import matplotlib.pyplot as plt acc=history.history["accuracy"] #fit方法返口的history类对象 #History类对象包含两个属性,分别为epoch(训练轮数)和history。History)所包含的内容是由compile参数的metrics确定的 loss=history.history["loss"]#训练集loss val_acc=history.history["val_accuracy"] val_loss=history.history["val_loss"]#测试集loss epochs=range(1,len(loss)+1) plt.figure() plt.plot(epochs,acc,"bo",label="Training acc") plt.plot(epochs,val_acc,"b",label="validation acc" ) plt.title("training and validation acc") plt.legend() plt.show()
时间: 2023-07-19 11:27:19 浏览: 176
这段代码是用来绘制训练集和测试集准确率随训练轮数变化的曲线。其中,`acc`是训练集的准确率,`val_acc`是测试集的准确率,`loss`是训练集的损失函数值,`val_loss`是测试集的损失函数值。`epochs`是训练的轮数。`plt.plot`函数用来绘制曲线,"bo"表示蓝色圆点,"b"表示蓝色实线。`plt.title`函数用来设置图标题,`plt.legend`函数用来设置图例,`plt.show`函数用来显示绘制好的图形。
相关问题
tokenizer = Tokenizer(num_words=max_words) tokenizer.fit_on_texts(data['text']) sequences = tokenizer.texts_to_sequences(data['text']) word_index = tokenizer.word_index print('Found %s unique tokens.' % len(word_index)) data = pad_sequences(sequences,maxlen=maxlen) labels = np.array(data[:,:1]) print('Shape of data tensor:',data.shape) print('Shape of label tensor',labels.shape) indices = np.arange(data.shape[0]) np.random.shuffle(indices) data = data[indices] labels = labels[indices] x_train = data[:traing_samples] y_train = data[:traing_samples] x_val = data[traing_samples:traing_samples+validation_samples] y_val = data[traing_samples:traing_samples+validation_samples] model = Sequential() model.add(Embedding(max_words,100,input_length=maxlen)) model.add(Flatten()) model.add(Dense(32,activation='relu')) model.add(Dense(10000,activation='sigmoid')) model.summary() model.compile(optimizer='rmsprop', loss='binary_crossentropy', metrics=['acc']) history = model.fit(x_train,y_train, epochs=1, batch_size=128, validation_data=[x_val,y_val]) import matplotlib.pyplot as plt acc = history.history['acc'] val_acc = history.history['val_acc'] loss = history.history['loss'] val_loss = history.history['val_loss'] epoachs = range(1,len(acc) + 1) plt.plot(epoachs,acc,'bo',label='Training acc') plt.plot(epoachs,val_acc,'b',label = 'Validation acc') plt.title('Training and validation accuracy') plt.legend() plt.figure() plt.plot(epoachs,loss,'bo',label='Training loss') plt.plot(epoachs,val_loss,'b',label = 'Validation loss') plt.title('Training and validation loss') plt.legend() plt.show() max_len = 10000 x_train = keras.preprocessing.sequence.pad_sequences(x_train, maxlen=max_len) x_test = data[10000:,0:] x_test = keras.preprocessing.sequence.pad_sequences(x_test, maxlen=max_len) # 将标签转换为独热编码 y_train = np.eye(2)[y_train] y_test = data[10000:,:1] y_test = np.eye(2)[y_test]
这段代码中存在几个问题:
1. `labels` 的赋值有误。应该是 `labels = np.array(data[:, 1:])`,因为标签是从第二列开始的。
2. `validation_data` 的格式有误。应该是 `validation_data=(x_val, y_val)`,而不是 `validation_data=[x_val, y_val]`。
3. 在使用 `Sequential` 模型时,需要指定输入的维度。这里应该是 `model.add(Dense(10000, activation='sigmoid', input_dim=maxlen))`,而不是 `model.add(Dense(10000, activation='sigmoid'))`。
4. 绘制图表时,应该使用 `val_acc` 和 `val_loss`,而不是 `test_acc` 和 `test_loss`。
5. 在将标签转换为独热编码时,应该使用 `y_train[:, 0]` 和 `y_test[:, 0]`,而不是 `y_train` 和 `y_test`。
以下是修改后的代码:
```python
tokenizer = Tokenizer(num_words=max_words)
tokenizer.fit_on_texts(data['text'])
sequences = tokenizer.texts_to_sequences(data['text'])
word_index = tokenizer.word_index
print('Found %s unique tokens.' % len(word_index))
data = pad_sequences(sequences, maxlen=maxlen)
labels = np.array(data[:, 1:])
print('Shape of data tensor:', data.shape)
print('Shape of label tensor', labels.shape)
indices = np.arange(data.shape[0])
np.random.shuffle(indices)
data = data[indices]
labels = labels[indices]
x_train = data[:training_samples]
y_train = labels[:training_samples]
x_val = data[training_samples:training_samples+validation_samples]
y_val = labels[training_samples:training_samples+validation_samples]
model = Sequential()
model.add(Embedding(max_words, 100, input_length=maxlen))
model.add(Flatten())
model.add(Dense(32, activation='relu'))
model.add(Dense(10000, activation='sigmoid', input_dim=maxlen))
model.summary()
