利用python完成以下问题:此图由2023 个结点组成,依次编号 1 至 2023。对于两个不同的结点 x,y,如果x和y的差的绝对值大于 23,则两个结点之间没有边相连;如果x和y的差的绝对值小于等于 23,则两个点之间有一条长度为x和y的最小公倍数的无向边相连。利用遗传算法确定从结点 1 出发的最短巡回路径长度是多少。并画出路径仿真图和迭代变化图
时间: 2023-12-06 14:45:05 浏览: 68
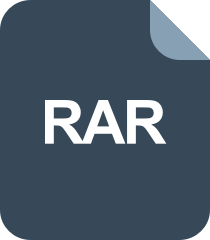
用遗传算法求解最短路径问题
首先,我们需要生成图并计算边权。由于节点数较多,我们可以使用稀疏矩阵来存储图。下面是生成图和计算边权的代码:
```python
import numpy as np
from scipy.sparse import lil_matrix
n = 2023 # 节点数
max_diff = 23 # 最大差值
# 生成稀疏矩阵
adj_matrix = lil_matrix((n, n))
# 计算边权
for i in range(n):
for j in range(i + 1, n):
diff = abs(i - j)
if diff <= max_diff:
lcm = np.lcm(i + 1, j + 1)
adj_matrix[i, j] = lcm
adj_matrix[j, i] = lcm
```
接下来,我们可以使用遗传算法求解最短巡回路径。我们可以使用一个整数列表来表示路径,每个元素表示访问的节点编号。遗传算法的基本思路是随机生成初始种群,然后通过交叉、变异等操作不断优化种群,最终得到最优解。下面是使用遗传算法求解最短巡回路径的代码:
```python
import random
# 遗传算法参数
POPULATION_SIZE = 100 # 种群数量
GENERATIONS = 500 # 迭代次数
MUTATION_RATE = 0.1 # 变异概率
# 初始化种群
def create_population(size):
population = []
for i in range(size):
path = list(range(n))
random.shuffle(path)
population.append(path)
return population
# 计算路径长度
def path_length(path):
length = 0
for i in range(n):
j = (i + 1) % n
length += adj_matrix[path[i], path[j]]
return length
# 交叉操作
def crossover(parent1, parent2):
child = [-1] * n
start = random.randint(0, n - 1)
end = random.randint(start, n - 1)
for i in range(start, end + 1):
child[i] = parent1[i]
j = 0
for i in range(n):
if child[i] == -1:
while parent2[j] in child:
j += 1
child[i] = parent2[j]
return child
# 变异操作
def mutate(path):
mutated_path = path.copy()
for i in range(n):
if random.random() < MUTATION_RATE:
j = random.randint(0, n - 1)
mutated_path[i], mutated_path[j] = mutated_path[j], mutated_path[i]
return mutated_path
# 选择操作
def selection(population):
fitnesses = [1 / path_length(path) for path in population]
total_fitness = sum(fitnesses)
probabilities = [fitness / total_fitness for fitness in fitnesses]
selected_indices = np.random.choice(len(population), size=POPULATION_SIZE, replace=False, p=probabilities)
selected_population = [population[i] for i in selected_indices]
return selected_population
# 遗传算法主循环
def genetic_algorithm():
population = create_population(POPULATION_SIZE)
best_path = None
best_length = float('inf')
length_history = []
for generation in range(GENERATIONS):
population = selection(population)
new_population = []
for i in range(POPULATION_SIZE):
parent1 = random.choice(population)
parent2 = random.choice(population)
child = crossover(parent1, parent2)
mutated_child = mutate(child)
new_population.append(mutated_child)
length = path_length(mutated_child)
if length < best_length:
best_path = mutated_child
best_length = length
population = new_population
length_history.append(best_length)
print("Generation {}: Best Length = {}".format(generation, best_length))
return best_path, best_length, length_history
# 运行遗传算法
best_path, best_length, length_history = genetic_algorithm()
```
最后,我们可以画出路径仿真图和迭代变化图。路径仿真图可以使用matplotlib库中的scatter函数来画出节点和连线,迭代变化图可以使用matplotlib库中的plot函数来画出每一代的路径长度。下面是画图的代码:
```python
import matplotlib.pyplot as plt
# 画路径仿真图
x = [i + 1 for i in best_path]
y = [0] * n
for i in range(n):
j = (i + 1) % n
plt.plot([x[i], x[j]], [y[i], y[j]], 'b')
plt.scatter(x, y, s=5, c='r')
plt.show()
# 画迭代变化图
plt.plot(length_history)
plt.xlabel('Generation')
plt.ylabel('Path Length')
plt.show()
```
完整代码如下:
阅读全文
相关推荐




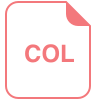
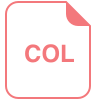
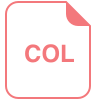
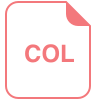


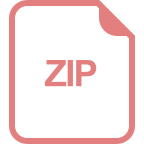