并通过深度遍历来查找图中的节点。
具体来说,使用邻接矩阵表示图时,我们可以使用一个二维数组来存储节点之间的连接关系和权值。在使用DFS算法时,我们可以从图的某个节点开始,递归地遍历与该节点相邻的节点,并标记已经访问过的节点,直到遍历完整个图为止。在遍历过程中,我们可以使用一个栈来保存已经访问过的节点,以便在回溯时能够正确地返回上一个节点。
总之,C++中的DFS算法和邻接矩阵可以很好地结合使用,用于解决图的遍历和相关问题。如果您需要更详细的信息,可以参考相关的教材或者网上的资料。
相关推荐
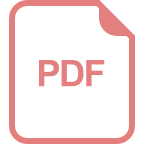












