用java写一个把mysql数据导出excel表格的代码
时间: 2023-05-12 11:04:48 浏览: 65
好的,以下是一个用Java编写的将MySQL数据导出到Excel表格的示例代码:
```java
import java.io.FileOutputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class MySQLToExcel {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "mypassword";
String query = "SELECT * FROM mytable";
try (Connection con = DriverManager.getConnection(url, user, password);
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery(query);
XSSFWorkbook workbook = new XSSFWorkbook();
FileOutputStream out = new FileOutputStream("output.xlsx")) {
// Create a new sheet in the workbook
workbook.createSheet("Data");
// Get the header row and add it to the sheet
Row headerRow = workbook.getSheet("Data").createRow(0);
int columnCount = rs.getMetaData().getColumnCount();
for (int i = 1; i <= columnCount; i++) {
Cell cell = headerRow.createCell(i - 1);
cell.setCellValue(rs.getMetaData().getColumnName(i));
}
// Get the data rows and add them to the sheet
int rowNum = 1;
while (rs.next()) {
Row dataRow = workbook.getSheet("Data").createRow(rowNum++);
for (int i = 1; i <= columnCount; i++) {
Cell cell = dataRow.createCell(i - 1);
cell.setCellValue(rs.getString(i));
}
}
// Write the workbook to the output file
workbook.write(out);
System.out.println("Data exported successfully!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个代码使用了 Apache POI 库来创建和写入 Excel 文件。它首先连接到 MySQL 数据库,执行查询语句,然后将结果写入 Excel 文件。你需要将 `url`、`user`、`password` 和 `query` 变量替换为你自己的值,以便它可以连接到你的数据库并执行正确的查询。
相关推荐
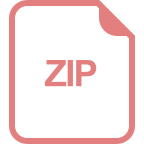
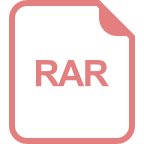














