用java高级图形界面实现利用map存放单词及汉字解释,map存储在文件中,程序运行时将文件中信息读入到 map中。支持: (1)单词查阅,输入英文,输出中文。 (2)可添加新单词;添加结果要写入到文件中。添加单词如果已存在,则修改信息。 (3)可删除单词。删除后的单词表写入文件中。
时间: 2024-01-22 18:20:02 浏览: 62
好的,这是一个比较完整的项目,需要编写一定的代码。我可以给你提供一个基本的思路,你可以根据这个思路进行代码的编写。
1. 定义一个HashMap用于存放单词及其对应的中文解释,key为单词,value为中文解释。
2. 将HashMap存储到文件中,可以使用Java的ObjectOutputStream来实现。
3. 程序启动时,读取文件中的HashMap,可以使用Java的ObjectInputStream来实现。
4. 实现单词查阅功能,可以通过输入英文单词,从HashMap中获取中文解释并输出。
5. 实现添加新单词功能,可以输入英文单词和对应的中文解释,将其存储到HashMap中,并将HashMap存储到文件中。
6. 实现删除单词功能,可以输入英文单词,从HashMap中删除该单词,并将HashMap存储到文件中。
下面是一个简单的代码示例,你可以根据这个示例进行修改和完善:
```java
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
import java.io.*;
public class WordDictionary {
private static final String FILE_NAME = "dictionary.dat";
private Map<String, String> dictionary;
public WordDictionary() {
loadDictionaryFromFile();
}
public void loadDictionaryFromFile() {
try {
FileInputStream fis = new FileInputStream(FILE_NAME);
ObjectInputStream ois = new ObjectInputStream(fis);
dictionary = (Map<String, String>) ois.readObject();
ois.close();
fis.close();
} catch (Exception e) {
e.printStackTrace();
}
if (dictionary == null) {
dictionary = new HashMap<>();
}
}
public void saveDictionaryToFile() {
try {
FileOutputStream fos = new FileOutputStream(FILE_NAME);
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(dictionary);
oos.close();
fos.close();
} catch (Exception e) {
e.printStackTrace();
}
}
public void addWord(String word, String meaning) {
dictionary.put(word, meaning);
saveDictionaryToFile();
}
public void removeWord(String word) {
dictionary.remove(word);
saveDictionaryToFile();
}
public void lookupWord(String word) {
String meaning = dictionary.get(word);
if (meaning == null) {
System.out.println("Word not found!");
} else {
System.out.println(meaning);
}
}
public static void main(String[] args) {
WordDictionary dict = new WordDictionary();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("1. Lookup word");
System.out.println("2. Add word");
System.out.println("3. Remove word");
System.out.println("4. Quit");
System.out.print("Enter your choice: ");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("Enter the word: ");
String word = scanner.next();
dict.lookupWord(word);
break;
case 2:
System.out.print("Enter the word: ");
String newWord = scanner.next();
System.out.print("Enter the meaning: ");
String meaning = scanner.next();
dict.addWord(newWord, meaning);
System.out.println("Word added successfully!");
break;
case 3:
System.out.print("Enter the word: ");
String removeWord = scanner.next();
dict.removeWord(removeWord);
System.out.println("Word removed successfully!");
break;
case 4:
System.exit(0);
break;
default:
System.out.println("Invalid choice!");
}
}
}
}
```
这个示例代码中实现了基本的单词查阅、添加新单词和删除单词的功能,并将HashMap存储到了文件中。你可以根据需求进行修改和完善。
阅读全文
相关推荐
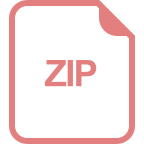
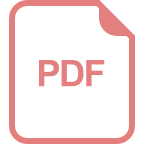
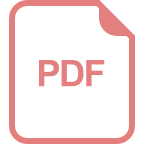















