import networkx as nx import random # 读入edge文件,构建图结构 graph = {} with open("./edge/football.edge", "r") as f: for line in f: u, v = line.strip().split() if u not in graph: graph[u] = [] if v not in graph: graph[v] = [] graph[u].append(v) graph[v].append(u) # 随机选择一个节点作为感染源 infected = random.choice(list(graph.keys())) print("Infected node:", infected) # 初始化每个节点的感染状态 status = {} for node in graph: status[node] = "S" status[infected] = "I" # SI模型参数 p = 0.5 # 感染过程 while True: changed = False for node in graph: if status[node] == "I": for neighbor in graph[node]: if status[neighbor] == "S" and random.random() < p: status[neighbor] = "I" changed = True if not changed: break # 输出每个节点的感染状态和邻居 for node in graph: print(node, status[node], graph[node])续写一个溯源代码
时间: 2023-07-15 21:13:47 浏览: 80
以下是基于SI模型的溯源代码,可以追踪感染源及其传播路径:
```python
# 溯源参数
q = 0.8 # 溯源成功率
source = None # 感染源
path = {} # 每个节点的传播路径
# 溯源过程
for node in graph:
if status[node] == "I":
success = random.random() < q
if success: # 溯源成功
source = node
path[node] = [node]
break
if source is not None: # 有感染源
for node in graph:
if status[node] == "I" and node != source:
current = node
current_path = [current]
while current != source:
neighbors = [neighbor for neighbor in graph[current] if status[neighbor] == "I" and neighbor != source and neighbor not in current_path]
if len(neighbors) == 0: # 无法继续追溯
break
else:
current = random.choice(neighbors)
current_path.append(current)
path[node] = current_path
# 输出感染源及其传播路径
print("Infection source:", source)
for node in path:
print(node, path[node])
else: # 无感染源
print("No infection source found.")
```
该代码首先定义了溯源参数,包括溯源成功率和感染源变量。然后,代码遍历图中所有感染节点,以指定的溯源成功率进行随机判断,如果溯源成功,则将该节点标记为感染源,并记录该节点的传播路径。接着,代码遍历所有感染节点,对于每个节点,从当前节点开始沿着感染路径一直追溯到感染源,如果无法继续追溯,则停止追溯。最后,代码输出感染源及其传播路径。如果没有感染源,则输出相应提示。
阅读全文
相关推荐
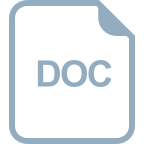
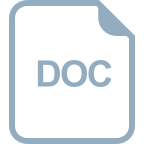
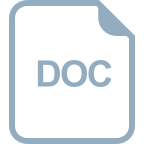















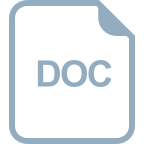