利用回溯法来设计并实现装载问题,数据自拟,给出python代码
时间: 2023-09-10 17:08:59 浏览: 84
装载问题是指有若干个集装箱需要被装载到一艘载重量为c的货船上,每个集装箱有自己的重量和编号,问如何选择装载的集装箱,使得货船装载的集装箱重量最大。
回溯法是一种试探性的搜索方法,能够找出所有满足条件的解。在装载问题中,我们可以通过回溯法来枚举所有可能的解,然后选出最优解。具体算法如下:
1. 定义一个数组selected,用于记录已经选择的集装箱编号;
2. 定义一个变量current_weight,用于记录当前已经装载的集装箱总重量;
3. 定义一个变量max_weight,用于记录找到的最大重量;
4. 定义一个函数load,用于递归搜索所有可能的解:
(1)如果已经选择的集装箱编号数等于总数,说明已经找到一组解,比较当前重量和最大重量,更新max_weight;
(2)否则,枚举所有未选择的集装箱,如果加上该箱子的重量不超过载重量c,就把该箱子加入selected数组,当前重量加上该箱子的重量,继续递归搜索;否则,不选择该箱子,继续枚举下一个未选择的箱子;
(3)回溯时,从selected数组中删除最后一个元素,当前重量减去该元素的重量。
根据以上算法,我们可以实现如下的Python代码:
```python
def load(boxes, c, selected, current_weight, max_weight):
if len(selected) == len(boxes):
if current_weight > max_weight:
max_weight = current_weight
return max_weight
for i in range(len(boxes)):
if i not in selected and current_weight + boxes[i] <= c:
selected.append(i)
current_weight += boxes[i]
max_weight = load(boxes, c, selected, current_weight, max_weight)
current_weight -= boxes[i]
selected.pop()
return max_weight
if __name__ == '__main__':
boxes = [50, 40, 30, 20, 10]
c = 100
selected = []
current_weight = 0
max_weight = 0
print(load(boxes, c, selected, current_weight, max_weight))
```
上述代码中,我们选取了5个集装箱,重量分别为50、40、30、20和10,而货船的载重量为100。运行程序后,输出结果为90,表示最大装载量为90。
阅读全文
相关推荐

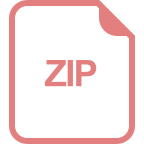











