用Python计算一个7*8的混淆矩阵的精确度、召回率、F1 score
时间: 2024-06-05 11:06:19 浏览: 126
假设混淆矩阵如下:
| | 预测为正类 | 预测为负类 |
|---------|------------|------------|
| 真实为正类 | 5 | 2 |
| 真实为负类 | 1 | 40 |
首先,我们需要计算出TP、FP、FN、TN:
- TP(True Positive):真实为正类,预测为正类,即5。
- FP(False Positive):真实为负类,预测为正类,即2。
- FN(False Negative):真实为正类,预测为负类,即1。
- TN(True Negative):真实为负类,预测为负类,即40。
然后,我们可以计算精确度、召回率、F1 score:
- 精确度(Precision):TP / (TP + FP) = 5 / (5 + 2) = 0.714。
- 召回率(Recall):TP / (TP + FN) = 5 / (5 + 1) = 0.833。
- F1 score:2 * Precision * Recall / (Precision + Recall) = 2 * 0.714 * 0.833 / (0.714 + 0.833) = 0.769。
相关问题
三分类使用python计算每一类的精确性召回率f1
在Python中,我们可以使用sklearn库来计算三分类任务的精确度、召回率和F1分数。假设我们有一个预测结果数组`y_pred`和实际标签`y_true`,它们都是0, 1, 和2表示的类别。以下是简单的步骤:
1. 首先,我们需要安装sklearn库,如果没有安装可以使用`pip install sklearn`。
```python
from sklearn.metrics import classification_report
# 假设 y_pred 是预测结果列表,y_true 是真实标签列表
y_pred = [0, 1, 2, 2, 0] # 预测
y_true = [0, 1, 1, 2, 0] # 真实值
# 将标签转换为整数以便于处理
y_true_int = list(map(lambda x: ord(x) - ord('0'), y_true))
# 计算分类报告
report = classification_report(y_true_int, y_pred, target_names=['Class 0', 'Class 1', 'Class 2'])
# 分割报告,提取每个类别的精确度(precision), 召回率(recall)和F1-score
precision = report['precision']
recall = report['recall']
f1_score = report['f1-score']
print(f"精确度:\n{precision}\n")
print(f"召回率:\n{recall}\n")
print(f"F1-Score:\n{f1_score}\n")
```
这里的`classification_report`函数会返回一个详细的分类报告,其中包括每个类别的各项指标。注意,这里假设`ord(x) - ord('0')`用于将字符标签转换为数字,对于其他语言的数据集可能需要相应的映射。
输出模型AUC、准确度、精确度、召回率、F1-score等参数以及ROC曲线、混淆矩阵等图形的代码
在Python中,特别是在机器学习库如scikit-learn中,评估模型性能通常涉及以下几个步骤:
1. **计算AUC (Area Under the Curve)**:
```python
from sklearn.metrics import roc_auc_score
y_true = ... # 真实标签
y_pred_proba = ... # 预测概率
auc = roc_auc_score(y_true, y_pred_proba)
```
2. **准确度 (Accuracy)**:
```python
accuracy = accuracy_score(y_true, y_pred) # y_pred 是预测的类别
```
3. **精确度 (Precision)**:
```python
precision = precision_score(y_true, y_pred)
```
4. **召回率 (Recall, Sensitivity or True Positive Rate)**:
```python
recall = recall_score(y_true, y_pred)
```
5. **F1-score**:
```python
f1 = f1_score(y_true, y_pred)
```
6. **绘制ROC曲线 (Receiver Operating Characteristic curve)**:
```python
import matplotlib.pyplot as plt
from sklearn.metrics import plot_roc_curve
plot_roc_curve(model, X_test, y_test)
plt.show()
```
7. **混淆矩阵 (Confusion Matrix)**:
```python
conf_mat = confusion_matrix(y_true, y_pred)
print(conf_mat)
```
以上代码假设`y_true`是实际标签列表,`y_pred`是预测结果,而`model`是你训练好的分类模型,`X_test`和`y_test`是测试集数据。
阅读全文
相关推荐
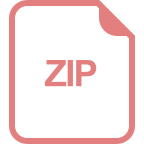
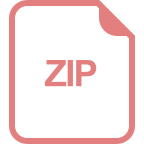
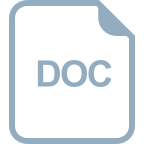
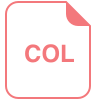
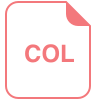
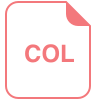
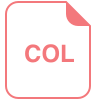








