给我一个python代码将含有坐标信息的json文件,转化为可用于分割训练的标签二值化黑白png图片
时间: 2023-04-03 22:00:51 浏览: 67
可以使用以下Python代码将含有坐标信息的JSON文件转化为可用于分割训练的标签二值化黑白PNG图片:
```python
import json
from PIL import Image, ImageDraw
# 读取JSON文件
with open('data.json', 'r') as f:
data = json.load(f)
# 获取图片大小
width = data['imageWidth']
height = data['imageHeight']
# 创建空白图片
img = Image.new('L', (width, height), )
# 绘制标签
draw = ImageDraw.Draw(img)
for shape in data['shapes']:
if shape['shape_type'] == 'polygon':
points = shape['points']
draw.polygon(points, fill=255)
# 保存图片
img.save('label.png')
```
其中,`data.json`是含有坐标信息的JSON文件的路径,`label.png`是生成的标签二值化黑白PNG图片的保存路径。这段代码会读取JSON文件中的坐标信息,并将其绘制在空白图片上,最后保存为PNG格式的图片。
相关问题
给我一个python代码用cv2的方法将含有坐标信息的json文件,转化为可用于分割训练的标签二值化黑白png图片
可以使用以下代码将含有坐标信息的json文件转化为标签二值化黑白png图片:
```python
import cv2
import json
import numpy as np
# 读取json文件
with open('json_file.json', 'r') as f:
data = json.load(f)
# 获取图片大小
img_size = (data['imageWidth'], data['imageHeight'])
# 创建空白图片
img = np.zeros((img_size[1], img_size[]), dtype=np.uint8)
# 遍历json文件中的每个坐标信息
for shape in data['shapes']:
# 获取坐标信息
points = np.array(shape['points'], np.int32)
# 将坐标信息转化为多边形
cv2.fillPoly(img, [points], 255)
# 保存标签二值化黑白png图片
cv2.imwrite('label.png', img)
```
其中,`json_file.json`为含有坐标信息的json文件的文件名,`label.png`为生成的标签二值化黑白png图片的文件名。
给我一个python代码将label中标注的json文件,转化为可用于分割训练的标签二值化黑白png图片
可以使用以下Python代码将标注的JSON文件转换为二值化的黑白PNG图像:
```python
import json
import numpy as np
from PIL import Image
# 读取JSON文件
with open('label.json', 'r') as f:
label = json.load(f)
# 获取图像大小
width = label['size']['width']
height = label['size']['height']
# 创建一个空白图像
img = np.zeros((height, width), dtype=np.uint8)
# 遍历每个对象
for obj in label['objects']:
# 获取对象的形状
shape = obj['shape_attributes']
# 如果是多边形
if shape['name'] == 'polygon':
# 获取多边形的顶点坐标
points = [(shape['all_points_x'][i], shape['all_points_y'][i]) for i in range(len(shape['all_points_x']))]
# 将多边形填充到图像中
Image.fromarray(img).draw.polygon(points, fill=1)
# 如果是矩形
elif shape['name'] == 'rect':
# 获取矩形的左上角和右下角坐标
x1, y1, x2, y2 = shape['x'], shape['y'], shape['x'] + shape['width'], shape['y'] + shape['height']
# 将矩形填充到图像中
Image.fromarray(img).draw.rectangle((x1, y1, x2, y2), fill=1)
# 保存图像
Image.fromarray(img).save('label.png')
```
其中,`label.json`是标注的JSON文件,`label.png`是生成的二值化黑白PNG图像。这段代码会遍历JSON文件中的每个对象,将多边形和矩形填充到空白图像中,并保存为PNG图像。
相关推荐
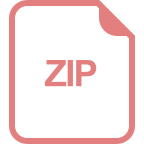
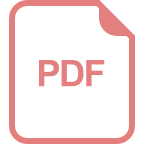
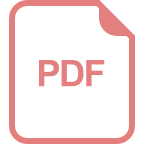




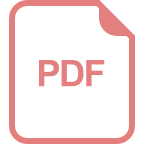
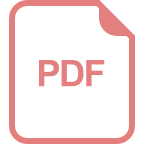
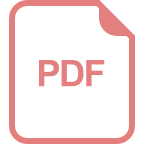
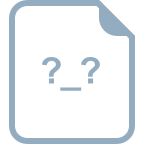
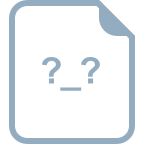
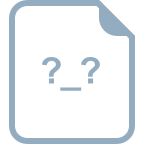
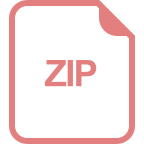
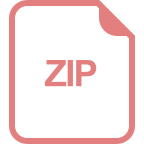