mapreduce实现读取两个文件
时间: 2023-07-18 20:25:21 浏览: 82
可以通过以下步骤实现MapReduce程序读取两个文件:
1. 在Mapper类的setup()方法中获取第二个文件的路径,并将其存储在变量中。
2. 在Mapper类的map()方法中,首先读取第一个文件的数据,处理后输出键值对。
3. 然后读取第二个文件的数据,处理后输出键值对。
4. 在Reducer类中,收集所有键值对并进行相应的处理。
下面是一个简单的示例代码:
```java
public class TwoFilesMapReduce extends Mapper<LongWritable, Text, Text, IntWritable> {
private Path secondFilePath;
private BufferedReader reader;
@Override
protected void setup(Context context) throws IOException, InterruptedException {
// 获取第二个文件的路径
secondFilePath = new Path(context.getConfiguration().get("secondFilePath"));
FileSystem fs = FileSystem.get(context.getConfiguration());
FSDataInputStream in = fs.open(secondFilePath);
reader = new BufferedReader(new InputStreamReader(in, "UTF-8"));
}
@Override
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// 处理第一个文件的数据
// 输出键值对
// 处理第二个文件的数据
String line = reader.readLine();
while (line != null) {
// 处理数据
// 输出键值对
line = reader.readLine();
}
}
@Override
protected void cleanup(Context context) throws IOException, InterruptedException {
reader.close();
}
}
public class TwoFilesReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
@Override
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
// 处理键值对
// 输出结果
}
}
public class TwoFilesMapReduceDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
conf.set("secondFilePath", args[1]); // 设置第二个文件的路径
Job job = Job.getInstance(conf, "TwoFilesMapReduce");
job.setJarByClass(TwoFilesMapReduceDriver.class);
job.setMapperClass(TwoFilesMapReduce.class);
job.setReducerClass(TwoFilesReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0])); // 设置第一个文件的路径
FileOutputFormat.setOutputPath(job, new Path(args[2])); // 设置输出路径
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在运行MapReduce程序时,需要将第一个文件的路径、第二个文件的路径以及输出路径作为命令行参数传递给程序。
阅读全文
相关推荐
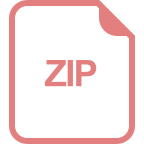
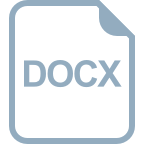
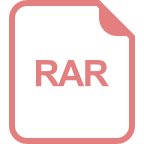


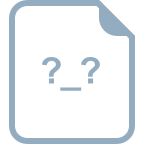
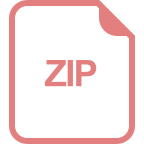
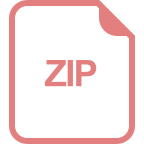
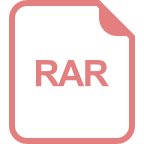
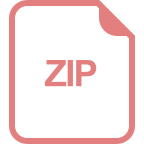
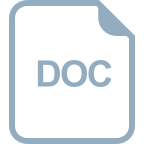
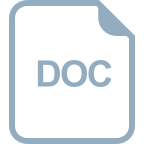
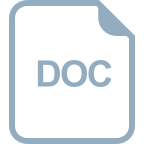
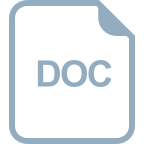
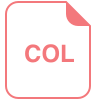



