编写“根据输入的字符串形式表示的广义表,创建广义表,在输出广义表“的c++代码
时间: 2024-02-24 09:53:09 浏览: 21
以下是基于递归实现的C++代码,可以实现根据输入的字符串形式表示的广义表,创建广义表,并输出广义表:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义广义表结点
struct GNode {
char data; // 数据域
GNode *subList; // 子表指针域
GNode *next; // 广义表中下一个元素的指针域
};
// 创建广义表
GNode* CreateGList(string str, int &i) {
GNode *head = new GNode; // 创建头结点
head->next = NULL;
GNode *p = head;
while (i < str.length()) {
char ch = str[i++];
if (ch == '(') {
p->subList = CreateGList(str, i); // 递归创建子表
} else if (ch == ')') {
return head;
} else if (ch == ',') {
continue;
} else {
GNode *newNode = new GNode; // 创建新结点
newNode->data = ch;
newNode->subList = NULL;
newNode->next = NULL;
p->next = newNode;
p = p->next;
}
}
return head;
}
// 输出广义表
void PrintGList(GNode *head) {
if (head == NULL) {
return;
}
if (head->data == '(') { // 如果是子表,递归输出子表
cout << '(';
PrintGList(head->subList);
cout << ')';
} else { // 如果是元素,输出元素值
cout << head->data;
}
if (head->next != NULL) { // 输出下一个元素
cout << ',';
PrintGList(head->next);
}
}
int main() {
string str = "(1,(2,3),4,(5,(6,7)))";
int i = 0;
GNode *head = CreateGList(str, i);
PrintGList(head);
cout << endl;
return 0;
}
```
在这个代码中,首先定义了一个广义表结点 `GNode`,其中包含数据域、子表指针域和下一个元素的指针域。然后定义了创建广义表的函数 `CreateGList`,它采用递归的方式,根据输入字符串逐个读取字符,如果是左括号则递归创建子表,如果是右括号则返回上一层,如果是逗号则继续读取下一个字符,如果是其他字符则创建新的广义表结点,并将其插入到广义表中。最后定义了输出广义表的函数 `PrintGList`,它也采用递归的方式,输出当前元素的值或子表,然后递归输出下一个元素。在 `main` 函数中,调用 `CreateGList` 函数创建广义表,然后调用 `PrintGList` 函数输出广义表。
相关推荐
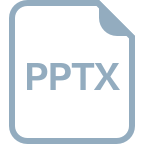
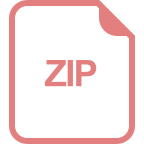
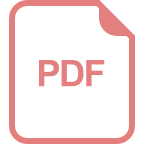














