编写分类性能度量的函数 my_metrics(y,y_predict), 要求(不使用 sklearn)返 回准确度、精确率、召回率和 F1 score。
时间: 2024-10-29 20:12:58 浏览: 48
编写一个自定义的分类性能度量函数 `my_metrics`,可以按照以下步骤完成:
首先,我们需要定义一些辅助函数用于计算精度(Precision)、召回率(Recall)和 F1 分数(F1 Score)。这些指标通常基于真正例(True Positives, TP)、假正例(False Positives, FP)、真反例(True Negatives, TN),以及假反例(False Negatives, FN)。
```python
def true_positives(TP):
return TP
def false_positives(FP):
return FP
def true_negatives(TN):
return TN
def false_negatives(FN):
return FN
def precision(TP, FP):
if TP + FP == 0:
return 0
else:
return TP / (TP + FP)
def recall(TP, FN):
if TP + FN == 0:
return 0
else:
return TP / (TP + FN)
def f1_score(precision_, recall_):
if precision_ + recall_ == 0:
return 0
else:
return 2 * precision_ * recall_ / (precision_ + recall_)
```
然后,我们可以设计 `my_metrics` 函数接收真实标签 `y` 和预测结果 `y_predict`,并计算上述四个值:
```python
def my_metrics(y, y_predict):
# 确定每个类别的真值和预测值
labels = set(y)
TP = {label: 0 for label in labels}
FP = {label: 0 for label in labels}
TN = {label: 0 for label in labels}
FN = {label: 0 for label in labels}
for i in range(len(y)):
true_label = y[i]
pred_label = y_predict[i]
if true_label == pred_label:
if true_label == 1: # 只对二分类任务处理,其他分类任务需要调整这部分
TP[true_label] += 1
else:
TN[true_label] += 1
elif true_label == 0 and pred_label == 1:
FP[true_label] += 1
elif true_label == 1 and pred_label == 0:
FN[true_label] += 1
accuracy = sum(TP.values()) + TN.values() / len(y)
overall_precision = precision(sum(TP.values()), sum(FP.values()))
overall_recall = recall(sum(TP.values()), sum(FN.values()))
average_f1_scores = {}
for label, tp in TP.items():
if tp > 0:
precision_ = precision(tp, FP[label])
recall_ = recall(tp, FN[label])
average_f1_scores[label] = f1_score(precision_, recall_)
return accuracy, overall_precision, overall_recall, average_f1_scores
```
注意:这个函数适用于二分类任务。对于多分类任务,你需要调整 TP、FP、TN、FN 的计数,以及 F1 分数的部分,以便分别计算每个类别的指标。
阅读全文
相关推荐
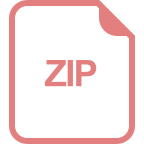
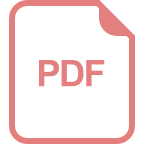
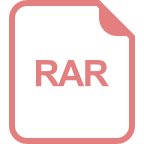


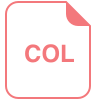
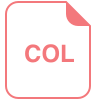
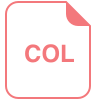
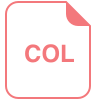
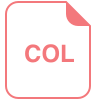
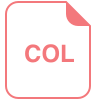
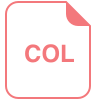
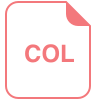
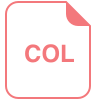
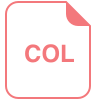
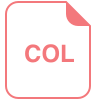
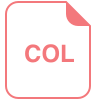
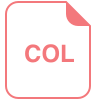
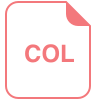