请写出关于ros1的程序填空题
时间: 2024-02-16 17:02:34 浏览: 66
好的,下面是五道关于ROS1程序的填空题:
1. 编写一个ROS节点,发布一个名为"/chatter"的字符串消息,其中的数据为"Hello, ROS!"。请填写下列程序的空白部分。
```python
#!/usr/bin/env python
import rospy
from std_msgs.msg import String
def talker():
pub = rospy.Publisher(_____, String, queue_size=10)
rospy.init_node(_____, anonymous=True)
rate = rospy.Rate(10) # 10hz
while not rospy.is_shutdown():
hello_str = "Hello, ROS!"
rospy.loginfo(hello_str)
pub.publish(hello_str)
rate.sleep()
if __name__ == '__main__':
try:
talker()
except rospy.ROSInterruptException:
pass
```
答案:
```python
pub = rospy.Publisher('/chatter', String, queue_size=10)
rospy.init_node('talker', anonymous=True)
```
2. 编写一个ROS节点,订阅名为"/chatter"的字符串消息,并将其输出到终端。请填写下列程序的空白部分。
```python
#!/usr/bin/env python
import rospy
from std_msgs.msg import String
def callback(data):
rospy.loginfo(rospy.get_caller_id() + "I heard %s", data.data)
def listener():
rospy.init_node(_____, anonymous=True)
rospy.Subscriber(_____, String, callback)
rospy.spin()
if __name__ == '__main__':
listener()
```
答案:
```python
rospy.init_node('listener', anonymous=True)
rospy.Subscriber('/chatter', String, callback)
```
3. 编写一个ROS服务,输入两个整数,返回它们的和。请填写下列程序的空白部分。
```python
#!/usr/bin/env python
import rospy
from beginner_tutorials.srv import AddTwoInts, AddTwoIntsResponse
def handle_add_two_ints(req):
print("Returning [%s + %s = %s]"%(req.a, req.b, (req.a + req.b)))
return AddTwoIntsResponse(req.a + req.b)
def add_two_ints_server():
rospy.init_node(_____)
s = rospy.Service('add_two_ints', AddTwoInts, handle_add_two_ints)
print("Ready to add two ints.")
rospy.spin()
if __name__ == "__main__":
add_two_ints_server()
```
答案:
```python
rospy.init_node('add_two_ints_server')
```
4. 编写一个ROS节点,读取名为"/camera/image_raw"的图像消息,将其转换为OpenCV格式并进行处理,最后将处理后的图像发布到名为"/processed_image"的话题上。请填写下列程序的空白部分。
```python
#!/usr/bin/env python
import rospy
import cv2
from sensor_msgs.msg import Image
from cv_bridge import CvBridge, CvBridgeError
def image_callback(data):
bridge = CvBridge()
cv_image = bridge.imgmsg_to_cv2(data, "bgr8")
# 处理图像
# ...
pub = rospy.Publisher(_____, Image, queue_size=10)
try:
pub.publish(bridge.cv2_to_imgmsg(cv_image, "bgr8"))
except CvBridgeError as e:
print(e)
def main():
rospy.init_node(_____)
rospy.Subscriber(_____, Image, image_callback)
rospy.spin()
if __name__ == '__main__':
main()
```
答案:
```python
pub = rospy.Publisher('/processed_image', Image, queue_size=10)
rospy.init_node('image_processor')
rospy.Subscriber('/camera/image_raw', Image, image_callback)
```
5. 编写一个ROS节点,使用MoveIt控制机械臂运动到预定位置。请填写下列程序的空白部分。
```python
#!/usr/bin/env python
import rospy
import moveit_commander
from moveit_msgs.msg import RobotTrajectory
from trajectory_msgs.msg import JointTrajectoryPoint
def moveit_move_to_joint_goal(joint_goal):
moveit_commander.roscpp_initialize(sys.argv)
robot = moveit_commander.RobotCommander()
group = moveit_commander.MoveGroupCommander(_____)
group.set_joint_value_target(joint_goal)
plan = group.plan()
traj = RobotTrajectory()
traj.joint_trajectory = plan.joint_trajectory
pub = rospy.Publisher(_____, RobotTrajectory, queue_size=10)
pub.publish(traj)
if __name__ == '__main__':
try:
rospy.init_node(_____)
joint_goal = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0]
moveit_move_to_joint_goal(joint_goal)
except rospy.ROSInterruptException:
pass
```
答案:
```python
group = moveit_commander.MoveGroupCommander('arm')
pub = rospy.Publisher('/robot_trajectory', RobotTrajectory, queue_size=10)
rospy.init_node('moveit_test')
```
阅读全文
相关推荐
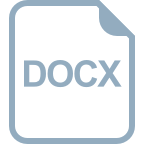
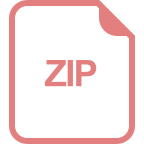
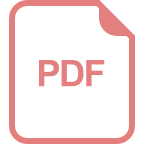
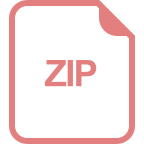
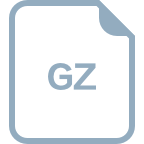
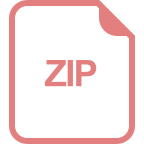
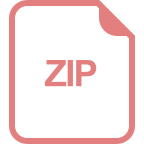
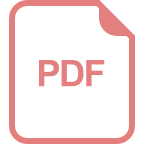
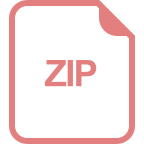
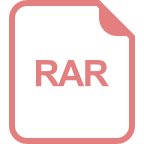
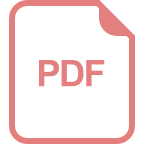
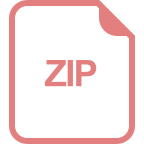