h = self.net(x) prediction = h.max(1)[1] accuracy = torch.eq(prediction, y_true).float().mean() cost = F.cross_entropy(h, y_true)
时间: 2024-04-20 08:25:12 浏览: 15
这部分代码是用来计算模型在原始样本上的预测结果、准确率和损失。
首先,通过self.net(x)将输入样本x输入到网络中,得到网络的输出结果h。
然后,通过h.max(1)[1]找到每个样本在输出结果中的最大值所对应的索引,即预测的类别。
接着,使用torch.eq(prediction, y_true)比较预测结果和真实标签是否相等,得到一个布尔值的张量。将这个布尔值张量转换为浮点型张量,并计算平均值,即为准确率。
最后,使用F.cross_entropy(h, y_true)计算交叉熵损失,其中h为网络的输出结果,y_true为真实标签。
这段代码的目的是评估模型在原始样本上的性能,准确率和损失是常用的评估指标。
如果还有其他问题,请随时提问。
相关问题
def FGSM(self, x, y_true, y_target=None, eps=0.03, alpha=2/255, iteration=1): self.set_mode('eval') x = Variable(cuda(x, self.cuda), requires_grad=True) y_true = Variable(cuda(y_true, self.cuda), requires_grad=False) if y_target is not None: targeted = True y_target = Variable(cuda(y_target, self.cuda), requires_grad=False) else: targeted = False h = self.net(x) prediction = h.max(1)[1] accuracy = torch.eq(prediction, y_true).float().mean() cost = F.cross_entropy(h, y_true) if iteration == 1: if targeted: x_adv, h_adv, h = self.attack.fgsm(x, y_target, True, eps) else: x_adv, h_adv, h = self.attack.fgsm(x, y_true, False, eps) else: if targeted: x_adv, h_adv, h = self.attack.i_fgsm(x, y_target, True, eps, alpha, iteration) else: x_adv, h_adv, h = self.attack.i_fgsm(x, y_true, False, eps, alpha, iteration) prediction_adv = h_adv.max(1)[1] accuracy_adv = torch.eq(prediction_adv, y_true).float().mean() cost_adv = F.cross_entropy(h_adv, y_true) # make indication of perturbed images that changed predictions of the classifier if targeted: changed = torch.eq(y_target, prediction_adv) else: changed = torch.eq(prediction, prediction_adv) changed = torch.eq(changed, 0) changed = changed.float().view(-1, 1, 1, 1).repeat(1, 3, 28, 28) changed[:, 0, :, :] = where(changed[:, 0, :, :] == 1, 252, 91) changed[:, 1, :, :] = where(changed[:, 1, :, :] == 1, 39, 252) changed[:, 2, :, :] = where(changed[:, 2, :, :] == 1, 25, 25) changed = self.scale(changed/255) changed[:, :, 3:-2, 3:-2] = x_adv.repeat(1, 3, 1, 1)[:, :, 3:-2, 3:-2] self.set_mode('train') return x_adv.data, changed.data,\ (accuracy.item(), cost.item(), accuracy_adv.item(), cost_adv.item())
这段代码是一个实现了FGSM(Fast Gradient Sign Method)攻击的函数。FGSM是一种用于生成对抗样本的方法,通过在输入样本上添加一小步扰动来欺骗分类器,使其产生错误的分类结果。
该函数的输入参数包括原始输入样本x、真实标签y_true、目标标签y_target(可选)、扰动范围eps、扰动步长alpha和迭代次数iteration。
函数首先将模型设置为评估模式,然后将输入样本转化为可求导变量,并计算原始样本在模型上的预测结果和准确率。然后计算原始样本在模型上的交叉熵损失。
接下来根据迭代次数选择使用FGSM攻击还是I-FGSM(Iterative FGSM)攻击。如果目标标签y_target存在,则使用目标攻击,否则使用非目标攻击。攻击过程中,对输入样本添加扰动,并计算扰动后的样本在模型上的预测结果和准确率。
最后,将产生的对抗样本、扰动图像、原始样本在模型上的准确率和损失作为输出返回。
值得注意的是,代码中还包含了一个用于生成扰动图像的部分。首先根据模型预测结果确定哪些样本的分类结果发生了变化,然后将这些样本的对应像素位置修改为特定的颜色值,以突出显示扰动部分。最后将扰动图像与对抗样本叠加,形成最终的扰动图像。
请问我还能为您解答其他问题吗?
def train(dataloader, model, loss_fn, optimizer): size = len(dataloader.dataset) model.train() LossList = [] for batch, (X, y) in enumerate(dataloader): X, y = X.to(device), y.to(device) y=y.long() # Compute prediction error pred = model(X) loss = loss_fn(pred, y) # Backpropagation optimizer.zero_grad() loss.backward() optimizer.step() if batch % 50 == 0: loss, current = loss.item(), (batch + 1) * len(X) print(f"loss: {loss:>7f} [{current:>5d}/{size:>5d}]") LossList.append(loss) return Losslist def test(dataloader, model, loss_fn): size = len(dataloader.dataset) num_batches = len(dataloader) model.eval() LossList = [] AccList = [] test_loss, correct = 0, 0 with torch.no_grad(): for X, y in dataloader: X, y = X.to(device), y.to(device) y=y.long() pred = model(X) test_loss += loss_fn(pred, y).item() correct += (pred.argmax(1) == y).type(torch.float).sum().item() test_loss /= num_batches correct /= size LossList.append(test_loss) AccList.append(correct) print(f"Test Error: \n Accuracy: {(100*correct):>0.1f}%, Avg loss: {test_loss:>8f} \n") return LossList,acclist报错显示lostlist未定义
在这段代码中,`losslist` 和 `acclist` 都没有被定义。你需要在函数内部定义这两个变量,或者在函数调用前将它们定义并且传入函数。例如,你可以在函数内部这样定义它们:
```
def train(dataloader, model, loss_fn, optimizer):
size = len(dataloader.dataset)
model.train()
losslist = [] # 在这里定义 losslist
for batch, (X, y) in enumerate(dataloader):
X, y = X.to(device), y.to(device)
y = y.long()
# Compute prediction error
pred = model(X)
loss = loss_fn(pred, y)
# Backpropagation
optimizer.zero_grad()
loss.backward()
optimizer.step()
if batch % 50 == 0:
loss, current = loss.item(), (batch + 1) * len(X)
print(f"loss: {loss:>7f} [{current:>5d}/{size:>5d}]")
losslist.append(loss) # 将 loss 添加到 losslist 中
return losslist
def test(dataloader, model, loss_fn):
size = len(dataloader.dataset)
num_batches = len(dataloader)
model.eval()
losslist = [] # 在这里定义 losslist
acclist = [] # 在这里定义 acclist
test_loss, correct = 0, 0
with torch.no_grad():
for X, y in dataloader:
X, y = X.to(device), y.to(device)
y = y.long()
pred = model(X)
test_loss += loss_fn(pred, y).item()
correct += (pred.argmax(1) == y).type(torch.float).sum().item()
test_loss /= num_batches
correct /= size
losslist.append(test_loss) # 将 test_loss 添加到 losslist 中
acclist.append(correct) # 将 correct 添加到 acclist 中
print(f"Test Error: \n Accuracy: {(100*correct):>0.1f}%, Avg loss: {test_loss:>8f} \n")
return losslist, acclist
```
相关推荐
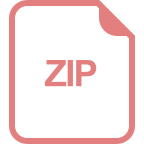
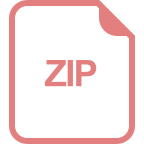












