编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班号和成绩,教师数据有编号、姓名、职称和部门。要求将编号、姓名输入和显示设计成一个类person,并作为学生数据作类student和教师数据操作类teacher的基类。
时间: 2024-06-09 09:06:20 浏览: 134
以下是一个可能的实现示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
int id;
string name;
public:
Person(int id, string name) {
this->id = id;
this->name = name;
}
void display() {
cout << "ID: " << id << endl;
cout << "Name: " << name << endl;
}
};
class Student : public Person {
private:
int classNumber;
float score;
public:
Student(int id, string name, int classNumber, float score) : Person(id, name) {
this->classNumber = classNumber;
this->score = score;
}
void display() {
Person::display();
cout << "Class Number: " << classNumber << endl;
cout << "Score: " << score << endl;
}
};
class Teacher : public Person {
private:
string title;
string department;
public:
Teacher(int id, string name, string title, string department) : Person(id, name) {
this->title = title;
this->department = department;
}
void display() {
Person::display();
cout << "Title: " << title << endl;
cout << "Department: " << department << endl;
}
};
int main() {
Student s(1, "Tom", 2, 90.5);
Teacher t(2, "Jane", "Associate Professor", "Computer Science");
cout << "Student Information:" << endl;
s.display();
cout << endl << "Teacher Information:" << endl;
t.display();
return 0;
}
```
这个程序定义了一个基类 `Person`,包含编号和姓名两个数据成员,以及一个 `display()` 方法用于显示编号和姓名。
然后定义了两个子类 `Student` 和 `Teacher`,它们都继承了 `Person` 类,并新增了一些数据成员和一个 `display()` 方法,用于显示所有数据。
在主函数中,我们创建了一个学生对象和一个教师对象,然后调用它们的 `display()` 方法来输出它们的数据。
注意,这里的 `display()` 方法在子类中覆盖了基类的实现,因此在调用时会优先调用子类的版本。在子类的 `display()` 方法中,我们通过调用基类的 `display()` 方法来显示编号和姓名。这种方式称为调用基类的成员函数,可以确保子类的 `display()` 方法能够完整地显示所有数据。
阅读全文
相关推荐
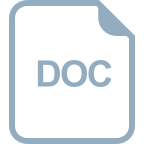
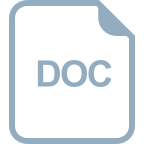
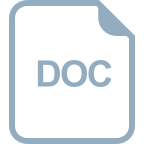












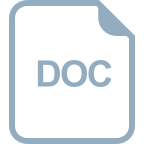
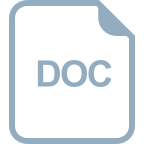
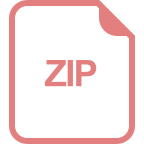