用python写个俄罗斯方块程序
时间: 2023-05-27 11:04:36 浏览: 59
这是一个简单的俄罗斯方块程序,使用Python的pygame模块实现。
```
import pygame
import random
# 游戏窗口大小
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 500
# 游戏方格大小
BLOCK_SIZE = 20
# 方块形状
SHAPES = [[[1, 1, 1, 1]],
[[1, 1],
[1, 1]],
[[1, 1, 0],
[0, 1, 1]],
[[0, 1, 1],
[1, 1, 0]],
[[1, 0, 0],
[1, 1, 1]],
[[0, 0, 1],
[1, 1, 1]],
[[1, 1, 1],
[0, 0, 1]]]
# 方块颜色
COLORS = [(0, 0, 0), (255, 255, 255), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255)]
class Block:
def __init__(self):
self.shape = random.choice(SHAPES)
self.color = random.choice(COLORS)
self.x = WINDOW_WIDTH // 2 - BLOCK_SIZE * len(self.shape[0]) // 2
self.y = 0
def move(self, dx, dy):
self.x += dx
self.y += dy
def rotate(self):
# 旋转矩阵
new_shape = []
for i in range(len(self.shape[0])):
new_row = []
for j in range(len(self.shape)):
new_row.append(self.shape[j][i])
new_shape.append(new_row[::-1])
self.shape = new_shape
def draw(self, surface):
for i in range(len(self.shape)):
for j in range(len(self.shape[0])):
if self.shape[i][j] == 1:
pygame.draw.rect(surface, self.color, (self.x+j*BLOCK_SIZE, self.y+i*BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
def get_rect(self):
return pygame.Rect(self.x, self.y, BLOCK_SIZE*len(self.shape[0]), BLOCK_SIZE*len(self.shape))
class Game:
def __init__(self):
pygame.init()
self.surface = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('俄罗斯方块')
self.clock = pygame.time.Clock()
self.current_block = Block()
self.next_block = Block()
self.grid = [[0 for _ in range(WINDOW_WIDTH // BLOCK_SIZE)] for _ in range(WINDOW_HEIGHT // BLOCK_SIZE)]
self.score = 0
def run(self):
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
self.move_current_block(-BLOCK_SIZE, 0)
elif event.key == pygame.K_RIGHT:
self.move_current_block(BLOCK_SIZE, 0)
elif event.key == pygame.K_UP:
self.rotate_current_block()
elif event.key == pygame.K_DOWN:
self.move_current_block(0, BLOCK_SIZE)
self.draw()
if not self.move_current_block(0, BLOCK_SIZE):
self.handle_full_rows()
if self.current_block.get_rect().top <= 0:
running = False
else:
self.current_block = self.next_block
self.current_block.x = WINDOW_WIDTH // 2 - BLOCK_SIZE * len(self.current_block.shape[0]) // 2
self.current_block.y = 0
self.next_block = Block()
pygame.display.update()
self.clock.tick(10)
pygame.quit()
def draw_grid(self):
for i in range(WINDOW_WIDTH // BLOCK_SIZE):
pygame.draw.line(self.surface, (127, 127, 127), (i*BLOCK_SIZE, 0), (i*BLOCK_SIZE, WINDOW_HEIGHT))
for i in range(WINDOW_HEIGHT // BLOCK_SIZE):
pygame.draw.line(self.surface, (127, 127, 127), (0, i*BLOCK_SIZE), (WINDOW_WIDTH, i*BLOCK_SIZE))
def draw(self):
self.surface.fill((255, 255, 255))
self.draw_grid()
for i in range(len(self.grid)):
for j in range(len(self.grid[0])):
if self.grid[i][j] != 0:
pygame.draw.rect(self.surface, COLORS[self.grid[i][j]], (j*BLOCK_SIZE, i*BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
self.current_block.draw(self.surface)
self.next_block.draw(self.surface)
font = pygame.font.SysFont(None, 24)
text = font.render('得分: {}'.format(self.score), True, (0, 0, 0))
self.surface.blit(text, (10, 10))
def move_current_block(self, dx, dy):
new_rect = self.current_block.get_rect().move(dx, dy)
if new_rect.left < 0 or new_rect.right > WINDOW_WIDTH or new_rect.bottom > WINDOW_HEIGHT:
return False
for i in range(len(self.grid)):
for j in range(len(self.grid[0])):
if new_rect.colliderect(pygame.Rect(j*BLOCK_SIZE, i*BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)) and self.grid[i][j] != 0:
return False
self.current_block.move(dx, dy)
return True
def rotate_current_block(self):
self.current_block.rotate()
new_rect = self.current_block.get_rect()
if new_rect.left < 0 or new_rect.right > WINDOW_WIDTH or new_rect.bottom > WINDOW_HEIGHT:
self.current_block.rotate()
else:
for i in range(len(self.grid)):
for j in range(len(self.grid[0])):
if new_rect.colliderect(pygame.Rect(j*BLOCK_SIZE, i*BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)) and self.grid[i][j] != 0:
self.current_block.rotate()
return
def handle_full_rows(self):
for i in range(len(self.grid)):
if all(self.grid[i]):
for j in range(i, 0, -1):
self.grid[j] = self.grid[j-1][:]
self.grid[0] = [0 for _ in range(len(self.grid[0]))]
self.score += 10
if __name__ == '__main__':
game = Game()
game.run()
```
运行后,你可以看到一个俄罗斯方块游戏界面。使用左右箭头控制方块左右移动,上箭头控制方块旋转,下箭头控制方块加速下落。当一行方块被填满时,该行会消失并累加得分。当方块堆积到最高行时,游戏结束。
相关推荐
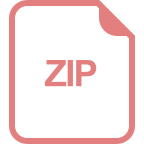
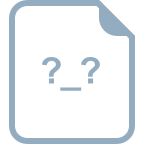
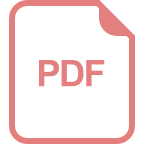














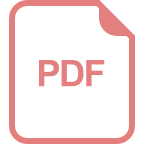