Unity 协程底层 如何自己实现一个协程
时间: 2024-03-10 15:50:16 浏览: 89
Unity协程的底层实现是基于C#的迭代器实现的。在C#中,使用yield关键字可以将方法转换为迭代器,通过迭代器可以实现协程的效果。Unity中的协程也是基于这个原理实现的。
如果要自己实现一个协程,可以按照以下步骤进行:
1. 定义一个委托,用于表示协程的执行体。
```csharp
public delegate IEnumerator CoroutineDelegate();
```
2. 定义一个协程类,保存协程的执行体和当前执行状态。
```csharp
public class Coroutine
{
private CoroutineDelegate m_CoroutineDelegate;
private IEnumerator m_Enumerator;
private bool m_IsDone;
public bool IsDone
{
get { return m_IsDone; }
}
public Coroutine(CoroutineDelegate coroutineDelegate)
{
m_CoroutineDelegate = coroutineDelegate;
m_Enumerator = m_CoroutineDelegate();
m_IsDone = false;
}
public void Update()
{
if (m_Enumerator != null && !m_IsDone)
{
if (!m_Enumerator.MoveNext())
{
m_IsDone = true;
}
}
}
}
```
3. 在需要使用协程的地方,创建一个协程对象并添加到一个协程管理器中。
```csharp
public class CoroutineManager : MonoBehaviour
{
private static CoroutineManager m_Instance;
private List<Coroutine> m_Coroutines = new List<Coroutine>();
public static CoroutineManager Instance
{
get
{
if (m_Instance == null)
{
m_Instance = new GameObject("CoroutineManager").AddComponent<CoroutineManager>();
}
return m_Instance;
}
}
private void Update()
{
for (int i = m_Coroutines.Count - 1; i >= 0; i--)
{
Coroutine coroutine = m_Coroutines[i];
coroutine.Update();
if (coroutine.IsDone)
{
m_Coroutines.RemoveAt(i);
}
}
}
public Coroutine StartCoroutine(CoroutineDelegate coroutineDelegate)
{
Coroutine coroutine = new Coroutine(coroutineDelegate);
m_Coroutines.Add(coroutine);
return coroutine;
}
}
```
4. 在协程中使用yield关键字来实现挂起和恢复。
```csharp
private IEnumerator MyCoroutine()
{
Debug.Log("Start Coroutine");
yield return null;
Debug.Log("Wait One Frame");
yield return new WaitForSeconds(1.0f);
Debug.Log("Wait One Second");
yield return new WaitForEndOfFrame();
Debug.Log("Wait End Of Frame");
}
```
以上就是一个简单的协程实现。注意,实际应用中还需要考虑协程的取消、异常处理等问题,需要根据具体需求进行扩展。
阅读全文
相关推荐
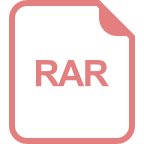
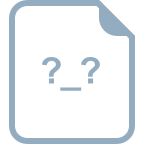

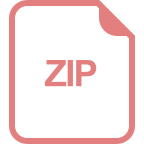
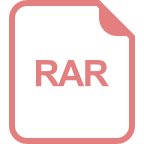
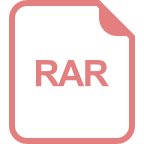
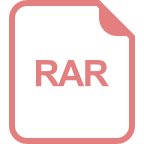
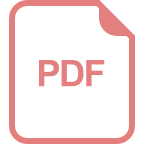
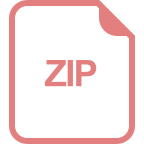
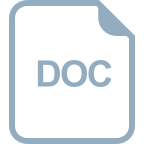
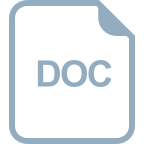
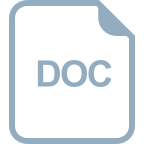
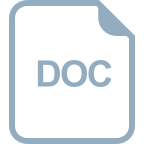
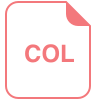
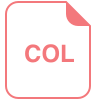
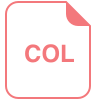
