javafx.animation中keyvalue
时间: 2024-06-08 12:07:06 浏览: 99
JavaFX中的KeyValue类表示一个属性在动画中的值,它会在指定的时间点上设置该属性的值。KeyValue类包含两个属性:key和value。key是一个Object类型,表示要设置的属性;value是一个Object类型,表示在指定的时间点上要设置的属性值。
例如,以下代码创建一个KeyValue对象,将一个矩形的宽度从100像素变为200像素:
```
KeyValue keyValue = new KeyValue(rectangle.widthProperty(), 200);
```
在使用KeyValue时,通常需要将多个KeyValue对象组合成一个KeyFrame对象,然后将多个KeyFrame对象组合成一个动画。例如,以下代码创建一个动画,将一个矩形的宽度从100像素变为200像素,持续时间为1秒:
```
KeyFrame keyFrame = new KeyFrame(Duration.seconds(1),
new KeyValue(rectangle.widthProperty(), 200));
Timeline timeline = new Timeline(keyFrame);
timeline.play();
```
相关问题
import javafx.animation.PathTransition; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.shape.Ellipse; import javafx.stage.Stage; import javafx.util.Duration; public class FXTest6 extends Application{ public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) throws Exception { Pane rootNode=new Pane(); Ellipse ellipse=new Ellipse(300,200,100,50); ellipse.setFill(Color.WHITE); ellipse.setStroke(Color.BLACK); Circle circle=new Circle(250,200,20); circle.setFill(Color.BLACK); PathTransition pt=new PathTransition(Duration.millis(4500),ellipse,circle); pt.play(); rootNode.getChildren().addAll(ellipse,circle); Scene scene =new Scene(rootNode,600,400); primaryStage.setTitle("移动动画"); primaryStage.setScene(scene); primaryStage.show(); } }我想让圆循环播放且可以控制旋转方向
你可以使用Timeline类来实现圆的循环播放,并且可以通过设置旋转方向来控制圆的旋转方向。以下是修改后的代码:
```
import javafx.animation.Animation;
import javafx.animation.KeyFrame;
import javafx.animation.KeyValue;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Ellipse;
import javafx.stage.Stage;
import javafx.util.Duration;
public class FXTest6 extends Application{
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
Pane rootNode=new Pane();
Ellipse ellipse=new Ellipse(300,200,100,50);
ellipse.setFill(Color.WHITE);
ellipse.setStroke(Color.BLACK);
Circle circle=new Circle(250,200,20);
circle.setFill(Color.BLACK);
PathTransition pt=new PathTransition(Duration.millis(4500),ellipse,circle);
pt.play();
Timeline timeline = new Timeline();
timeline.setCycleCount(Animation.INDEFINITE); // 设置循环播放
timeline.setAutoReverse(true); // 设置自动反转
KeyValue keyValue = new KeyValue(circle.rotateProperty(), 360);
KeyFrame keyFrame = new KeyFrame(Duration.seconds(2), keyValue);
timeline.getKeyFrames().add(keyFrame);
timeline.play();
rootNode.getChildren().addAll(ellipse,circle);
Scene scene =new Scene(rootNode,600,400);
primaryStage.setTitle("移动动画");
primaryStage.setScene(scene);
primaryStage.show();
}
}
```
在上述代码中,我们创建了一个Timeline对象,并将其循环播放,并且设置了自动反转。我们使用KeyValue和KeyFrame类来定义圆的旋转动画,其中KeyValue类用于定义属性的起始值和结束值,KeyFrame类则用于定义关键帧。我们将关键帧添加到Timeline对象中,并播放动画。你可以根据需要修改Duration和KeyValue的值以控制旋转速度和方向。
javafx中keyValue具体的用法
JavaFX中的KeyValue类表示一个属性的键值对。它通常用于在动画中设置属性的值,例如设置一个节点的透明度或位置。
下面是一个简单的例子,展示了如何使用KeyValue在动画中改变一个节点的透明度:
```
import javafx.animation.KeyFrame;
import javafx.animation.KeyValue;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import javafx.util.Duration;
public class KeyValueExample extends Application {
@Override
public void start(Stage primaryStage) {
Rectangle rect = new Rectangle(100, 100);
rect.setFill(Color.BLUE);
StackPane root = new StackPane(rect);
Scene scene = new Scene(root, 300, 250);
Timeline timeline = new Timeline();
KeyValue keyValue = new KeyValue(rect.opacityProperty(), 0.0);
KeyFrame keyFrame = new KeyFrame(Duration.seconds(2), keyValue);
timeline.getKeyFrames().add(keyFrame);
timeline.play();
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
在这个例子中,我们创建了一个矩形,并将其添加到一个StackPane容器中。然后,我们创建了一个Timeline对象,并向其中添加了一个KeyFrame。这个KeyFrame中包含了一个KeyValue对象,它将矩形的透明度属性从1.0变为0.0,持续时间为2秒。最后,我们调用timeline.play()方法来启动动画。
需要注意的是,每个KeyValue对象都与一个具体的属性相关联。在上面的例子中,我们使用了rect.opacityProperty()来获取矩形的透明度属性,并将其传递给了KeyValue构造函数。如果您想改变其他属性,就需要使用相应的属性方法来获取它们的属性对象。
希望这个例子可以帮助您更好地理解JavaFX中KeyValue的用法。
阅读全文
相关推荐
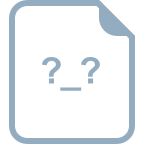













