制作一个有顶层窗口式的单摆python程序
时间: 2024-03-25 18:38:46 浏览: 140
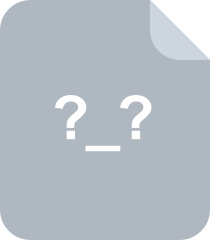
vpython单摆模拟运动
好的,以下是一个简单的单摆Python程序,使用了Tkinter库来实现有顶层窗口式的界面:
```python
import math
import tkinter as tk
class Pendulum:
def __init__(self, length, angle, dt=0.01, g=9.8):
self.length = length
self.angle = angle
self.dt = dt
self.g = g
self.velocity = 0
self.acceleration = 0
def update(self):
self.acceleration = -self.g/self.length * math.sin(self.angle)
self.velocity += self.acceleration * self.dt
self.angle += self.velocity * self.dt
def get_xy(self):
x = self.length * math.sin(self.angle)
y = self.length * math.cos(self.angle)
return x, y
class PendulumApp:
def __init__(self):
self.window = tk.Tk()
self.window.title("单摆程序")
self.canvas = tk.Canvas(self.window, width=500, height=500, bg="white")
self.canvas.pack()
self.pendulum = Pendulum(length=200, angle=math.pi/4)
self.draw_pendulum()
self.window.after(10, self.update)
self.window.mainloop()
def draw_pendulum(self):
x0 = 250
y0 = 50
x1, y1 = self.pendulum.get_xy()
self.canvas.delete("all")
self.canvas.create_line(x0, y0, x0+x1, y0+y1, width=2, fill="blue")
self.canvas.create_oval(x0-10, y0-10, x0+10, y0+10, fill="blue")
self.canvas.create_oval(x0+x1-10, y0+y1-10, x0+x1+10, y0+y1+10, fill="blue")
def update(self):
self.pendulum.update()
self.draw_pendulum()
self.window.after(10, self.update)
if __name__ == "__main__":
app = PendulumApp()
```
程序中定义了一个Pendulum类,表示单摆,包含了单摆的物理量和运动模拟方法。PendulumApp类则表示程序的窗口界面,使用了Tkinter库来实现。在程序运行时,窗口中会显示一个单摆的摆动过程。
阅读全文
相关推荐
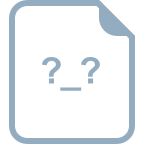
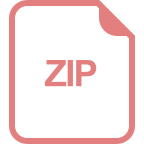















