c++某公司需要存储雇员的编号、姓名、性别、所在部门,级别,并进行工资的计算。其中的雇员分为经理、技术人员。月薪计算方法如下:经理拿固定目薪;技术人员按小时领取月薪。功能需求: (1)设计一程序能够对公司人员进行管理,应用到继承、抽象类、虚函数、虚基类、多态和文件的输入/输出等内容。 (2)添加功能:根据用户选择的人员类别,添加经理或者技术人员。要求员工的编号要唯一,如果添加了重复编号的记录时,则提示数据添加重复,并取消添加。 (3)查询功能:可根据编号、姓名等信息对已添加的记录进行查询,如果未找到,给出相应的提示信息,如果找到,则显示相应的记录信息;可显示当前系统中所有记录,每条记录占据一行。
时间: 2023-07-14 11:14:16 浏览: 65
这是一个比较典型的面向对象设计问题,需要考虑继承、类的关系以及多态的应用。以下是一个简单的设计方案:
1. 建立一个基类Employee,包含编号、姓名、性别、所在部门和级别等基本信息,以及计算月薪的纯虚函数calculateMonthlySalary()。
2. 派生出Manager类和Technician类,分别代表经理和技术人员。Manager类只需要重写calculateMonthlySalary()函数,计算固定目薪即可;Technician类需要增加一个小时工资的成员变量并重写calculateMonthlySalary()函数。
3. 建立一个EmployeeList类,用于管理所有员工的信息,包含一个vector<Employee*>类型的成员变量employees,用于存储所有员工的指针。
4. 在EmployeeList类中添加添加员工和查询员工的功能,可以通过员工类别、编号、姓名等信息进行查询。添加员工时需要检查是否有重复编号的记录。
5. 使用文件的输入/输出,将员工信息保存到文件中,以便下次程序运行时可以读取。
以下是一个简单的代码实现,仅供参考:
```
#include <iostream>
#include <vector>
#include <fstream>
using namespace std;
class Employee {
public:
Employee(int id, string name, char gender, string department, string level)
: m_id(id), m_name(name), m_gender(gender), m_department(department), m_level(level) {}
virtual ~Employee() {}
virtual double calculateMonthlySalary() = 0;
int getId() const { return m_id; }
string getName() const { return m_name; }
char getGender() const { return m_gender; }
string getDepartment() const { return m_department; }
string getLevel() const { return m_level; }
protected:
int m_id;
string m_name;
char m_gender;
string m_department;
string m_level;
};
class Manager : public Employee {
public:
Manager(int id, string name, char gender, string department, string level, double salary)
: Employee(id, name, gender, department, level), m_salary(salary) {}
double calculateMonthlySalary() { return m_salary; }
private:
double m_salary;
};
class Technician : public Employee {
public:
Technician(int id, string name, char gender, string department, string level, double hourlyWage)
: Employee(id, name, gender, department, level), m_hourlyWage(hourlyWage) {}
double calculateMonthlySalary() { return m_hourlyWage * 160; }
private:
double m_hourlyWage;
};
class EmployeeList {
public:
EmployeeList() {}
~EmployeeList() {
for (auto it = employees.begin(); it != employees.end(); ++it) {
delete *it;
}
}
void addEmployee(Employee* employee) {
for (auto it = employees.begin(); it != employees.end(); ++it) {
if ((*it)->getId() == employee->getId()) {
cout << "Error: Duplicate employee ID.\n";
return;
}
}
employees.push_back(employee);
}
Employee* findEmployeeById(int id) {
for (auto it = employees.begin(); it != employees.end(); ++it) {
if ((*it)->getId() == id) {
return *it;
}
}
return nullptr;
}
void printAllEmployees() {
for (auto it = employees.begin(); it != employees.end(); ++it) {
cout << (*it)->getId() << "\t" << (*it)->getName() << "\t"
<< (*it)->getGender() << "\t" << (*it)->getDepartment() << "\t"
<< (*it)->getLevel() << "\t" << (*it)->calculateMonthlySalary() << endl;
}
}
void saveToFile(string filename) {
ofstream ofs(filename);
if (!ofs.is_open()) {
cout << "Error: Cannot open file " << filename << " for writing.\n";
return;
}
