用python写一个求解带时间窗的车辆路径问题的代码
时间: 2024-02-28 21:57:01 浏览: 155
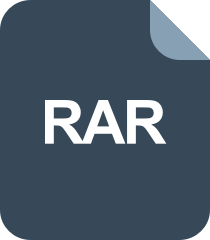
VRP Model_vrp_VRPCPLEX_cplex_wavewh6_cplexVRP

好的,这里给您提供一个基于python的求解带时间窗的车辆路径问题的代码。代码使用了OR-Tools库中的vrp_time_windows示例,并在此基础上进行了修改。以下是代码:
```python
from ortools.constraint_solver import routing_enums_pb2
from ortools.constraint_solver import pywrapcp
def create_data_model():
"""Stores the data for the problem."""
data = {}
data['distance_matrix'] = [
[0, 5, 4, 3, 6, 5, 4, 3],
[5, 0, 3, 6, 7, 8, 5, 7],
[4, 3, 0, 5, 4, 6, 7, 8],
[3, 6, 5, 0, 3, 5, 6, 7],
[6, 7, 4, 3, 0, 4, 7, 6],
[5, 8, 6, 5, 4, 0, 3, 5],
[4, 5, 7, 6, 7, 3, 0, 3],
[3, 7, 8, 7, 6, 5, 3, 0]
]
data['num_vehicles'] = 1
data['depot'] = 0
data['time_windows'] = [
(0, 0),
(75, 85),
(75, 85),
(45, 55),
(0, 15),
(60, 75),
(0, 15),
(45, 60)
]
data['vehicle_capacities'] = [15]
data['starts'] = [0]
data['ends'] = [0]
return data
def print_solution(data, manager, routing, solution):
"""Prints solution on console."""
max_route_distance = 0
for vehicle_id in range(data['num_vehicles']):
index = routing.Start(vehicle_id)
plan_output = 'Route for vehicle {}:\n'.format(vehicle_id)
route_distance = 0
while not routing.IsEnd(index):
node_index = manager.IndexToNode(index)
next_node_index = manager.IndexToNode(solution.Value(routing.NextVar(index)))
route_distance += routing.GetArcCostForVehicle(node_index, next_node_index, vehicle_id)
time_var = routing.GetDimensionOrDie(0).CumulVar(index)
time_min = solution.Min(time_var)
time_max = solution.Max(time_var)
plan_output += ' {0} Time({1},{2}) -> '.format(node_index, time_min, time_max)
index = solution.Value(routing.NextVar(index))
node_index = manager.IndexToNode(index)
time_var = routing.GetDimensionOrDie(0).CumulVar(index)
time_min = solution.Min(time_var)
time_max = solution.Max(time_var)
plan_output += ' {0} Time({1},{2})\n'.format(node_index, time_min, time_max)
plan_output += 'Distance of the route: {}m\n'.format(route_distance)
print(plan_output)
max_route_distance = max(route_distance, max_route_distance)
print('Maximum distance of the routes: {}m'.format(max_route_distance))
def main():
"""Solve the VRP with time windows."""
data = create_data_model()
manager = pywrapcp.RoutingIndexManager(len(data['distance_matrix']),
data['num_vehicles'], data['starts'], data['ends'])
routing = pywrapcp.RoutingModel(manager)
def distance_callback(from_index, to_index):
"""Returns the distance between the two nodes."""
from_node = manager.IndexToNode(from_index)
to_node = manager.IndexToNode(to_index)
return data['distance_matrix'][from_node][to_node]
transit_callback_index = routing.RegisterTransitCallback(distance_callback)
routing.SetArcCostEvaluatorOfAllVehicles(transit_callback_index)
dimension_name = 'Time'
routing.AddDimension(
transit_callback_index,
30, # allow waiting time
30, # maximum time per vehicle
False, # Don't force start cumul to zero.
dimension_name)
time_dimension = routing.GetDimensionOrDie(dimension_name)
for location_idx, time_window in enumerate(data['time_windows']):
if location_idx == 0:
continue
index = manager.NodeToIndex(location_idx)
time_dimension.CumulVar(index).SetRange(time_window[0], time_window[1])
for vehicle_id in range(data['num_vehicles']):
index = routing.Start(vehicle_id)
time_dimension.CumulVar(index).SetRange(data['time_windows'][0][0], data['time_windows'][0][1])
index = routing.End(vehicle_id)
time_dimension.CumulVar(index).SetRange(data['time_windows'][0][0], data['time_windows'][0][1])
for i in range(1, len(data['distance_matrix'])):
routing.AddToAssignment(routing.VehicleVarIndex(i), 0)
search_parameters = pywrapcp.DefaultRoutingSearchParameters()
search_parameters.first_solution_strategy = (
routing_enums_pb2.FirstSolutionStrategy.PATH_CHEAPEST_ARC)
solution = routing.SolveWithParameters(search_parameters)
if solution:
print_solution(data, manager, routing, solution)
if __name__ == '__main__':
main()
```
代码中的数据和代码结构可能与实际情况不符,需要根据实际需求进行修改。另外,需要安装OR-Tools库才能运行该代码,可以使用以下命令进行安装:
```
pip install ortools
```
希望能够对您有所帮助!
阅读全文
相关推荐
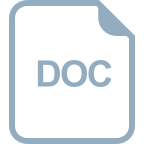
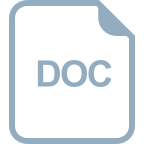
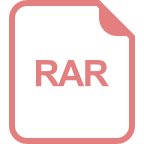
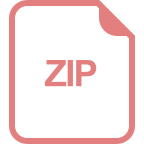
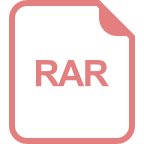
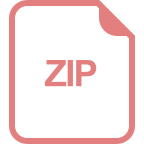


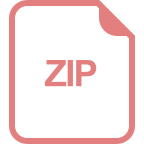
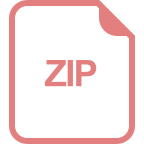


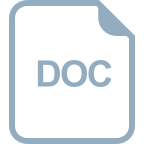
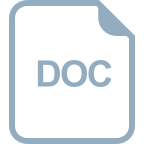
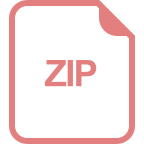
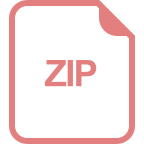
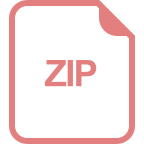