keil5 define a struct
时间: 2024-03-22 11:42:16 浏览: 16
Sure, here's an example of how you can define a struct in Keil5:
```
// Define a struct called 'Person'
struct Person {
char name[20];
int age;
float height;
};
// Declare a variable of type 'Person'
struct Person person1;
```
In this example, we define a struct called 'Person' with three members: 'name', 'age', and 'height'. We then declare a variable called 'person1' of type 'Person'. You can access the members of the struct using the dot operator, like this: 'person1.name', 'person1.age', and 'person1.height'.
相关问题
keil5如何打印输出
在Keil5中,可以使用两种方法进行打印输出:通过HAL库和使用printf函数。
1. 通过HAL库打印输出:
- 首先,需要配置RCC和SYS,并设置USART1的参数。
- 然后,编写以下代码实现功能:
```
HAL_UART_Transmit(&huart1, (uint8_t *)&ch, 1, 0xFFFF);
```
2. 使用printf函数打印输出:
- 首先,进行与HAL库相同的配置。
- 添加头文件 "stdio.h" 和 "printf_task.h"。
- 在printf_task.c文件中添加以下代码:
```
typedef struct __FILE FILE;
#ifdef __GNUC__
#define PUTCHAR_PROTOTYPE int __io_putchar(int ch)
#else
#define PUTCHAR_PROTOTYPE int fputc(int ch, FILE *f)
#endif
PUTCHAR_PROTOTYPE {
HAL_UART_Transmit(&huart1, (uint8_t *)&ch, 1, 0xFFFF);
return ch;
}
```
以上两种方法均可实现Keil5中的打印输出功能。
keil uvision5蜂鸣器
在Keil uVision5中使用蜂鸣器可以通过以下步骤实现:
1. 首先,确保你的Keil uVision5已经正确安装并配置好了开发板和编译器。
2. 在Keil uVision5中创建一个新的工程或打开一个已有的工程。
3. 在工程中添加蜂鸣器的驱动程序或库文件。这些文件通常由硬件供应商提供,用于控制蜂鸣器的功能。
4. 在代码中引入蜂鸣器的头文件,并初始化蜂鸣器的引脚。
5. 使用适当的函数或方法来控制蜂鸣器的开关状态,以产生所需的声音。
下面是一个示例代码,演示了如何在Keil uVision5中使用蜂鸣器:
```c
#include <stdio.h>
#include <stm32f4xx.h> // 假设使用的是STM32F4系列的开发板
// 定义蜂鸣器引脚
#define BUZZER_PIN GPIO_Pin_0
#define BUZZER_PORT GPIOA
// 初始化蜂鸣器引脚
void buzzer_init(void) {
GPIO_InitTypeDef GPIO_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStruct.GPIO_Pin = BUZZER_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(BUZZER_PORT, &GPIO_InitStruct);
}
// 控制蜂鸣器发声
void buzzer_sound(uint32_t duration) {
GPIO_SetBits(BUZZER_PORT, BUZZER_PIN); // 打开蜂鸣器
// 延时一段时间,控制蜂鸣器发声的持续时间
for (uint32_t i = 0; i < duration; i++) {
for (uint32_t j = 0; j < 100000; j++);
}
GPIO_ResetBits(BUZZER_PORT, BUZZER_PIN); // 关闭蜂鸣器
}
int main(void) {
buzzer_init(); // 初始化蜂鸣器
while (1) {
buzzer_sound(1000); // 控制蜂鸣器发声,持续1秒
}
}
```
请注意,上述示例代码是基于STM32F4系列的开发板,使用了GPIO库来控制蜂鸣器的引脚。具体的硬件平台和蜂鸣器驱动程序可能会有所不同,你需要根据你的实际情况进行相应的修改。
相关推荐
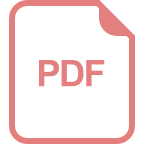