for (auto it = employees.begin(); it != employees.end(); ++it) {
ofs << (*it)->getId() << "\t" << (*it)->getName() << "\t"
<< (*it)->getGender() << "\t" << (*it)->getDepartment() << "\t"
<< (*it)->getLevel() << "\t" << (*it)->calculateMonthlySalary() << endl;
}
ofs.close();
}
void loadFromFile(string filename) {
ifstream ifs(filename);
if (!ifs.is_open()) {
cout << "Error: Cannot open file " << filename << " for reading.\n";
return;
}
int id;
string name, department, level;
char gender;
double salaryOrHourlyWage;
while (ifs >> id >> name >> gender >> department >> level >> salaryOrHourlyWage) {
if (level == "Manager") {
employees.push_back(new Manager(id, name, gender, department, level, salaryOrHourlyWage));
} else if (level == "Technician") {
employees.push_back(new Technician(id, name, gender, department, level, salaryOrHourlyWage));
}
}
ifs.close();
}
private:
vector<Employee*> employees;
};
int main() {
EmployeeList list;
list.loadFromFile("employees.txt");
while (true) {
cout << "1. Add employee\n";
cout << "2. Find employee\n";
cout << "3. Print all employees\n";
cout << "4. Save to file\n";
cout << "5. Exit\n";
int choice;
cout << "Enter your choice: ";
cin >> choice;
if (choice == 1) {
cout << "1. Manager\n";
cout << "2. Technician\n";
int type;
cout << "Enter employee type: ";
cin >> type;
if (type == 1) {
int id;
string name, department, level;
char gender;
double salary;
cout << "Enter employee ID: ";
cin >> id;
cout << "Enter employee name: ";
cin >> name;
cout << "Enter employee gender: ";
cin >> gender;
cout << "Enter employee department: ";
cin >> department;
cout << "Enter employee level: ";
cin >> level;
cout << "Enter employee salary: ";
cin >> salary;
list.addEmployee(new Manager(id, name, gender, department, level, salary));
} else if (type == 2) {
int id;
string name, department, level;
char gender;
double hourlyWage;
cout << "Enter employee ID: ";
cin >> id;
cout << "Enter employee name: ";
cin >> name;
cout << "Enter employee gender: ";
cin >> gender;
cout << "Enter employee department: ";
cin >> department;
cout << "Enter employee level: ";
cin >> level;
cout << "Enter employee hourly wage: ";
cin >> hourlyWage;
list.addEmployee(new Technician(id, name, gender, department, level, hourlyWage));
}
} else if (choice == 2) {
int id;
cout << "Enter employee ID to find: ";
cin >> id;
Employee* employee = list.findEmployeeById(id);
if (employee == nullptr) {
cout << "Employee not found.\n";
} else {
cout << employee->getId() << "\t" << employee->getName() << "\t"
<< employee->getGender() << "\t" << employee->getDepartment() << "\t"
<< employee->getLevel() << "\t" << employee->calculateMonthlySalary() << endl;
}
} else if (choice == 3) {
list.printAllEmployees();
} else if (choice == 4) {
list.saveToFile("employees.txt");
} else if (choice == 5) {
break;
}
}
return 0;
}
```
在以上代码中,我们使用了vector来存储所有员工的指针,并且在EmployeeList类的析构函数中遍历所有指针并释放内存。在添加员工和查询员工时,我们遍历vector来查找对应的员工指针。在保存和读取文件时,我们使用了fstream库提供的文件输入/输出功能,将员工信息保存到文件中或从文件中读取。
相关推荐
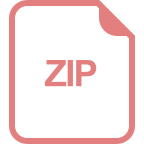
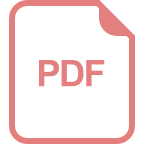
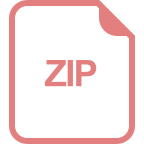















