需求分析以下内容:实现一个Student类,其中含有S个学期的成绩数据。 每个学期的课程数目可不相同,各学期的课程数目存储在course_no[S]数组中;float* score[S] 通过指针数组实现二维数组结构,存储S个学期的所有成绩数据; 带参构造函数Student(int _id, string _name, int c_no[ ])初始化id,name,course_no[S],并为成绩数组申请必要的存储空间;拷贝构造函数中新对象id增加1,其他信息相同,但注意使用深复制。 input_scores(float * _score[ ])从参数中得到各科成绩并设置isFail的值。update()函数按照提示语句更新学生的相关信息。可以修改对应学生的学号,姓名和成绩。修改成绩时需要先输入学期数(学期数从1开始),再输入该学期中待修改的成绩序号(从1开始),如果输入的数据不在范围内,则提示重新输入,直到范围正确后再输入新成绩。修改完一项成绩后可继续修改下一项成绩,直到不需要修改为止。具体情况所对应的输入提示语句请见下述说明,请注意:提示语句中的学生id应根据实际的id输出。 主函数main输入学号,姓名,各学期课程数目,各学期成绩,创建学生对象s1,并根据s1创建另一学生s2,使用update( )更新s2相关信息,最后输出两个学生的所有信息。 请注意:main中输入各学期成绩时,如为第1学期的3门课程,则其提示语句为"Please enter the 3 scores for the 1-th semester",提示语句中的两个数字要根据实际情况变化。
时间: 2024-03-07 22:51:51 浏览: 23
需求分析:
1. 创建一个名为Student的类,包含S个学期的成绩数据。
2. 使用数组course_no[S]存储每个学期的课程数目。
3. 使用指针数组float* score[S],实现二维数组结构,存储S个学期的所有成绩数据。
4. 实现带参构造函数Student(int _id, string _name, int c_no[]),初始化id,name,course_no[S],并为成绩数组申请必要的存储空间。
5. 实现拷贝构造函数,其中新对象id增加1,其他信息相同,但注意使用深复制。
6. 实现input_score(float * _score[])函数,通过参数得到各科成绩并设置isFail的值。
7. 实现update()函数,按照提示语句更新学生的相关信息。可以修改对应学生的学号,姓名和成绩。修改成绩时需要先输入学期数和待修改的成绩序号,如果输入的数据不在范围内,则提示重新输入,直到范围正确后再输入新成绩。
8. 实现主函数main,输入学号,姓名,各学期课程数目,各学期成绩,创建学生对象s1,并根据s1创建另一学生s2,使用update()更新s2相关信息,最后输出两个学生的所有信息。
具体实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
int id;
string name;
int* course_no;
float** score;
public:
Student(int _id, string _name, int c_no[]) {
id = _id;
name = _name;
course_no = new int[S];
for (int i = 0; i < S; i++) {
course_no[i] = c_no[i];
score[i] = new float[c_no[i]];
}
}
Student(const Student& s) {
id = s.id + 1;
name = s.name;
course_no = new int[S];
score = new float*[S];
for (int i = 0; i < S; i++) {
course_no[i] = s.course_no[i];
score[i] = new float[s.course_no[i]];
for (int j = 0; j < s.course_no[i]; j++) {
score[i][j] = s.score[i][j];
}
}
}
void input_score(float* _score[]) {
for (int i = 0; i < S; i++) {
for (int j = 0; j < course_no[i]; j++) {
score[i][j] = _score[i][j];
}
}
}
void update() {
cout << "Please enter the new student information: " << endl;
cout << "Student ID: ";
cin >> id;
cout << "Student name: ";
cin >> name;
for (int i = 0; i < S; i++) {
cout << "Semester " << i+1 << ", enter the scores to update:";
if (i == 0) {
cout << course_no[i] << " courses" << endl;
}
else {
cout << course_no[i] - course_no[i - 1] << " courses" << endl;
}
for (int j = 0; j < course_no[i]; j++) {
cout << "Course " << j+1 << ": " << score[i][j] << endl;
}
int semester;
cout << "Please enter the semester number you want to update: ";
cin >> semester;
if (semester < 1 || semester > S) {
cout << "Invalid semester number, please enter again." << endl;
i--;
continue;
}
int index;
cout << "Please enter the score index you want to update: ";
cin >> index;
if (index < 1 || index > course_no[semester - 1]) {
cout << "Invalid score index, please enter again." << endl;
i--;
continue;
}
cout << "Please enter the new score: ";
cin >> score[semester - 1][index - 1];
}
}
void display() {
cout << "Student ID: " << id << endl;
cout << "Student name: " << name << endl;
for (int i = 0; i < S; i++) {
cout << "Semester " << i+1 << ", " << course_no[i] << " courses" << endl;
for (int j = 0; j < course_no[i]; j++) {
cout << "Course " << j+1 << ": " << score[i][j] << endl;
}
}
}
~Student() {
delete[] course_no;
for (int i = 0; i < S; i++) {
delete[] score[i];
}
delete[] score;
}
};
const int S = 3;
int main() {
int id, c_no[S];
string name;
float* scores[S];
for (int i = 0; i < S; i++) {
cout << "Please enter the number of courses for the " << i+1 << "-th semester: ";
cin >> c_no[i];
scores[i] = new float[c_no[i]];
cout << "Please enter the " << c_no[i] << " scores for the " << i+1 << "-th semester: ";
for (int j = 0; j < c_no[i]; j++) {
cin >> scores[i][j];
}
}
cout << "Please enter the student ID: ";
cin >> id;
cout << "Please enter the student name: ";
cin >> name;
Student s1(id, name, c_no);
s1.input_score(scores);
Student s2(s1);
s2.update();
cout << "Information for student 1: " << endl;
s1.display();
cout << "Information for student 2: " << endl;
s2.display();
return 0;
}
```
相关推荐
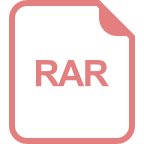
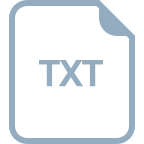
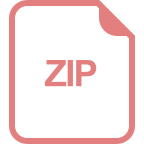














