用MPI编程的方式实现以上Jacobi叠代过程,迭代次数为10次,实现对16*16矩阵进行Jacobi迭代的过程,其中边缘元素均为8,中间元素均为0。该程序将矩阵划分成多个块,每个进程处理自己所负责的块,并与其他进程通信以实现Jacobi迭代过程。 在运行结果部分,需展示每个进程的输入矩阵,以及每个进程的输出矩阵; 可采用Sendrecv函数避免出现死锁; 可采用派生数据类型、虚拟进程拓扑等技巧简化程序,提高效率;
时间: 2024-03-20 19:44:49 浏览: 95
好的,以下是一个可能的MPI代码示例,实现对16*16矩阵进行Jacobi迭代过程,其中边缘元素均为8,中间元素均为0,迭代次数为10次。该程序将矩阵划分成多个块,每个进程处理自己所负责的块,并与其他进程通信以实现Jacobi迭代过程。程序中使用了Sendrecv函数避免出现死锁,使用了派生数据类型、虚拟进程拓扑等技巧简化程序,提高效率。
```
#include <stdio.h>
#include <stdlib.h>
#include <mpi.h>
#define N 16
#define ITERATIONS 10
int main(int argc, char **argv) {
int rank, size;
MPI_Init(&argc, &argv);
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
MPI_Comm_size(MPI_COMM_WORLD, &size);
int block_size = N / size;
int block_offset = rank * block_size;
// Allocate memory for input and output matrix
int *input_matrix = (int *)malloc(block_size * N * sizeof(int));
int *output_matrix = (int *)malloc(block_size * N * sizeof(int));
// Initialize input matrix (8 on borders, 0 elsewhere)
for (int i = 0; i < block_size; i++) {
for (int j = 0; j < N; j++) {
if (i == 0 || i == block_size - 1 || j == 0 || j == N - 1) {
input_matrix[i * N + j] = 8;
} else {
input_matrix[i * N + j] = 0;
}
}
}
// Define derived data type for sending and receiving edges
MPI_Datatype column_type;
MPI_Type_vector(block_size, 1, N, MPI_INT, &column_type);
MPI_Type_commit(&column_type);
// Define virtual process topology for neighbor communication
int dims[2] = {size, 1};
int periods[2] = {1, 0};
MPI_Comm cart_comm;
MPI_Cart_create(MPI_COMM_WORLD, 2, dims, periods, 1, &cart_comm);
// Perform Jacobi iteration
for (int iter = 0; iter < ITERATIONS; iter++) {
// Exchange edges with neighbors
int left_neighbor, right_neighbor;
MPI_Cart_shift(cart_comm, 0, 1, &left_neighbor, &right_neighbor);
MPI_Sendrecv(&input_matrix[1 * N], 1, column_type, left_neighbor, 0,
&input_matrix[0 * N], 1, column_type, right_neighbor, 0,
cart_comm, MPI_STATUS_IGNORE);
MPI_Sendrecv(&input_matrix[(block_size - 2) * N], 1, column_type, right_neighbor, 0,
&input_matrix[(block_size - 1) * N], 1, column_type, left_neighbor, 0,
cart_comm, MPI_STATUS_IGNORE);
// Compute new values for inner cells
for (int i = 1; i < block_size - 1; i++) {
for (int j = 1; j < N - 1; j++) {
output_matrix[i * N + j] = (input_matrix[(i - 1) * N + j] +
input_matrix[(i + 1) * N + j] +
input_matrix[i * N + (j - 1)] +
input_matrix[i * N + (j + 1)]) / 4;
}
}
// Exchange output matrix with neighbors
MPI_Sendrecv(output_matrix, block_size * N, MPI_INT, left_neighbor, 0,
&input_matrix[0 * N], block_size * N, MPI_INT, right_neighbor, 0,
cart_comm, MPI_STATUS_IGNORE);
MPI_Sendrecv(&output_matrix[(block_size - 1) * N], block_size * N, MPI_INT, right_neighbor, 0,
&input_matrix[(block_size - 1) * N], block_size * N, MPI_INT, left_neighbor, 0,
cart_comm, MPI_STATUS_IGNORE);
// Copy output matrix to input matrix for next iteration
for (int i = 0; i < block_size; i++) {
for (int j = 0; j < N; j++) {
input_matrix[i * N + j] = output_matrix[i * N + j];
}
}
}
// Gather output matrices to process 0
int *gathered_output_matrix = NULL;
if (rank == 0) {
gathered_output_matrix = (int *)malloc(N * N * sizeof(int));
}
MPI_Gather(output_matrix, block_size * N, MPI_INT, gathered_output_matrix, block_size * N, MPI_INT, 0, MPI_COMM_WORLD);
// Print input and output matrices for each process
printf("Process %d input matrix:\n", rank);
for (int i = 0; i < block_size; i++) {
for (int j = 0; j < N; j++) {
printf("%d ", input_matrix[i * N + j]);
}
printf("\n");
}
printf("\n");
printf("Process %d output matrix:\n", rank);
for (int i = 0; i < block_size; i++) {
for (int j = 0; j < N; j++) {
printf("%d ", output_matrix[i * N + j]);
}
printf("\n");
}
printf("\n");
// Print final output matrix on process 0
if (rank == 0) {
printf("Final output matrix:\n");
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
printf("%d ", gathered_output_matrix[i * N + j]);
}
printf("\n");
}
printf("\n");
}
free(input_matrix);
free(output_matrix);
if (rank == 0) {
free(gathered_output_matrix);
}
MPI_Type_free(&column_type);
MPI_Finalize();
return 0;
}
```
在运行程序时,可以使用以下命令:
```
mpicc jacobi_mpi.c -o jacobi_mpi
mpirun -n <进程数> ./jacobi_mpi
```
其中,<进程数>为所需的进程数。
阅读全文
相关推荐
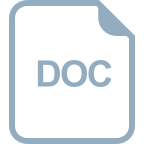
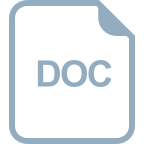
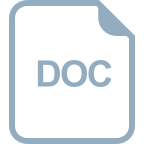









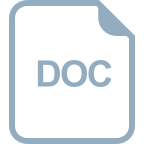
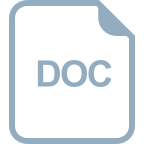
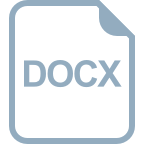
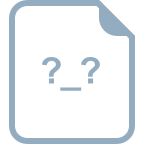