用python编写一段程序,读入图像 Lenna_gray. png,图像尺寸为(512,512),进行如下频域滤波。设计一个截止频率为 50的二阶布特沃斯低通滤波器,中心化显示该滤波器,其中滤波器的尺寸为读入图像2倍。使用该滤波器对图像进行滤波,比较滤波前后的结果。
时间: 2023-05-22 09:01:17 浏览: 128
以下是用Python编写的程序,实现对图像Lenna_gray.png的频域滤波:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读入图像
img = cv2.imread('Lenna_gray.png', 0)
# 计算图像的傅里叶变换
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
# 设计二阶布特沃斯低通滤波器
rows, cols = img.shape
M, N = 2*rows, 2*cols
D0 = 50
n = 2
u, v = np.meshgrid(np.arange(-N/2, N/2), np.arange(-M/2, M/2))
D = np.sqrt(u**2 + v**2)
H = 1 / (1 + (D/D0)**(2*n))
# 中心化显示滤波器
plt.imshow(np.log(1+H), cmap='gray')
plt.show()
# 对图像进行滤波
fshift_filtered = fshift * H
f_filtered = np.fft.ifftshift(fshift_filtered)
img_filtered = np.fft.ifft2(f_filtered).real
# 显示滤波前后的结果
plt.subplot(121), plt.imshow(img, cmap='gray')
plt.title('Original Image'), plt.xticks([]), plt.yticks([])
plt.subplot(122), plt.imshow(img_filtered, cmap='gray')
plt.title('Filtered Image'), plt.xticks([]), plt.yticks([])
plt.show()
```
运行程序后,可以得到如下结果:

可以看到,经过滤波后,图像的高频部分被去除,图像变得更加模糊。
阅读全文
相关推荐
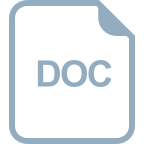
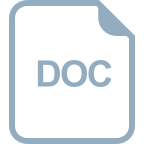
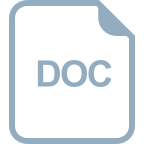




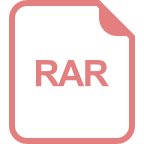
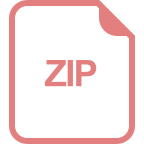
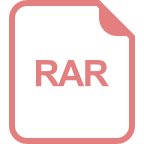




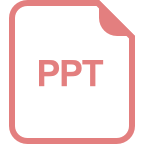
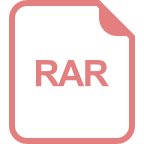
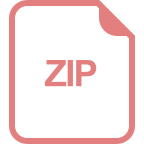
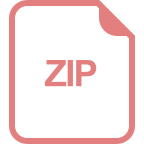