使用c语言,用链表储存三元组
时间: 2023-08-10 17:23:29 浏览: 106
好的,以下是使用 C 语言实现用链表储存三元组的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int val1; // 第一个元素
int val2; // 第二个元素
int val3; // 第三个元素
struct Node* next; // 指向下一个节点的指针
} Node;
typedef struct LinkedList{
Node* head; // 链表头指针
} LinkedList;
// 初始化链表
void initLinkedList(LinkedList* list) {
list->head = (Node*)malloc(sizeof(Node));
list->head->next = NULL;
}
// 在链表末尾插入一个三元组
void insert(LinkedList* list, int v1, int v2, int v3) {
Node* cur = list->head;
while (cur->next != NULL) {
cur = cur->next;
}
Node* node = (Node*)malloc(sizeof(Node));
node->val1 = v1;
node->val2 = v2;
node->val3 = v3;
node->next = NULL;
cur->next = node;
}
// 删除链表中指定的三元组
void removeNode(LinkedList* list, int v1, int v2, int v3) {
Node* cur = list->head;
while (cur->next != NULL) {
if (cur->next->val1 == v1 && cur->next->val2 == v2 && cur->next->val3 == v3) {
Node* temp = cur->next;
cur->next = cur->next->next;
free(temp);
return;
}
cur = cur->next;
}
}
// 查找链表中指定的三元组
int find(LinkedList* list, int v1, int v2, int v3) {
Node* cur = list->head->next;
while (cur != NULL) {
if (cur->val1 == v1 && cur->val2 == v2 && cur->val3 == v3) {
return 1;
}
cur = cur->next;
}
return 0;
}
// 释放链表空间
void freeLinkedList(LinkedList* list) {
Node* cur = list->head;
while (cur != NULL) {
Node* temp = cur;
cur = cur->next;
free(temp);
}
}
// 测试代码
int main() {
LinkedList list;
initLinkedList(&list);
insert(&list, 1, 2, 3);
insert(&list, 4, 5, 6);
insert(&list, 7, 8, 9);
removeNode(&list, 4, 5, 6);
printf("%d\n", find(&list, 7, 8, 9)); // 输出 1
printf("%d\n", find(&list, 4, 5, 6)); // 输出 0
freeLinkedList(&list);
return 0;
}
```
在这个示例中,我们定义了链表节点结构体 `Node` 和链表结构体 `LinkedList`,并实现了初始化链表、插入、删除、查找和释放链表空间等操作。
阅读全文
相关推荐
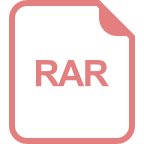

















