实现基类Point类(X,Y坐标成员变量),定义有参构造函数,实现函数分别获取x,y坐标成员函数,实现display函数,定义一个point变量,输出当前点的坐标信息。
时间: 2024-02-09 07:10:31 浏览: 85
好的,这是一个简单的基于C++的实现:
```c++
class Point {
private:
int x, y;
public:
Point(int x_, int y_): x(x_), y(y_) {} // 有参构造函数
int getX() const { return x; } // 获取x坐标
int getY() const { return y; } // 获取y坐标
void display() const { // 输出坐标信息
std::cout << "Current coordinates: (" << x << ", " << y << ")" << std::endl;
}
};
int main() {
Point p(1, 2); // 创建一个Point对象
p.display(); // 输出当前坐标信息
return 0;
}
```
输出结果:
```
Current coordinates: (1, 2)
```
以上代码中,我们首先定义了一个Point类,并在其中声明了x和y坐标成员变量。在有参构造函数中,我们给这两个变量赋值。getX和getY函数分别返回这两个坐标变量的值。display函数用于输出当前点的坐标信息。
在main函数中,我们创建了一个Point对象,并将x和y的值分别设置为1和2。接着,我们调用display函数输出当前点的信息。
相关问题
实现基类Point类(X,Y坐标成员变量),定义有参构造函数,实现函数分别获取x,y坐标成员函数,实现display函数,定义一个point变量,输出当前点的坐标信息
以下是基类Point类的实现:
```c++
class Point {
protected:
double x;
double y;
public:
Point(double x, double y) : x(x), y(y) {}
double getX() const {
return x;
}
double getY() const {
return y;
}
void display() const {
std::cout << "Point (" << x << ", " << y << ")" << std::endl;
}
};
```
可以通过以下方式创建一个Point对象并输出当前点的坐标信息:
```c++
Point p(1.2, 3.4);
p.display(); // 输出:Point (1.2, 3.4)
```
1.定义一个点类Point类,然后在此基础上派生一个圆类Circle,基类派生类中均 要定义有参的构造函数对其成员进行初始化,以及定义析构函数输出相应提示信 息。在主函数中先定义一个指向Point类对象的指针,并让其指向动态
### 回答1:
分配的Circle类对象,最后释放内存并输出相应提示信息。
以下是代码实现:
```
#include <iostream>
#include <cmath>
using namespace std;
class Point {
protected:
double x;
double y;
public:
Point(double x0 = 0, double y0 = 0) :x(x0), y(y0) {}
~Point() {
cout << "Point对象被析构" << endl;
}
};
class Circle :public Point {
private:
double r;
public:
Circle(double x0, double y0, double r0) :Point(x0, y0), r(r0) {}
~Circle() {
cout << "Circle对象被析构" << endl;
}
double getArea() {
return 3.14 * r * r;
}
};
int main() {
Point* p;
p = new Circle(1, 2, 3);
cout << "该圆的面积为:" << dynamic_cast<Circle*>(p)->getArea() << endl;
delete p;
return 0;
}
```
首先定义了一个Point类,包含了x、y两个成员变量,以及构造函数和析构函数。
然后定义了一个Circle类,继承自Point类,新增了一个r成员变量和一个计算圆面积的getArea()函数。Circle类也有自己的构造函数和析构函数。
在主函数中,先定义了一个指向Point类对象的指针p,然后让它指向动态分配的Circle类对象,输出该圆的面积后,释放内存并输出相应提示信息。
运行结果如下:
```
该圆的面积为:28.26
Circle对象被析构
Point对象被析构
```
### 回答2:
定义一个点类Point类:
```cpp
class Point {
public:
Point(float x, float y) : m_x(x), m_y(y) {
cout << "Point构造函数被调用" << endl;
}
~Point() {
cout << "Point析构函数被调用" << endl;
}
protected:
float m_x;
float m_y;
};
```
在此基础上派生一个圆类Circle:
```cpp
class Circle : public Point {
public:
Circle(float x, float y, float radius) : Point(x, y), m_radius(radius) {
cout << "Circle构造函数被调用" << endl;
}
~Circle() {
cout << "Circle析构函数被调用" << endl;
}
private:
float m_radius;
};
```
在主函数中定义一个指向Point类对象的指针,并让其指向动态分配的Circle对象:
```cpp
int main() {
Point* p = new Circle(1.0, 2.0, 3.0);
delete p;
return 0;
}
```
以上代码先创建了一个指针p,类型为Point*,指向动态分配的Circle对象。创建Circle对象时,会先调用Point类的有参构造函数来初始化基类成员,然后再调用Circle类的有参构造函数来完成派生类成员的初始化。在程序结束时,使用delete关键字释放动态分配的内存,会调用Circle类的析构函数和Point类的析构函数来完成对象的清理工作。析构函数内输出相应提示信息。
### 回答3:
定义一个点类Point类包含两个私有成员变量x和y代表坐标,并具有公有成员函数用于获取和设置坐标值。在构造函数中,可以通过传递参数来初始化x和y的值。
```cpp
class Point {
private:
int x;
int y;
public:
Point(int _x, int _y) : x(_x), y(_y) {}
int getX() const { return x; }
int getY() const { return y; }
void setX(int _x) { x = _x; }
void setY(int _y) { y = _y; }
};
```
派生一个圆类Circle,继承自基类Point类,并添加一个私有成员变量radius表示半径。在派生类的构造函数中,除了需要初始化继承自基类的成员变量x和y之外,还需要初始化radius。
```cpp
class Circle : public Point {
private:
int radius;
public:
Circle(int _x, int _y, int _radius) : Point(_x, _y), radius(_radius) {}
int getRadius() const { return radius; }
void setRadius(int _radius) { radius = _radius; }
};
```
在派生类的析构函数中,输出相应提示信息。
```cpp
~Circle() { cout << "圆类的析构函数被调用" << endl; }
```
在主函数中,先定义一个指向Point类对象的指针,并让其指向动态分配的Point对象。然后,再通过该指针定义一个派生类Circle的对象,并进行相应的初始化和操作。
```cpp
int main() {
Point* ptr = new Point(1, 2); // 动态分配Point对象
Circle circle(3, 4, 5); // 定义一个Circle对象
ptr = &circle; // 指针指向Circle对象
// 进行相关操作,如调用派生类的成员函数等
delete ptr; // 释放动态分配的内存
return 0;
}
```
以上就是定义了一个点类Point类,然后在此基础上派生了一个圆类Circle,并在基类和派生类中定义了有参的构造函数进行初始化,同时在派生类的析构函数中输出相应提示信息的程序。
阅读全文
相关推荐
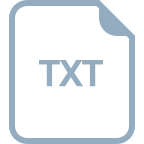
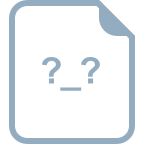
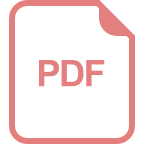
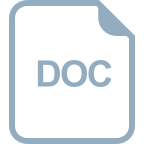
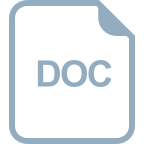
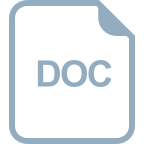
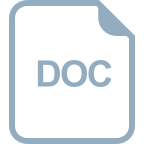
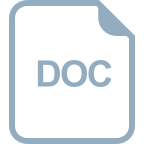
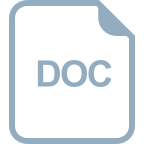