model.compile(optimizer='rmsprop',
loss='binary_crossentropy',
metrics=['acc'])
history = model.fit(x_train, y_train,
epochs=1,
batch_size=128,
validation_data=(x_val, y_val))
import matplotlib.pyplot as plt
acc = history.history['acc']
val_acc = history.history['val_acc']
loss = history.history['loss']
val_loss = history.history['val_loss']
epochs = range(1, len(acc) + 1)
plt.plot(epochs, acc, 'bo', label='Training acc')
plt.plot(epochs, val_acc, 'b', label='Validation acc')
plt.title('Training and validation accuracy')
plt.legend()
plt.figure()
plt.plot(epochs, loss, 'bo', label='Training loss')
plt.plot(epochs, val_loss, 'b', label='Validation loss')
plt.title('Training and validation loss')
plt.legend()
plt.show()
max_len = 10000
x_train = keras.preprocessing.sequence.pad_sequences(x_train, maxlen=max_len)
x_test = data[10000:, 0:]
x_test = keras.preprocessing.sequence.pad_sequences(x_test, maxlen=max_len)
# 将标签转换为独热编码
y_train = np.eye(2)[y_train[:, 0]]
y_test = data[10000:, 1:]
y_test = np.eye(2)[y_test[:, 0]]
```
import numpy as np import pandas as pd from keras.models import Sequential from keras.layers import Dense from sklearn.model_selection import train_test_split # 读取数据集 data = pd.read_csv('img_16_10k.txt') X = data.iloc[:, :-1].values y = data.iloc[:, -1].values # 将标签进行one-hot编码 n_classes = len(np.unique(y)) y = np.eye(n_classes)[y] # 划分训练集和验证集 X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.1) # 搭建神经网络模型 model = Sequential() model.add(Dense(128, input_dim=X.shape[1], activation='relu')) model.add(Dense(64, activation='relu')) model.add(Dense(n_classes, activation='softmax')) # 编译模型 model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) # 训练模型 epochs = 50 batch_size = 32 history = model.fit(X_train, y_train, epochs=epochs, batch_size=batch_size, validation_data=(X_val, y_val)) # 绘制损失值变化图像和准确率变化图像 import matplotlib.pyplot as plt plt.plot(history.history['loss'], label='train_loss') plt.plot(history.history['val_loss'], label='val_loss') plt.legend() plt.show() plt.plot(history.history['accuracy'], label='train_acc') plt.plot(history.history['val_accuracy'], label='val_acc') plt.legend() plt.show()从绘制的可视化途中看得出预测的模型过拟合,帮我重新优化模型,处理过拟合问题
好的,根据您提供的信息,模型出现了过拟合问题,我们可以采取以下一些方法来进行优化:
1. 增加数据量:增加数据量是解决过拟合问题的最好方法之一,可以通过数据增强等方式增加数据量。
2. 简化模型:过拟合问题通常是由于模型过于复杂导致的,因此可以通过简化模型结构来减少过拟合的风险。比如可以减少神经网络的层数或者减少每层的神经元个数。
3. 正则化:正则化是一种常用的处理过拟合问题的方法,可以通过L1正则化或L2正则化等方式对网络权重进行惩罚,减少模型复杂度。在Keras中,可以通过在层中添加kernel_regularizer参数来进行正则化。
以下是修改过的代码:
```python
import numpy as np
import pandas as pd
from keras.models import Sequential
from keras.layers import Dense, Dropout
from sklearn.model_selection import train_test_split
from keras.regularizers import l2
# 读取数据集
data = pd.read_csv('img_16_10k.txt')
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
# 将标签进行one-hot编码
n_classes = len(np.unique(y))
y = np.eye(n_classes)[y]
# 划分训练集和验证集
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.1)
# 搭建神经网络模型
model = Sequential()
model.add(Dense(128, input_dim=X.shape[1], activation='relu', kernel_regularizer=l2(0.01)))
model.add(Dropout(0.5))
model.add(Dense(64, activation='relu', kernel_regularizer=l2(0.01)))
model.add(Dropout(0.5))
model.add(Dense(n_classes, activation='softmax'))
# 编译模型
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
epochs = 50
batch_size = 32
history = model.fit(X_train, y_train, epochs=epochs, batch_size=batch_size, validation_data=(X_val, y_val))
# 绘制损失值变化图像和准确率变化图像
import matplotlib.pyplot as plt
plt.plot(history.history['loss'], label='train_loss')
plt.plot(history.history['val_loss'], label='val_loss')
plt.legend()
plt.show()
plt.plot(history.history['accuracy'], label='train_acc')
plt.plot(history.history['val_accuracy'], label='val_acc')
plt.legend()
plt.show()
```
在上面的代码中,我们增加了Dropout层来减少过拟合的风险,在每个Dense层后添加了kernel_regularizer参数来进行L2正则化,减少模型复杂度。另外,我们可以适当增加Dropout的比例,减少神经元的数量,也可以达到减少过拟合的效果。
阅读全文
相关推荐
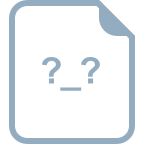
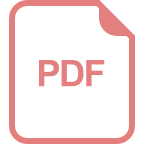
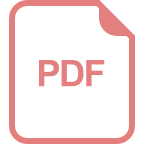













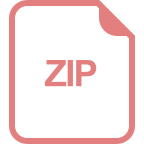