python写个俄罗斯方块游戏
时间: 2023-08-24 14:09:55 浏览: 63
好的,下面是一个简单的 Python 俄罗斯方块游戏的代码示例:
```
import pygame
import random
# 初始化游戏
pygame.init()
# 设置游戏窗口大小
WIDTH = 800
HEIGHT = 600
SCREEN = pygame.display.set_mode((WIDTH, HEIGHT))
# 定义游戏颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 设置游戏字体
FONT = pygame.font.SysFont(None, 50)
# 定义方块大小和行列数
BLOCK_SIZE = 30
ROWS = HEIGHT // BLOCK_SIZE
COLS = WIDTH // BLOCK_SIZE
# 定义方块类
class Block:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
def draw(self):
pygame.draw.rect(SCREEN, self.color, (self.x * BLOCK_SIZE, self.y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 定义俄罗斯方块类
class Tetromino:
def __init__(self):
self.x = COLS // 2
self.y = 0
self.color = random.choice([RED, GREEN, BLUE])
self.blocks = []
self.shapes = [
[(0, 0), (1, 0), (2, 0), (3, 0)], # I
[(0, 0), (1, 0), (0, 1), (1, 1)], # O
[(0, 0), (1, 0), (2, 0), (2, 1)], # L
[(0, 0), (1, 0), (2, 0), (0, 1)], # J
[(1, 0), (2, 0), (0, 1), (1, 1)], # S
[(0, 0), (1, 0), (1, 1), (2, 1)], # Z
[(0, 1), (1, 1), (2, 1), (1, 0)] # T
]
self.shape = random.choice(self.shapes)
for pos in self.shape:
block = Block(self.x + pos[0], self.y + pos[1], self.color)
self.blocks.append(block)
def move_down(self):
for block in self.blocks:
block.y += 1
self.y += 1
def move_left(self):
if self.x > 0:
for block in self.blocks:
block.x -= 1
self.x -= 1
def move_right(self):
if self.x < COLS - max(pos[0] for pos in self.shape):
for block in self.blocks:
block.x += 1
self.x += 1
def rotate(self):
if self.shape != self.shapes[1]:
new_shape = []
for pos in self.shape:
new_pos = (-pos[1], pos[0])
new_shape.append(new_pos)
max_x = max(pos[0] for pos in new_shape)
min_x = min(pos[0] for pos in new_shape)
delta_x = self.x - (max_x + min_x) // 2
self.shape = new_shape
for i, block in enumerate(self.blocks):
block.x = self.x + self.shape[i][0] + delta_x
block.y = self.y + self.shape[i][1]
def draw(self):
for block in self.blocks:
block.draw()
# 定义游戏主循环
def main():
tetromino = Tetromino()
clock = pygame.time.Clock()
speed = 1
score = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
tetromino.move_left()
elif event.key == pygame.K_RIGHT:
tetromino.move_right()
elif event.key == pygame.K_UP:
tetromino.rotate()
SCREEN.fill(BLACK)
if tetromino.y < ROWS - max(pos[1] for pos in tetromino.shape):
tetromino.move_down()
else:
for block in tetromino.blocks:
if block.y < 0:
pygame.quit()
quit()
for block in tetromino.blocks:
x, y = block.x, block.y
if y >= 0:
grid[y][x] = block
tetromino = Tetromino()
for y in range(ROWS):
for x in range(COLS):
if grid[y][x]:
grid[y][x].draw()
tetromino.draw()
score_text = FONT.render("Score: " + str(score), True, WHITE)
SCREEN.blit(score_text, (10, 10))
pygame.display.update()
clock.tick(60)
# 启动游戏
if __name__ == "__main__":
grid = [[None for _ in range(COLS)] for _ in range(ROWS)]
main()
```
这是一个基本的俄罗斯方块游戏,你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
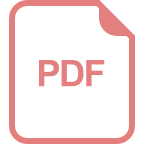
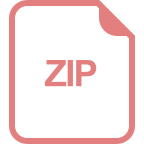
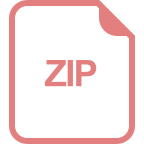










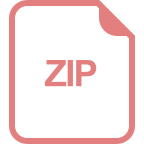
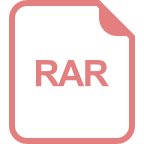
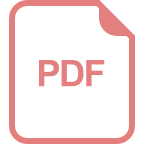
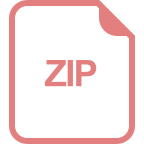
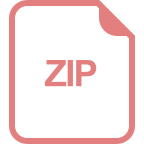
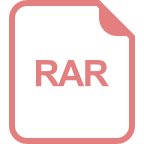
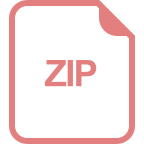