python代码去除中文停用词
时间: 2023-09-09 16:10:18 浏览: 81
以下是一个示例代码,可以使用中文停用词表去除文本中的中文停用词:
```python
import jieba
import codecs
# 加载中文停用词表
stopwords = codecs.open('stopwords.txt', 'r', encoding='utf8').readlines()
stopwords = [w.strip() for w in stopwords]
# 分词并去除中文停用词
def cut_without_stopwords(text):
words = jieba.cut(text)
return [word for word in words if word not in stopwords]
# 示例
text = '今天天气真好,我想出去玩。'
words = cut_without_stopwords(text)
print(words)
```
其中,`stopwords.txt` 是一个包含中文停用词的文本文件,每个停用词占一行。在代码中,首先将停用词表加载到一个列表中。然后,定义一个函数 `cut_without_stopwords`,该函数接受一个文本字符串作为输入,将文本分词并去除其中的停用词,最终返回一个不包含停用词的词列表。最后,我们可以将一个示例文本字符串传递给该函数,并打印输出结果。
需要注意的是,以上代码使用了 `jieba` 库进行中文分词,需要先安装该库。
相关问题
python去中文文本停用词代码
好的,以下是一个基本的 Python 去除中文停用词的代码示例:
```
import jieba
import codecs
# 加载中文停用词表
def load_stopwords(file_path):
stopwords = []
with codecs.open(file_path, 'r', 'utf-8') as f:
for line in f.readlines():
stopwords.append(line.strip())
return set(stopwords)
# 去除停用词
def remove_stopwords(text, stopwords):
words = jieba.lcut(text)
filtered_words = [word for word in words if word not in stopwords]
return "".join(filtered_words)
# 加载中文停用词表
stopwords = load_stopwords("stopwords.txt")
# 测试
text = "今天是个好日子,我们一起去郊游吧。"
filtered_text = remove_stopwords(text, stopwords)
print(filtered_text)
```
在上述代码中,我们首先使用 `codecs` 模块读取了一个中文停用词表文件,并将其中的停用词存储在一个集合中。接着,我们定义了一个 `remove_stopwords` 函数,它接受一个字符串和一个停用词集合作为输入,然后将其中的停用词去除,并返回处理后的文本。在函数中,我们首先使用 `jieba` 对输入文本进行分词,然后逐个判断每个单词是否为停用词,如果不是则将其加入到 `filtered_words` 列表中。最后,我们使用 `join` 方法将 `filtered_words` 中的单词拼接成一个字符串,并返回该字符串。
请注意,上述代码仅仅是一个基本的示例,实际应用中可能需要根据具体情况对其进行更加细致的调整。此外,还需要注意的是,在实际应用中,可能需要使用更加全面、准确的中文停用词表。
python去除停用词代码
可以使用以下代码来去除停用词:
```
import nltk
nltk.download('stopwords')
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
words = ['hello', 'world', 'this', 'is', 'a', 'test', 'sentence']
filtered_words = [word for word in words if word.lower() not in stop_words]
print(filtered_words) # Output: ['hello', 'world', 'test', 'sentence']
```
此处的停用词列表为英语停用词列表,如果需要用其他语言的停用词列表可以在nltk.corpus中找到相应的语言停用词列表。
相关推荐
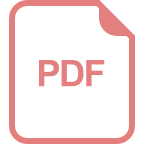
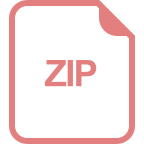
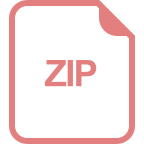












