用python写一个俄罗斯方块程序
时间: 2023-09-26 20:07:32 浏览: 91
好的,以下是一个简单的Python俄罗斯方块程序示例代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 游戏窗口大小
width, height = 500, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption('俄罗斯方块')
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
CYAN = (0, 255, 255)
MAGENTA = (255, 0, 255)
# 定义方块大小
block_size = 25
# 定义游戏区域
play_width = 10 * block_size
play_height = 20 * block_size
play_x = width // 2 - play_width // 2
play_y = height - play_height - 10
# 定义游戏区域边框
border_width = 4
# 定义字体
font = pygame.font.SysFont(None, 30)
# 定义方块形状
shapes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6],
[6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块颜色
colors = [
GRAY,
BLUE,
YELLOW,
RED,
CYAN,
MAGENTA,
GREEN
]
# 定义方块类
class Block:
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
self.color = colors[shapes.index(shape)]
self.rotation = 0
# 定义游戏区域数组
play_area = [[0 for _ in range(10)] for _ in range(20)]
# 绘制游戏区域
def draw_play_area():
pygame.draw.rect(screen, WHITE, (play_x - border_width, play_y - border_width, play_width + border_width * 2, play_height + border_width * 2))
pygame.draw.rect(screen, BLACK, (play_x, play_y, play_width, play_height))
for y in range(20):
for x in range(10):
if play_area[y][x] != 0:
pygame.draw.rect(screen, colors[play_area[y][x] - 1], (play_x + x * block_size, play_y + y * block_size, block_size, block_size), 0)
pygame.draw.rect(screen, BLACK, (play_x + x * block_size, play_y + y * block_size, block_size, block_size), 1)
# 绘制方块
def draw_block(block):
for y in range(len(block.shape)):
for x in range(len(block.shape[0])):
if block.shape[y][x] != 0:
pygame.draw.rect(screen, block.color, (play_x + (block.x + x) * block_size, play_y + (block.y + y) * block_size, block_size, block_size), 0)
pygame.draw.rect(screen, BLACK, (play_x + (block.x + x) * block_size, play_y + (block.y + y) * block_size, block_size, block_size), 1)
# 判断方块是否可以移动
def can_move(block, dx, dy):
for y in range(len(block.shape)):
for x in range(len(block.shape[0])):
if block.shape[y][x] != 0:
if block.y + y + dy < 0:
return False
if block.x + x + dx < 0 or block.x + x + dx >= 10:
return False
if play_area[block.y + y + dy][block.x + x + dx] != 0:
return False
return True
# 判断方块是否可以旋转
def can_rotate(block):
new_shape = [list(row) for row in block.shape]
for i in range(len(block.shape)):
for j in range(len(block.shape[0])):
new_shape[j][len(block.shape) - 1 - i] = block.shape[i][j]
for y in range(len(new_shape)):
for x in range(len(new_shape[0])):
if new_shape[y][x] != 0:
if block.y + y < 0:
return False
if block.x + x < 0 or block.x + x >= 10:
return False
if play_area[block.y + y][block.x + x] != 0:
return False
return True
# 将方块放到游戏区域中
def place_block(block):
for y in range(len(block.shape)):
for x in range(len(block.shape[0])):
if block.shape[y][x] != 0:
play_area[block.y + y][block.x + x] = shapes.index(block.shape) + 1
# 消除满行
def clear_lines():
lines_cleared = 0
for y in range(len(play_area)):
if all(play_area[y]):
play_area.pop(y)
play_area.insert(0, [0 for _ in range(10)])
lines_cleared += 1
return lines_cleared
# 绘制得分
def draw_score(score):
text = font.render('得分: ' + str(score), True, WHITE)
screen.blit(text, (10, 10))
# 开始游戏
def start_game():
# 初始化游戏
game_over = False
block = Block(3, 0, random.choice(shapes))
next_block = Block(3, 0, random.choice(shapes))
score = 0
# 游戏循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT and can_move(block, -1, 0):
block.x -= 1
if event.key == pygame.K_RIGHT and can_move(block, 1, 0):
block.x += 1
if event.key == pygame.K_DOWN and can_move(block, 0, 1):
block.y += 1
if event.key == pygame.K_UP and can_rotate(block) :
block.rotation = (block.rotation + 1) % len(block.shape)
block.shape = [list(row) for row in zip(*block.shape[::-1])]
# 自动下落
if can_move(block, 0, 1):
block.y += 1
else:
place_block(block)
lines_cleared = clear_lines()
score += lines_cleared * 100
if lines_cleared > 0:
pygame.time.delay(100)
block = next_block
next_block = Block(3, 0, random.choice(shapes))
if not can_move(block, 0, 0):
game_over = True
# 绘制游戏界面
screen.fill(BLACK)
draw_play_area()
draw_block(block)
draw_block(next_block)
draw_score(score)
pygame.display.update()
# 控制游戏速度
pygame.time.delay(50)
# 退出游戏
pygame.quit()
# 开始游戏
start_game()
```
运行程序后,它将启动一个简单的俄罗斯方块游戏,玩家可以使用方向键控制方块的移动和旋转,目标是在游戏区域中放置方块并消除满行。注意,此代码只是一个简单的示例,还有很多地方可以进行改进和扩展,比如添加音效、增加难度等等。
阅读全文
相关推荐
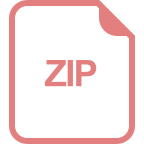
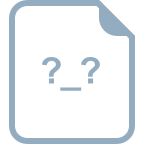
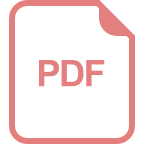















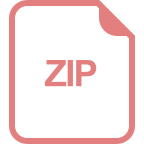