Multi-Scale Training and Prediction Techniques in YOLOv8
发布时间: 2024-09-15 07:24:03 阅读量: 45 订阅数: 48 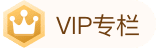
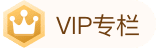
# Multi-scale Training and Prediction Techniques in YOLOv8
## 2.1 Data Augmentation Techniques
### 2.1.1 Image Transformations
Image transformation is a common data augmentation technique that generates new training samples by applying various transformations to the original images, ***mon image transformations include:
- **Flipping:** Flipping the image horizontally or vertically to enhance the model's robustness to objects in different orientations.
- **Rotation:** Rotating the image at certain angles to simulate the different postures that objects may assume in the real world.
- **Scaling:** Changing the size of the image to mimic the appearance of objects at varying distances.
- **Cropping:** Randomly cropping out regions of different sizes and shapes from the original image to increase the model's adaptability to occlusion and local variations.
### 2.1.2 Mosaic Data Augmentation
Mosaic data augmentation is a special data augmentation technique that divides an image into multiple grids and then randomly replaces the pixels in each grid with those from other grids. This technique can effectively disrupt the local correlation within images, enhancing the model's robustness to noise and interference.
## 2. YOLOv8 Training Techniques
### 2.1 Data Augmentation Techniques
Data augmentation techniques are effective means to improve a model's generalization and robustness. YOLOv8 provides a variety of data augmentation techniques, including image transformations and mosaic data augmentation.
#### 2.1.1 Image Transformations
Image transformations include random cropping, rotation, flipping, and scaling. These operations can alter the dimensions, angles, and orientation of images, thus increasing the model's adaptability to different images.
```python
import cv2
import numpy as np
# Random Crop
def random_crop(image, target_size):
h, w, c = image.shape
x = np.random.randint(0, w - target_size[0])
y = np.random.randint(0, h - target_size[1])
return image[y:y+target_size[1], x:x+target_size[0], :]
# Random Rotate
def random_rotate(image, angle_range):
angle = np.random.uniform(angle_range[0], angle_range[1])
return cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE, angle)
# Random Flip
def random_flip(image):
return cv2.flip(image, 1)
# Random Scale
def random_scale(image, scale_range):
scale = np.random.uniform(scale_range[0], scale_range[1])
return cv2.resize(image, (int(image.shape[1] * scale), int(image.shape[0] * scale)))
```
#### 2.1.2 Mosaic Data Augmentation
Mosaic data augmentation is a technique that divides images into small blocks and randomly mixes these blocks. It helps the model learn the local features and global relationships of images.
```python
import cv2
import numpy as np
# Mosaic Data Augmentation
def mosaic_augment(images, target_size):
h, w, c = images[0].shape
num_grids = np.random.randint(1, 5)
grid_size = target_size // num_grids
mosaic_image = np.zeros((target_size, target_size, c), dtype=np.uint8)
for i in range(num_grids):
for j in range(num_grids):
grid_x = np.random.randint(0, w - grid_size)
grid_y = np.random.randint(0, h - grid_size)
mosaic_image[i*grid_size:(i+1)*grid_size, j*grid_size:(j+1)*grid_size, :] = images[np.random.randint(0, len(images))][grid_y:grid_y+grid_size, grid_x:grid_x+grid_size, :]
return mosaic_image
```
### 2.2 Optimizers and Loss Functions
Optimizers and loss functions are key factors in training a model. YOLOv8 provides various options for optimizers and loss functions.
#### 2.2.1 Common Optimizers
Common optimizers include SGD, Momentum, Adam, and RMSprop. These optimizers minimize the loss function by updating the model's weights.
| Optimizer | Pros | Cons |
|---|---|---|
| SGD | Simple and efficient | Slow convergence |
| Momentum | Accelerates convergence | May cause oscillations |
| Adam | Adaptive learning rate | May lead to overfitting |
| RMSprop | Good stability | May lead to slow convergence |
#### 2.2.2 Selection of Loss Functions
Loss functions measure the difference between the model's predictions and the true labels. YOLOv8 supports various loss functions, including cross-entropy loss, mean squared error loss, and IoU loss.
| Loss Function | Pros | Cons |
|---|---|---|
| Cross-entropy loss | Computationally simple | Sensitive to outliers |
| Mean squared error loss | Robust | May lead to overfitting |
| IoU loss | Directly measures the overlap of predi
0
0
相关推荐
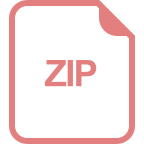
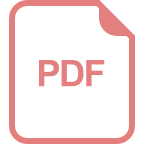
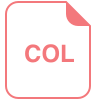
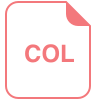
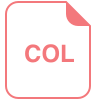
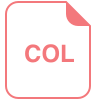
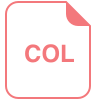
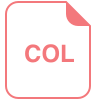
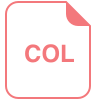