Handling Class Imbalance in YOLOv8 Object Detection Tasks
发布时间: 2024-09-15 07:29:23 阅读量: 104 订阅数: 24 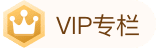
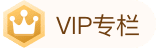
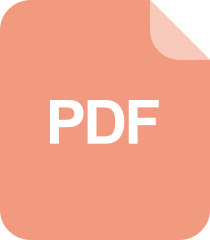
Combating the class imbalance problem in sparse representation learning
# 1. Overview of the Class Imbalance Problem
The class imbalance problem is prevalent in machine learning, referring to an uneven distribution of sample quantities across different classes within a dataset. Some classes (minority classes) have significantly fewer samples compared to others (majority classes). This imbalance can lead to overfitting of the model to the majority class samples during training, resulting in lower prediction accuracy for the minority class samples.
# 2. Methods for Handling Class Imbalance
Class imbalance is common in real-world datasets, where the sample quantities of some classes (minorities) are far less than others (majorities). This can cause machine learning models to favor the majority classes, leading to poor prediction outcomes for the minority samples. To address this issue, various methods have been proposed, including oversampling, undersampling, and cost-sensitive learning.
### 2.1 Oversampling Methods
Oversampling methods increase the quantity of minority class samples by duplicating or synthesizing new instances, thereby balancing the dataset.
#### 2.1.1 Random Oversampling
Random oversampling is the simplest form of oversampling, where minority class samples are randomly duplicated to increase their number. While easy to implement, it may introduce noise and overfitting.
#### 2.1.2 SMOTE Algorithm
Synthetic Minority Over-sampling Technique (SMOTE) is a more complex but effective oversampling algorithm. It synthesizes new samples by interpolating between existing minority class samples. This method generates synthetic samples that are similar to the original data distribution, reducing noise and overfitting.
### 2.2 Undersampling Methods
Undersampling methods decrease the number of majority class samples to balance the dataset.
#### 2.2.1 Random Undersampling
Random undersampling is the simplest form of undersampling, where majority class samples are randomly deleted. It is straightforward but may result in the loss of valuable information.
#### 2.2.2 Cluster Centroid Undersampling
Cluster centroid undersampling is a more complex yet more effective undersampling algorithm. It clusters the majority class samples and then deletes the centroid samples of each cluster. This method preserves diversity within the majority class samples, reducing information loss.
### 2.3 Cost-sensitive Learning
Cost-sensitive learning addresses class imbalance by adjusting the model's loss function or regularization terms.
#### 2.3.1 Cost-sensitive Loss Function
The cost-sensitive loss function adjusts the model's loss function by assigning higher weights to minority class samples. This forces the model to focus more on minority classes, thereby improving prediction outcomes.
#### 2.3.2 Cost-sensitive Regularization
Cost-sensitive regularization adjusts the model's regularization terms by assigning higher weights to minority class samples. This helps prevent overfitting to the majority class samples, thus improving the prediction outcomes for minority classes.
**Code Example:**
```python
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
# Load data
data = pd.read_csv('data.csv')
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(data.drop('label', axis=1), data['label'], test_size=0.2)
# Create cost-sensitive loss function
class_weights = {0: 1, 1: 10}
loss_function = 'log_loss'
# Create cost-sensitive model
model = LogisticRegression(class_weight=class_weights, loss=loss_function)
# Train model
model.fit(X_train, y_train)
# Evaluate model
score = model.score(X_test, y_test)
print('Model Score:', score)
```
**Logical Analysis:**
This code example demonstrates the implementation of cost-sensitive learning. It adjusts the model's loss function by assigning higher weights to minority class samples, thus improving their prediction outcomes. The `class_weight` parameter specifies the weights for different classes, while the `loss_function` parameter specifies the loss function. During model training, the model is optimized based on the cost-sensitive loss function, focusing more on minority class samples.
**Parameter Explanation:**
* `class_weight`: Weights for different classes, in dictionary form with class labels as keys and weights as values.
* `loss_function`: Loss function, in string form, with supported loss functions including `log_loss`, `hinge`, `squared_loss`, etc.
# 3. Handling Class Imbalance in YOLOv8
### 3.1 YOLOv8 Network Architecture
YOLOv8 is a one-stage object detection algorithm. Its network architecture mainly consists of the following parts:
***Backbone Network:** Utilizes EfficientNet as the backbone network to extract image features.
***Neck Network:** Employs PANet as the neck network to fuse feature maps from different levels.
***Detection Head:** Adopts the YOLOv5 detection head to predict bounding boxes and class probabilities.
### 3.2 Class Imbalance Handling Strategies
YOLOv8 employs various strategies to handle class imbalance, including:
#### 3.2.1 Data Augmentation
Data augmentation is a common method for dealing with class imbalance. The data augmentation techniques used in YOLOv8 include:
***Random Cropping:** Randomly crops images to different sizes and shapes.
***Random Flipping:** Horizontally or vertically flips images.
***Color Jittering:** Changes the brightness, contrast, saturation, and hue of images.
***Mosaic Data Augmentation:** Combines multiple images into a single mo
0
0
相关推荐
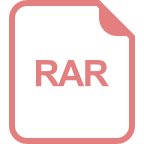
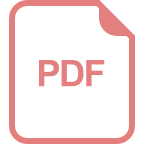
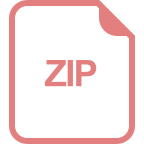
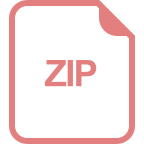
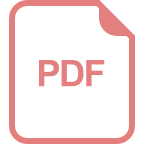
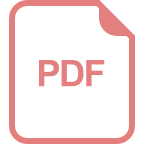
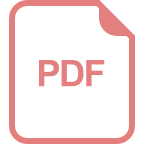
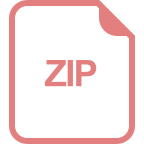