用unity实现第三人称相机控制脚本
时间: 2023-03-21 09:03:21 浏览: 142
可以使用以下脚本来实现Unity中第三人称相机的控制:
```
using UnityEngine;
public class ThirdPersonCamera : MonoBehaviour
{
public Transform target; // 跟随的目标物体
public float distance = 5.0f; // 相机与目标物体的距离
public float height = 2.0f; // 相机距离目标物体的高度
public float damping = 2.0f; // 相机移动的平滑度
public bool smoothRotation = true; // 是否平滑旋转
void LateUpdate()
{
if (!target) return;
float wantedRotationAngle = target.eulerAngles.y;
float wantedHeight = target.position.y + height;
float currentRotationAngle = transform.eulerAngles.y;
float currentHeight = transform.position.y;
currentRotationAngle = Mathf.LerpAngle(currentRotationAngle, wantedRotationAngle, damping * Time.deltaTime);
currentHeight = Mathf.Lerp(currentHeight, wantedHeight, damping * Time.deltaTime);
Quaternion currentRotation = Quaternion.Euler(0, currentRotationAngle, 0);
transform.position = target.position;
transform.position -= currentRotation * Vector3.forward * distance;
transform.position = new Vector3(transform.position.x, currentHeight, transform.position.z);
if (smoothRotation)
{
transform.LookAt(target);
}
else
{
transform.rotation = target.rotation;
}
}
}
```
将此脚本挂载到相机上,并将要跟随的目标物体指定为target,即可实现第三人称相机的控制。
阅读全文
相关推荐
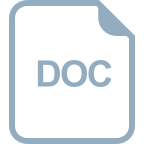
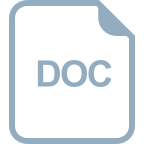
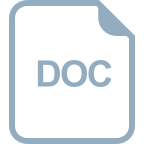
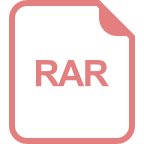
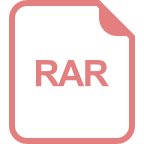
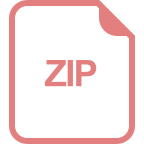
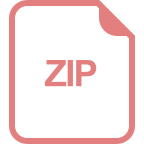





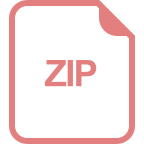



