python多进程如何获取返回值
时间: 2023-10-10 22:06:34 浏览: 395

multi-process-test.tar.gz
在 Python 多进程中,可以使用 `multiprocessing` 模块的 `Pool` 对象中的 `apply_async()` 方法来实现异步执行函数,然后使用 `get()` 方法获取函数的返回值。
具体操作如下:
1. 导入 `multiprocessing` 模块:
```python
import multiprocessing
```
2. 创建一个 `Pool` 对象:
```python
pool = multiprocessing.Pool(processes=4) # 创建 4 个进程
```
3. 使用 `apply_async()` 方法异步执行函数:
```python
result = pool.apply_async(func, args=(arg1, arg2, ...))
```
其中,`func` 是需要执行的函数名,`args` 是传递给函数的参数。
4. 使用 `get()` 方法获取函数的返回值:
```python
value = result.get()
```
`get()` 方法会阻塞程序,直到函数执行完毕并返回结果。
完整的示例代码如下:
```python
import multiprocessing
def func(x):
return x * x
if __name__ == '__main__':
pool = multiprocessing.Pool(processes=4)
result = pool.apply_async(func, args=(10,))
value = result.get()
print(value)
```
输出结果为:
```
100
```
注意:在多进程编程中,由于子进程与主进程是相互独立的,因此不能直接使用全局变量来传递数据,需要使用 `Queue` 或 `Pipe` 等进程通信方式来传递数据。
阅读全文
相关推荐
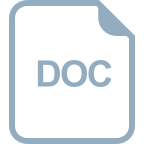
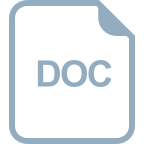

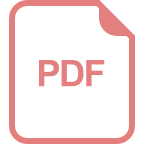






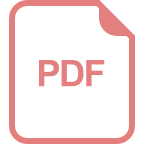
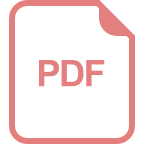





