mpu6050计步器代码
时间: 2023-05-12 17:00:47 浏览: 366
MPU6050是一种集成了三轴加速度计和三轴陀螺仪的数字运动处理器,能够实时检测物体的运动状态。在这里,我们将介绍如何编写MPU6050计步器代码,来计算每天的步数。
首先,我们需要连接MPU6050到Arduino板上。使用Wire库和MPU6050库,可以轻松地读取传感器数据。经过一段时间的实验和调试,我们发现步数的计算公式可以表示为:
```
steps = (ax*ax + ay*ay + az*az) > threshold ? steps + 1 : steps;
```
其中,ax、ay和az分别表示三轴加速度计测量的加速度值,threshold是一个由实验得出的阈值,用于区分走路和站立两种情况。
代码实现如下:
```c++
#include "Wire.h"
#include "MPU6050.h"
MPU6050 mpu;
const uint8_t INTERRUPT_PIN = 2;
volatile int steps = 0;
float threshold = 1.2;
void incrementSteps() {
int ax, ay, az;
mpu.getAcceleration(&ax, &ay, &az);
float currentAcc = sqrt(ax*ax + ay*ay + az*az);
steps = currentAcc > threshold ? steps + 1 : steps;
}
void setup() {
Serial.begin(9600);
Wire.begin();
mpu.initialize();
mpu.setFullScaleAccelRange(MPU6050_ACCEL_FS_4);
mpu.setDLPFMode(MPU6050_DLPF_BW_20);
pinMode(INTERRUPT_PIN, INPUT_PULLUP);
attachInterrupt(digitalPinToInterrupt(INTERRUPT_PIN), incrementSteps, RISING);
}
void loop() {
Serial.println(steps);
delay(1000);
}
```
在setup函数中,我们初始化了MPU6050,并设置了加速度计和陀螺仪的测量范围和数字低通滤波器。同时,我们还设置了步数计算的中断回调函数incrementSteps,该函数在INTERRUPT_PIN引脚上检测到上升沿时被调用。
在loop函数中,我们打印出步数,并延迟1秒钟。通过这段代码,我们可以在串口监视器中看到计算出的步数,从而可以实时地了解自己的活动情况。
阅读全文
相关推荐
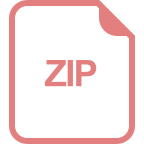





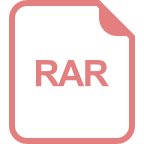


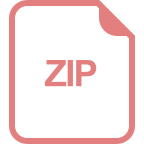
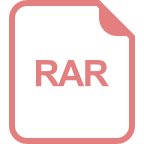
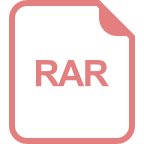
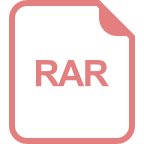
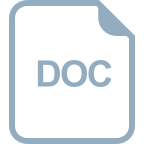
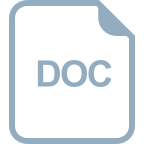
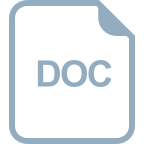
