python代码实现:Qt界面链接yolov5目标检测
时间: 2023-09-20 20:10:25 浏览: 123
以下是使用 PyQT5 实现 Qt 界面链接 YOLOv5 目标检测的 Python 代码示例:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton, QFileDialog
from PyQt5.QtGui import QPixmap
import cv2
from yolov5.detect import YOLOv5Detector
class App(QWidget):
def __init__(self):
super().__init__()
self.title = 'YOLOv5 Object Detection'
self.left = 50
self.top = 50
self.width = 640
self.height = 480
self.image_path = ''
self.initUI()
def initUI(self):
self.setWindowTitle(self.title)
self.setGeometry(self.left, self.top, self.width, self.height)
# 选择图像文件按钮
self.select_button = QPushButton('Select Image', self)
self.select_button.move(20, 20)
self.select_button.clicked.connect(self.select_image)
# 显示图像
self.image_label = QLabel(self)
self.image_label.move(20, 60)
self.image_label.resize(600, 400)
# 检测目标按钮
self.detect_button = QPushButton('Detect Objects', self)
self.detect_button.move(20, 470)
self.detect_button.clicked.connect(self.detect_objects)
self.show()
def select_image(self):
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_name, _ = QFileDialog.getOpenFileName(self, 'Select Image', '', 'Images (*.png *.xpm *.jpg *.bmp *.gif)', options=options)
if file_name:
self.image_path = file_name
pixmap = QPixmap(file_name)
self.image_label.setPixmap(pixmap)
self.image_label.setScaledContents(True)
def detect_objects(self):
if not self.image_path:
return
detector = YOLOv5Detector()
image = cv2.imread(self.image_path)
result_image, _ = detector.detect(image)
cv2.imwrite('result.jpg', result_image)
pixmap = QPixmap('result.jpg')
self.image_label.setPixmap(pixmap)
self.image_label.setScaledContents(True)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = App()
sys.exit(app.exec_())
```
其中 `yolov5.detect` 模块的代码可以参考 YOLOv5 的官方实现。在 `detect` 函数中,需要对输入的图像进行目标检测,并返回检测结果。在本例中,我们将检测结果保存为 `result.jpg`,并在界面中显示出来。
注意,需要将模型文件和权重文件放在适当的位置,并在 `YOLOv5Detector` 类中设置正确的路径。
阅读全文
相关推荐
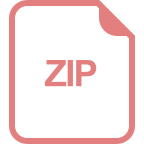
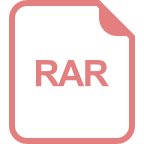
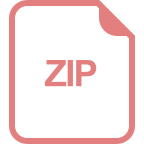
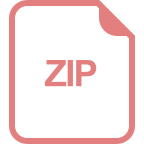
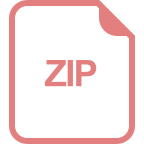
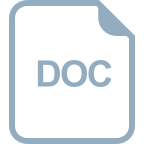




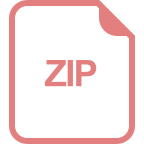
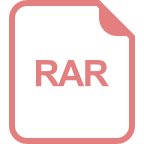
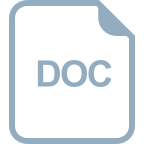
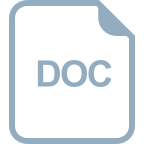
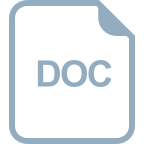



