2)数据结构设计: 1、创建一个Int类型的二维数组int maze[n1][n2],用来存放迷宫各位置(0表示通道;1表示墙壁,数组矩阵增加外圈墙壁;2表示该位置被选作路径); 2) 创建一个栈linkstack top[n1*n2],用来存储“当前路径”,即“在搜索过程中某一时刻所在图中某个方块位置”,其中linkstack定义为: typedef struct node { int x; //当前路径在迷宫中的位置 int y; //当前路径在迷宫中的位置 int c; //当前路径的前进方向 一共有8个方向 }linkstack;
时间: 2024-02-17 18:02:53 浏览: 24
好的,根据你的要求,我来设计一个数据结构,用来解决迷宫问题。
首先,我们需要创建一个二维数组 maze[n1][n2],用来存放迷宫各位置的状态,其中:
- 0 表示通道
- 1 表示墙壁
- 2 表示该位置被选作路径
为了方便处理边界问题,我们可以将数组矩阵增加外圈墙壁。这样,迷宫的大小就变成了 (n1+2) x (n2+2)。
然后,我们需要创建一个栈 linkstack,用来存储“当前路径”,即“在搜索过程中某一时刻所在图中某个方块位置”。栈中的元素类型为 linkstack,其中 x 和 y 表示当前路径在迷宫中的位置,c 表示当前路径的前进方向,一共有 8 个方向。
linkstack 的定义如下:
```c++
typedef struct node {
int x; // 当前路径在迷宫中的位置
int y; // 当前路径在迷宫中的位置
int c; // 当前路径的前进方向,一共有8个方向
} linkstack;
```
下面是完整的数据结构设计代码:
```c++
const int N = 1000;
int maze[N+2][N+2]; // 迷宫地图
typedef struct node {
int x; // 当前路径在迷宫中的位置
int y; // 当前路径在迷宫中的位置
int c; // 当前路径的前进方向,一共有8个方向
} linkstack;
linkstack top[N*N]; // 栈
int top_index = -1; // 栈顶指针
void push(int x, int y, int c) { // 入栈
top_index++;
top[top_index].x = x;
top[top_index].y = y;
top[top_index].c = c;
maze[x][y] = 2; // 标记为路径
}
void pop() { // 出栈
maze[top[top_index].x][top[top_index].y] = 0; // 标记为通道
top_index--;
}
bool is_empty() { // 判断栈是否为空
return top_index == -1;
}
int get_top_x() { // 获取栈顶元素的 x 坐标
return top[top_index].x;
}
int get_top_y() { // 获取栈顶元素的 y 坐标
return top[top_index].y;
}
int get_top_c() { // 获取栈顶元素的前进方向
return top[top_index].c;
}
```
这个数据结构中,我们还定义了一些常用的函数,如 push、pop、is_empty、get_top_x、get_top_y、get_top_c 等,用来操作栈。
相关推荐
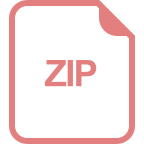
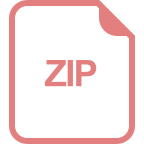














