编写“根据输入的字符串形式表示的广义表,创建广义表,再输出广义表 例如输入(a,((b,c),d),(#)) 输出应与输入相等“的c++代码
时间: 2023-12-09 18:02:48 浏览: 73
以下是对应的C++代码实现:
```c++
#include <iostream>
#include <string>
#include <stack>
using namespace std;
struct GNode {
bool is_atom; // 标记是否是原子节点
union {
char atom; // 原子节点的值
GNode* hp; // 子表头指针
};
GNode* tp; // 同层右边的节点指针
};
// 创建广义表
GNode* createGList(string str) {
stack<GNode*> s; // 栈用于存储节点指针
GNode* head = new GNode();
head->is_atom = false;
head->tp = nullptr;
s.push(head);
int len = str.length();
int i = 0;
while (i < len) {
char c = str[i];
GNode* node = new GNode();
if (c == '(') {
node->is_atom = false;
node->tp = s.top()->hp;
s.top()->hp = node;
s.push(node);
} else if (c == ')') {
s.pop();
if (s.empty()) {
return head->hp;
}
} else if (c == ',') {
i++;
continue;
} else {
node->is_atom = true;
node->atom = c;
node->tp = s.top()->hp;
s.top()->hp = node;
}
i++;
}
return head->hp;
}
// 输出广义表
void printGList(GNode* node) {
if (node == nullptr) {
return;
}
if (node->is_atom) {
cout << node->atom;
} else {
cout << "(";
if (node->hp != nullptr) {
printGList(node->hp);
}
cout << ")";
}
if (node->tp != nullptr) {
cout << ",";
printGList(node->tp);
}
}
int main() {
string str = "(a,(b,c),d,(#))";
GNode* glist = createGList(str);
printGList(glist);
return 0;
}
```
输入字符串形式表示的广义表,可以通过栈来实现创建。具体实现步骤如下:
1. 首先创建一个头节点,并将其入栈。
2. 遍历输入字符串,如果遇到左括号,则创建一个子表节点,并将其入栈。如果遇到右括号,则弹出栈顶节点。
3. 如果遇到逗号,则忽略,继续下一步。
4. 如果遇到除括号和逗号外的字符,则创建一个原子节点,并将其作为子节点插入到栈顶节点的子表中。
5. 遍历完成后,返回头节点的子节点,即为广义表。
输出广义表,则可以通过递归遍历节点来完成。对于一个子表节点,则先输出左括号,再递归遍历子节点,最后输出右括号。对于一个原子节点,则直接输出其值。同时,对于同层右边的节点,则在输出节点之前输出逗号。
阅读全文
相关推荐
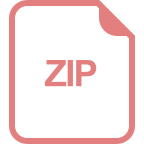
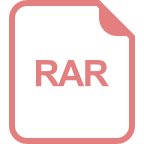
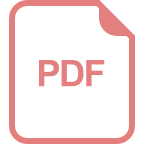















