定义一个二维数组: int maze[5][5] = { 0, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 1, 0, }; 它表示一个迷宫,其中的1表示墙壁,0表示可以走的路,只能横着走或竖着走,不能斜着走,要求编程序找出从左上角到右下角的最短路线。
时间: 2023-05-30 19:01:36 浏览: 128
以下是一个可能的解法,使用BFS算法:
#include <iostream>
#include <queue>
using namespace std;
const int N = 5;
int maze[N][N] = {
{0, 1, 0, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 0, 0, 1, 0}
};
// 定义一个结构体表示一个点的坐标
struct Point {
int x, y;
};
// 定义一个函数判断一个点是否合法(在迷宫内且没有墙壁)
bool isValid(int x, int y) {
return x >= 0 && x < N && y >= 0 && y < N && maze[x][y] == 0;
}
int bfs(Point start, Point end) {
// 定义一个队列,用于存储待访问的点
queue<Point> q;
// 定义一个二维数组,用于记录每个点是否已经访问过
bool visited[N][N] = {false};
// 定义一个二维数组,用于记录从起点到每个点的距离
int distance[N][N] = {0};
// 将起点加入队列
q.push(start);
visited[start.x][start.y] = true;
distance[start.x][start.y] = 0;
// 定义一个数组表示可以走的四个方向
int dx[4] = {-1, 0, 1, 0};
int dy[4] = {0, 1, 0, -1};
// 开始遍历队列
while (!q.empty()) {
// 取出队首元素
Point p = q.front();
q.pop();
// 如果当前点是终点,直接返回距离
if (p.x == end.x && p.y == end.y) {
return distance[p.x][p.y];
}
// 枚举四个方向
for (int i = 0; i < 4; i++) {
int nx = p.x + dx[i];
int ny = p.y + dy[i];
// 如果新的点合法且没有访问过,加入队列
if (isValid(nx, ny) && !visited[nx][ny]) {
q.push({nx, ny});
visited[nx][ny] = true;
distance[nx][ny] = distance[p.x][p.y] + 1;
}
}
}
// 如果没有找到终点,返回-1
return -1;
}
int main() {
Point start = {0, 0};
Point end = {N-1, N-1};
int shortestPath = bfs(start, end);
cout << "Shortest path: " << shortestPath << endl;
return 0;
}
相关推荐
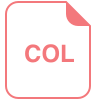
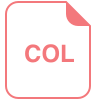













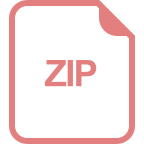